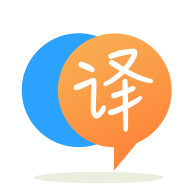
[英]NullPointerException when registering a new command in main class
[英]NullPointerException when registering the Listeners
Plase看看下面的代碼
MainActivity.java
public class MainActivity extends Activity {
private TextView words;
private Map<String, String> wordsMap;
private List wordList;
private Animation animation1, animation2;
private LinearLayout cards;
private int displayIndex = 0;
private static final int ENGLISH = 1;
private static final int BRAZIL = 2;
private int languageDisplaying;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
words = (TextView)findViewById(R.id.word);
cards = (LinearLayout)findViewById(R.id.cards_layout);
languageDisplaying = ENGLISH;
animation1 = AnimationUtils.loadAnimation(this, R.anim.to_middle);
animation2 = AnimationUtils.loadAnimation(this, R.anim.from_middle);
wordsMap = new HashMap<String, String>();
wordsMap.put("Dog", "Perro");
wordsMap.put("Cat", "Gato");
wordsMap.put("Universe", "Universo");
wordsMap.put("Telephone", "Teléfono");
wordsMap.put("KeyBoard", "TecladoDel");
wordsMap.put("Country", "País");
wordList = new ArrayList<String>();
wordList.add("Dogsssssssssss");
wordList.add("Cat");
wordList.add("Universe");
wordList.add("Telephone");
wordList.add("KeyBoard");
wordList.add("Country");
words.setText(wordList.get(0).toString());
//Registering Listeners
words.setOnClickListener(new TextClicked());
cards.setOnClickListener(new CardsClicked());
animation1.setAnimationListener(new AnimationEvent());
}
@Override
public boolean onCreateOptionsMenu(Menu menu) {
// Inflate the menu; this adds items to the action bar if it is present.
getMenuInflater().inflate(R.menu.main, menu);
return true;
}
//Animation Listener
private class AnimationEvent implements AnimationListener
{
String str = "";
@Override
public void onAnimationEnd(Animation arg0) {
// TODO Auto-generated method stub
if(languageDisplaying==ENGLISH)
{
str = wordList.get(displayIndex).toString();
words.setText(wordsMap.get(str));
words.setTextColor(Color.BLUE);
languageDisplaying = BRAZIL;
}
else
{
str = wordList.get(displayIndex).toString();
words.setText(str);
words.setTextColor(Color.BLACK);
languageDisplaying = ENGLISH;
}
}
@Override
public void onAnimationRepeat(Animation animation) {
// TODO Auto-generated method stub
}
@Override
public void onAnimationStart(Animation animation) {
// TODO Auto-generated method stub
}
}
private OnTouchListener textViewSwiped = new OnSwipeTouchListener()
{
public boolean onSwipeLeft()
{
return true;
}
};
private class TextClicked implements OnClickListener
{
@Override
public void onClick(View v) {
// TODO Auto-generated method stub
words.startAnimation(animation1);
}
}
private class CardsClicked implements View.OnClickListener
{
@Override
public void onClick(View v) {
// TODO Auto-generated method stub
//showMenu();
}
}
private void showMenu()
{
final Dialog dialog = new Dialog(this);
dialog.setContentView(R.layout.glass_menu);
dialog.show();
}
}
activity_main.xml中
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical"
android:gravity="center"
android:paddingBottom="@dimen/activity_vertical_margin"
android:paddingLeft="@dimen/activity_horizontal_margin"
android:paddingRight="@dimen/activity_horizontal_margin"
android:paddingTop="@dimen/activity_vertical_margin"
tools:context=".MainActivity" >
<TextView
android:id="@+id/textView1"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginLeft="202dp"
android:textAppearance="?android:attr/textAppearanceMedium"
android:text="100 de 554" />
<LinearLayout
android:id="@+id/cards_layout"
android:layout_width="300dp"
android:layout_height="100dp"
android:background="@drawable/round_corners"
android:clickable="true"
