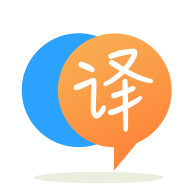
[英]How to take a specific line from a text file and read it into an array? (Java)
[英]How to read a specific line from a text file in Java?
我正在使用文件中的信息創建測驗游戲。 文本文件將包含所有問題,答案和正確答案。 文本文件的結構如下:
Question
answer 1
answer 2
answer 3
answer 4
The right answer repeated
使用從文件中讀取的信息,我正在創建多個測驗。 我將所有問題保存在一個數組中,將答案保存在另一個數組中,並將正確答案(重復)保存在另一個數組中。 有什么辦法可以使用掃描儀轉到文件中的特定行?
到目前為止,這就是我所擁有的。 我能夠從文件中獲取所有問題,但是我不知道如何讀取答案(我在這里省略了用於獲取文件名和計數行數的方法的代碼,因為我知道這些工作):
import java.io.File;
import java.io.FileNotFoundException;
import java.util.Scanner;
//for the file, it is assumed that it is structured as follows: question /n, answer 1 /n, answer 2 /n,
//answer 3 /n, answer 4 /n, correct answer repeated
public class CoreCode {
public static void main(String[] args) {
// TODO Auto-generated method stub
String fileName = getFile();
// gets the file and deals with exceptions
Scanner fileIn = null;
try {
fileIn = new Scanner(new File(fileName));
} catch (FileNotFoundException e) {
System.out.println("Error. File not found.");
// e.printStackTrace();
}
//counts number of lines in the program
int numberOfLines = countLines(fileIn);
//Every sixth line is a question
int numberOfQuestions = numberOfLines / 6;
fileIn.close();
fileIn = null;
try {
fileIn = new Scanner(new File(fileName));
} catch (FileNotFoundException e) {
System.out.println("Error. File not found.");
}
//create an array of the questions from the file
String[] questions = getQuestions(fileIn, numberOfQuestions);
fileIn.close();
for (int i = 0; i < questions.length; i++){
System.out.println(questions[i]);
}
}
// method to get file name
public static String getFile() {
}
//method to count total lines in file
public static int countLines(Scanner fileIn) {
}
// method to read questions
public static String[] getQuestions(Scanner fileIn, int numberOfQuestions) {
String[] questions = new String[numberOfQuestions];
int count = 0;
//method 2, every sixth line is a question
while (fileIn.hasNext()){
String line = fileIn.nextLine();
if (count == 0 || count % 6 == 0){
//count is number of total lines, so the index is count divided by 6 (every sixth lines is
//a question
int index = count / 6;
questions[index] = line;
}
count++;
}
return questions;
}
}
LineIterator it = IOUtils.lineIterator(
new BufferedReader(new FileReader("file.txt")));
for (int lineNumber = 0; it.hasNext(); lineNumber++) {
String line = (String) it.next();
if (lineNumber == expectedLineNumber) {
return line;
}
}
由於有緩沖區,這將稍微更有效。
看看Scanner.skip(..)
我將按照另一個答案的建議,使用BufferedReader
讀取文件,並將信息保存在包含以下內容的對象中:
class Question {
String question;
String[] answers;
String correctAnswer;
}
然后,在讀取文件之后,您將在內存中存儲所有問題和答案(存儲在ArrayList
)。 這樣,您將只讀取一次文件。
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.