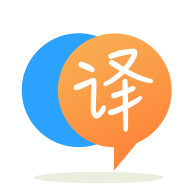
[英]How to make for loop wait until Async call was successful before to continue
[英]How to add a delay with JQuery? (make it wait 5s before continue)
具體而言:
發生的情況是,第一次運行正常...但是在下次運行時,它不會等待這5秒鍾!
代碼如下:
//==================== create variables: ====================
var x = 0;
//==================== create functions: ====================
function wait5s() {
setTimeout(function() {
alert("alert # " + x + "\nNext alert will pop up in 5 seconds now!");
}, 5000);
x++;
}
function repeat5times(){
while (x<5) {
wait5s()
}
}
//==================== run this: ====================
alert("Click Ok and Wait 10 seconds for next alert!");
setTimeout(function() {
repeat5times();
}, 5000);
該代碼不是我的原始代碼,但問題是相同的:-在警報彈出之前,x會增加到5! 然后他們一個接一個地彈出! 我試圖把x++;
在setTimeout內部的wait5s()內部...但是瀏覽器停止響應! :(
我希望每個警報之間延遲5秒!
所以,我在做什么錯? :\\ 提前致謝 ;)
setTimeout
是一個異步函數,不會阻止您的wait5s方法調用。 因此,您一次又一次將超時設置為5次,這會將警報調用功能放入要在5秒后立即調用的超時隊列中。
您需要的是在調用第一個超時功能后調用下一個wait5s。
function wait5s(callback) {
setTimeout(function () {
alert("alert # " + x + "\nNext alert will pop up in 5 seconds now!");
x++;
if(callback){
callback();
}
}, 5000);
}
function repeat5times() {
var callback = function () {
if (x < 5) {
wait5s(callback);
}
};
wait5s(callback);
}
您需要在setTimeout
函數中包含x,以便每5秒觸發一次x ++。
您正在調用wait5s()
; 在x為5之前連續調用它。它連續啟動5個setTimeOut函數,這就是為什么5秒鍾后它們一個接一個地彈出的原因:
while (x<5) { wait5s() }
就像有:
setTimeout(function() { alert("go after 5sec");}, 5000);
setTimeout(function() { alert("go after 5sec");}, 5000);
setTimeout(function() { alert("go after 5sec");}, 5000);
setTimeout(function() { alert("go after 5sec");}, 5000);
setTimeout(function() { alert("go after 5sec");}, 5000);
這就是為什么它無法按您預期的方式工作的原因。
一種解決方案是讓wait5s();
在setTimeout
函數內部的if語句中。
問題是此代碼:
function repeat5times(){
while (x<5) {
wait5s()
}
}
只需同時設置5個setTimeouts即可。 如果您希望它每5秒運行5次,則需要對它進行不同的編碼。 您可以在第一個觸發時使用setInterval()
或設置一個新的setTimeout()
,也可以設置5個超時,每個超時具有不同的時間間隔。
這是一個在上一個方法完成時為下一次迭代調用setTimeout()
方法:
function repeat5times() {
var cntr = 0;
function run() {
if (cntr < 5) {
setTimeout(function() {
++cntr;
console.log("alert # " + x + "\nNext alert will pop up in 5 seconds now!");
run();
}, 5000);
}
}
run();
}
這是另一個使用setInterval
的方法:
function repeat5times() {
var cntr = 0;
var timer = setInterval(function() {
console.log("alert # " + x + "\nNext alert will pop up in 5 seconds now!");
++cntr;
if (cntr >= 5) {
clearInterval(timer);
}
}, 5000);
}
這是使用變量setTimeouts的方法:
function waitNs(sec) {
setTimeout(function() {
console.log("alert # " + x + "\nNext alert will pop up in 5 seconds now!");
}, sec * 1000);
}
function repeat5times() {
for (var i = 1; i <= 5; i++) {
waitNs(i * 5);
}
}
您正在同時調用5個警報,請嘗試以下代碼
var x = 0;
var t=0;
//==================== create functions: ====================
function wait5s() {
t+=5000;
setTimeout(function() {
alert("alert # " + x + "\nNext alert will pop up in 5 seconds now!");
},t);
x++;
}
function repeat5times(){
while (x<5) { wait5s() }
}
您可以嘗試用更簡單的方式嘗試此操作,如果第一次調用應該在5秒后執行,那么對於第二個函數調用,它應該在10秒之后。 同樣,三分之一應該在15秒后
//==================== create variables: ====================
var x = 1;
//==================== create functions: ====================
function wait5s(myVariable) {
setTimeout(function() {
alert("alert # " + x + "\nNext alert will pop up in 5 seconds now!");
}, myVariable * 5000);
}
function repeat5times(){
while (x<=5) {
wait5s(x)
x++;
}
}
看來您實際上是在尋找setInterval
而不是setTimeout
。
function myAlert() {
alert('Next alert in 5 secs');
}
var si = setInterval(myAlert, 5000)
使用clearInterval
停止計時器。
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.