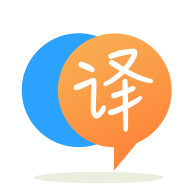
[英]How to share variables between two functions without declaring it a global variable in C language
[英]How to share a variable between two functions in C?
在C中,假設var1是foo1()中的一個變量,foo2()想要訪問var1,但是foo1()並沒有調用foo2(),所以我們不能通過參數傳遞它。 同時,只有 foo1() 和 foo2() 會訪問它,所以我不想將它聲明為全局變量。 它將類似於 C++ 中的“朋友語義”,有沒有辦法在 C 中實現它?
void foo1() {
...
var1;
....
}
void foo2() {
...
how to access var1?
...
}
您將變量傳遞給兩個函數......一般來說,函數不應該保持狀態。
很快你就會發現傳遞變量不是那么好並且變得脆弱,所以相反,你傳遞結構......然后函數開始處理結構的狀態。
typedef struct
{
int var1;
} blah_t;
void foo1(blah_t* b)
{
b->var1=0;
}
void foo2(blah_t* b)
{
b->var1++;
}
這是做 OO C 背后的非常簡單的種子想法。
您需要在函數范圍之外聲明var1
,然后將其作為參數發送給兩者。 或者,將其聲明為全局變量。
通過引用是一種方式:(在這個例子中, i
的內存是caller()
本地的)
void caller()
{
int i = 5;
foo(&i);
bar(&i);
printf("\n final i is %d",i);
}
void foo(int *i)
{
printf("%d",*i);
*i += 5;
}
void bar (int *i)
{
printf("%d",*i);
*i += 5;
}
全球:(通常被認為是可怕的, i
會有一個更像GLOBAL_I
類的名字)
int i = 0;
void caller()
{
i=5;
foo();
bar();
printf("\n final i is %d",i);
}
void foo()
{
printf("%d",i);
i += 5;
}
void bar (int i)
{
printf("%d",i);
i += 5;
}
關於類似於 c++ 中的“朋友語義” 。 C 沒有相同的能力。
關於所以我們不能通過參數傳遞它
在C
中從一個函數訪問一個變量而不作為函數參數傳遞的唯一選擇是使用某種類型的全局范圍變量。
如果void foo1()
和void foo2()
存在於不同的 C 模塊中...
但您仍然希望能夠訪問相同的變量,並確保其值在項目中的所有位置始終相同,然后考慮使用extern scope
:
在兩個(多個)模塊共有的頭文件中,項目范圍全局可以如下實現。
文件.h
void foo1(void);
void foo2(void);
extern int var1;
文件1.c
#include "file.h"
int var1 = 5; //in only 1 module, declare and initialize the
//extern defined in the common header -file.h-
int main(void)
{
printf("value of var1 is %d\n", var1);//original value of var1
foo1();
printf("value of var1 is %d\n", var1);//var1 modified by foo1()
foo2();
printf("value of var1 is %d\n", var1);//var1 modified by foo2()
return 0;
}
void foo1(void)
{
var1 = 15;//Now that the project global variable
//has already been declared and defined, it can simply
//be used, in this file...
}
文件2.c
#include "file.h"
void foo2(void)
{
var1 = 20;... and in this one
}
不。該變量僅在foo1()
運行時存在於函數堆棧中。 離開函數時堆棧將消失。 您可以將變量設為靜態以使其保持活動狀態,但是如果沒有黑客攻擊,您也無法從外部訪問它。
這個答案的靈感來自許多其他語言中的“模塊”概念,可以使用 gcc 的嵌套函數來近似。 變量var1
在foo1()
和foo2()
的范圍內,但在其他所有范圍之外。 該解決方案既不使用全局變量也不使用參數。
void foo(int fn)
{
static int var1;
void fn1(void)
{
var1 = 15;
}
void fn2(void)
{
var1 = 20;
}
(fn == 1)? fn1(): fn2();
printf("Value of var1 is now %d\n", var1);
}
void foo1(void){foo(1);}
void foo2(void){foo(2);}
int main (void)
{
foo1();
// Expected stdout: Value of var1 is now 15
foo2();
// Expected stdout: Value of var1 is now 20
}
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.