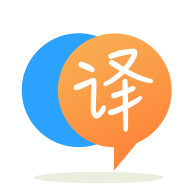
[英]Java: Creating a frequency table for integers randomly generated and placed in an array
[英]Java Array of unique randomly generated integers
public static int[] uniqueRandomElements (int size) {
int[] a = new int[size];
for (int i = 0; i < size; i++) {
a[i] = (int)(Math.random()*10);
for (int j = 0; j < i; j++) {
if (a[i] == a[j]) {
a[j] = (int)(Math.random()*10);
}
}
}
for (int i = 0; i < a.length; i++) {
System.out.print(a[i]+" ");
}
System.out.println();
return a;
}
我上面有一個方法,它應該生成用戶指定的隨機元素數組。 隨機生成的整數應介於 0 和 10 之間。 我能夠生成隨機整數,但我遇到的問題是檢查唯一性。 我在上面的代碼中嘗試檢查唯一性,但數組仍然包含整數的重復項。 我做錯了什么,有人可以給我一個提示嗎?
for (int i = 0; i < size; i++) {
a[i] = (int)(Math.random()*10);
for (int j = 0; j < i; j++) {
if (a[i] == a[j]) {
a[j] = (int)(Math.random()*10); //What's this! Another random number!
}
}
}
您確實找到了重復值。 但是,您將其替換為另一個可能重復的隨機數。 相反,試試這個:
for (int i = 0; i < size; i++) {
a[i] = (int)(Math.random()*10);//note, this generates numbers from [0,9]
for (int j = 0; j < i; j++) {
if (a[i] == a[j]) {
i--; //if a[i] is a duplicate of a[j], then run the outer loop on i again
break;
}
}
}
然而,這種方法效率低下。 我建議制作一個數字列表,然后將其隨機化:
ArrayList<Integer> a = new ArrayList<>(11);
for (int i = 0; i <= 10; i++){ //to generate from 0-10 inclusive.
//For 0-9 inclusive, remove the = on the <=
a.add(i);
}
Collections.shuffle(a);
a = a.sublist(0,4);
//turn into array
或者你可以這樣做:
ArrayList<Integer> list = new ArrayList<>(11);
for (int i = 0; i <= 10; i++){
list.add(i);
}
int[] a = new int[size];
for (int count = 0; count < size; count++){
a[count] = list.remove((int)(Math.random() * list.size()));
}
如果您有重復,則只能重新生成相應的數字一次。 但它可能會創建另一個副本。 你重復的檢查代碼應該包含在一個循環中:
while (true) {
boolean need_to_break = true;
for (int j = 0; j < i; j++) {
if (a[i] == a[j]) {
need_to_break = false; // we might get another conflict
a[j] = (int)(Math.random()*10);
}
}
if (need_to_break) break;
}
但請確保size
小於10
,否則您將獲得無限循環。
編輯:雖然上述方法解決了問題,但效率不高,不應用於大型數組。 此外,這對完成所需的迭代次數沒有保證的上限。
一個更好的解決方案(不幸的是只解決了第二點)可能是生成一個你想要生成的不同數字的序列( 10
數字),隨機排列這個序列,然后只選擇該序列的第一個size
元素並將它們復制到你的陣列。 你會用一些空間換取時間范圍內的保證。
int max_number = 10;
int[] all_numbers = new int[max_number];
for (int i = 0; i < max_number; i++)
all_numbers[i] = i;
/* randomly permute the sequence */
for (int i = max_number - 1; i >= 0; i--) {
int j = (int)(Math.random() * i); /* pick a random number up to i */
/* interchange the last element with the picked-up index */
int tmp = all_numbers[j];
all_numbers[j] = a[i];
all_numbers[i] = tmp;
}
/* get the a array */
for (int i = 0; i < size; i++)
a[i] = all_numbers[i];
或者,您可以創建一個具有相同數字的ArrayList
,而不是中間循環,您可以在其上調用Collections.shuffle()
。 然后,你仍舊需要第三環獲得元素融入a
。
從順序數組開始並對其進行洗牌可能會更快。 那么它們在定義上都是獨一無二的。
看一看Random shuffle of an array和 Collections.shuffle 函數。
int [] arr = [1,2,3,.....(size)]; //this is pseudo code
Collections.shuffle(arr);// you probably need to convert it to list first
如果您只是不想為 ArrayList 的額外開銷付費,您可以只使用一個數組並使用Knuth shuffle :
public Integer[] generateUnsortedIntegerArray(int numElements){
// Generate an array of integers
Integer[] randomInts = new Integer[numElements];
for(int i = 0; i < numElements; ++i){
randomInts[i] = i;
}
// Do the Knuth shuffle
for(int i = 0; i < numElements; ++i){
int randomIndex = (int)Math.floor(Math.random() * (i + 1));
Integer temp = randomInts[i];
randomInts[i] = randomInts[randomIndex];
randomInts[randomIndex] = temp;
}
return randomInts;
}
上面的代碼生成 numElements 個連續整數,沒有以均勻隨機打亂順序重復。
import java.util.Scanner;
class Unique
{
public static void main(String[]args)
{
int i,j;
Scanner in=new Scanner(System.in);
int[] a=new int[10];
System.out.println("Here's a unique no.!!!!!!");
for(i=0;i<10;i++)
{
a[i]=(int)(Math.random()*10);
for(j=0;j<i;j++)
{
if(a[i]==a[j])
{
i--;
}
}
}
for(i=0;i<10;i++)
{
System.out.print(a[i]);
}
}
}
使用 Collections 輸入您的尺寸並獲取隨機唯一數字列表。
public static ArrayList<Integer> noRepeatShuffleList(int size) {
ArrayList<Integer> arr = new ArrayList<>();
for (int i = 0; i < size; i++) {
arr.add(i);
}
Collections.shuffle(arr);
return arr;
}
詳細說明 Karthik 的回答。
int[] a = new int[20];
for (int i = 0; i < size; i++) {
a[i] = (int) (Math.random() * 20);
for (int j = 0; j < i; j++) {
if (a[i] == a[j]) {
a[i] = (int) (Math.random() * 20); //What's this! Another random number!
i--;
break;
}
}
}
int[] a = new int [size];
for (int i = 0; i < size; i++)
{
a[i] = (int)(Math.random()*16); //numbers from 0-15
for (int j = 0; j < i; j++)
{
//Instead of the if, while verifies that all the elements are different with the help of j=0
while (a[i] == a[j])
{
a[i] = (int)(Math.random()*16); //numbers from 0-15
j=0;
}
}
}
for (int i = 0; i < a.length; i++)
{
System.out.println(i + ". " + a[i]);
}
//Initialize array with 9 elements
int [] myArr = new int [9];
//Creating new ArrayList of size 9
//and fill it with number from 1 to 9
ArrayList<Integer> myArrayList = new ArrayList<>(9);
for (int i = 0; i < 9; i++) {
myArrayList.add(i + 1);
}
//Using Collections, I shuffle my arrayList
Collections.shuffle(myArrayList);
//With for loop and method get() of ArrayList
//I fill my array
for(int i = 0; i < myArrayList.size(); i++){
myArr[i] = myArrayList.get(i);
}
//printing out my array
for(int i = 0; i < myArr.length; i++){
System.out.print(myArr[i] + " ");
}
你可以試試這個解決方案:
public static int[] uniqueRandomElements(int size) {
List<Integer> numbers = IntStream.rangeClosed(0, size).boxed().collect(Collectors.toList());
return Collections.shuffle(numbers);
}
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.