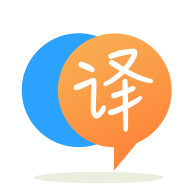
[英]Python and remove duplicates in list of lists regardless of order within lists
[英]Python: Determine if an unsorted list is contained in a 'list of lists', regardless of the order to the elements
我對此問題有一個類似的問題: 確定2個列表是否具有相同的元素,而不管順序如何?
確定“列表列表” myListOfLists
是否包含未排序列表list1
的最佳/最快方法是myListOfLists
,而不管list1
元素的順序如何? 我的嘗試被包裝在函數doSomething(...)
,該函數多次調用:
def doSomething(myListOfLists, otherInputs):
list1 = []
... # do something here with `otherInputs'
... # which gives `list1' some values
# now only append `list1' to `myListOfLists' if it doesn't already exist
# and if it does exist, remove it
removeFromList = False
for myList in myListOfLists:
if sorted(list1) == sorted(myList):
removeFromList = True
break
if removeFromList:
myListOfLists.remove(list1)
else:
myListOfLists.append(list1)
return myListOfLists
問題是我需要運行函數doSomething(...)
大約1.0e5次。 由於每次調用doSomething(...)
myListOfLists
都會變大,因此變得非常耗時。
編輯:
一些任務的澄清。 讓我在此處給出所需輸出的示例:
a = []
doSomething(a, [1,2,3])
>> a = [1,2,3]
因為[1,2,3]
不是a
,它被附加到a
。
doSomething(a, [3,4,5])
>> a = [[1,2,3], [3,4,5]]
因為[3,4,5]
不是a
,它被附加到a
。
doSomething(a, [1,2,3])
>>[3,4,5]
因為[1,2,3]
是在a
,從移除a
。
編輯:
所有列表的長度相同。
您可以在此處使用集:
def doSomething(myListOfLists, otherInputs):
s = set(otherInputs) #create set from otherInputs
for item in myListOfLists:
#remove the common items between `s` and current sublist from `s`.
s -= s.intersection(item)
#if `s` is empty, means all items found. Return True
if not s:
return True
return not bool(s)
...
>>> doSomething([[1, 2, 7],[6, 5, 4], [10, 9, 10]], [7, 6, 8])
False
>>> doSomething([[1, 2, 7],[6, 5, 4], [10, 8, 10]], [7, 6, 8])
True
更新1: otherInputs
包含與otherInputs
完全相同的項。
def doSomething(myListOfLists, otherInputs):
s = set(otherInputs)
return any(set(item) == s for item in myListOfLists)
...
>>> doSomething([[6, 8, 7],[6, 5, 4], [10, 8, 10]], [7, 6, 8])
True
>>> doSomething([[1, 2, 7],[6, 5, 4], [10, 8, 10]], [7, 6, 8])
False
更新2: otherInputs
是任何子列表的子集:
def doSomething(myListOfLists, otherInputs):
s = set(otherInputs)
return any(s.issubset(item) for item in myListOfLists)
...
>>> doSomething([[6, 8, 7],[6, 5, 4], [10, 8, 10]], [7, 6, 8])
True
>>> doSomething([[6, 8, 7, 10],[6, 5, 4], [10, 8, 10]], [7, 6, 8])
True
>>> doSomething([[1, 2, 7],[6, 5, 4], [10, 8, 10]], [7, 6, 8])
False
使用集
def doSomething(myDictOfLists, otherInputs):
list1 = []
... # do something here with `otherInputs'
... # which gives `list1' some values
# now only append `list1' to `myListOfLists' if it doesn't already exist
# and if it does exist, remove it
list1Set = set(list1)
if list1Set not in myDictOfLists:
myDictOfLists[list1Set] = list1
return myDictOfLists
如果對給定列表進行排序,然后將其附加到myListOfLists
,則可以使用以下命令:
if sorted(list1) in myListOfLists:
該算法似乎更快一些:
l1 = [3, 4, 1, 2, 3]
l2 = [4, 2, 3, 3, 1]
same = True
for i in l1:
if i not in l2:
same = False
break
對於1000000循環,這在我的計算機上花費1.25399184227秒,而
same = sorted(l1) == sorted(l2)
需要1.9238319397秒。
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.