android:gravity="center">
<TextView
android:id="@+id/word"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_gravity="center"
android:gravity="center"
android:text="Text"
android:textSize="50sp" />
</LinearLayout>
</LinearLayout>
當我運行它時,我得到一個NullPointerException
。 錯誤發生在這個地方。
cards.setOnClickListener(new CardsClicked());
以下是錯誤日志
12-09 06:05:24.040: E/AndroidRuntime(2424): FATAL EXCEPTION: main
12-09 06:05:24.040: E/AndroidRuntime(2424): java.lang.RuntimeException: Unable to start activity ComponentInfo{com.example.googleglass_demo/com.example.googleglass_demo.MainActivity}: java.lang.NullPointerException
12-09 06:05:24.040: E/AndroidRuntime(2424): at android.app.ActivityThread.performLaunchActivity(ActivityThread.java:2180)
12-09 06:05:24.040: E/AndroidRuntime(2424): at android.app.ActivityThread.handleLaunchActivity(ActivityThread.java:2230)
12-09 06:05:24.040: E/AndroidRuntime(2424): at android.app.ActivityThread.access$600(ActivityThread.java:141)
12-09 06:05:24.040: E/AndroidRuntime(2424): at android.app.ActivityThread$H.handleMessage(ActivityThread.java:1234)
12-09 06:05:24.040: E/AndroidRuntime(2424): at android.os.Handler.dispatchMessage(Handler.java:99)
12-09 06:05:24.040: E/AndroidRuntime(2424): at android.os.Looper.loop(Looper.java:137)
12-09 06:05:24.040: E/AndroidRuntime(2424): at android.app.ActivityThread.main(ActivityThread.java:5041)
12-09 06:05:24.040: E/AndroidRuntime(2424): at java.lang.reflect.Method.invokeNative(Native Method)
12-09 06:05:24.040: E/AndroidRuntime(2424): at java.lang.reflect.Method.invoke(Method.java:511)
12-09 06:05:24.040: E/AndroidRuntime(2424): at com.android.internal.os.ZygoteInit$MethodAndArgsCaller.run(ZygoteInit.java:793)
12-09 06:05:24.040: E/AndroidRuntime(2424): at com.android.internal.os.ZygoteInit.main(ZygoteInit.java:560)
12-09 06:05:24.040: E/AndroidRuntime(2424): at dalvik.system.NativeStart.main(Native Method)
12-09 06:05:24.040: E/AndroidRuntime(2424): Caused by: java.lang.NullPointerException
12-09 06:05:24.040: E/AndroidRuntime(2424): at com.example.googleglass_demo.MainActivity.onCreate(MainActivity.java:71)
12-09 06:05:24.040: E/AndroidRuntime(2424): at android.app.Activity.performCreate(Activity.java:5104)
12-09 06:05:24.040: E/AndroidRuntime(2424): at android.app.Instrumentation.callActivityOnCreate(Instrumentation.java:1080)
12-09 06:05:24.040: E/AndroidRuntime(2424): at android.app.ActivityThread.performLaunchActivity(ActivityThread.java:2144)
12-09 06:05:24.040: E/AndroidRuntime(2424): ... 11 more
導致此錯誤的原因是什么?
更新:
有兩種可能發生的可能性:
嘗試清理並重建項目,並檢查是否沒有類似的文件。
我編譯你的代碼,工作正常。 當我使用相同的@id(card_layout)創建類似的文件時,我再現了錯誤,從xml綁定到Activity中刪除它
05-02 09:06:19.514: ERROR/AndroidRuntime(519): FATAL EXCEPTION: main
java.lang.RuntimeException: Unable to start activity ComponentInfo{com.example.stacktest/com.example.stacktest.MyActivity}: java.lang.NullPointerException
at android.app.ActivityThread.performLaunchActivity(ActivityThread.java:2663)
at android.app.ActivityThread.handleLaunchActivity(ActivityThread.java:2679)
at android.app.ActivityThread.access$2300(ActivityThread.java:125)
at android.app.ActivityThread$H.handleMessage(ActivityThread.java:2033)
at android.os.Handler.dispatchMessage(Handler.java:99)
at android.os.Looper.loop(Looper.java:123)
at android.app.ActivityThread.main(ActivityThread.java:4627)
at java.lang.reflect.Method.invokeNative(Native Method)
at java.lang.reflect.Method.invoke(Method.java:521)
at com.android.internal.os.ZygoteInit$MethodAndArgsCaller.run(ZygoteInit.java:868)
at com.android.internal.os.ZygoteInit.main(ZygoteInit.java:626)
at dalvik.system.NativeStart.main(Native Method)
Caused by: java.lang.NullPointerException
at com.example.stacktest.MyActivity.onCreate(MyActivity.java:38)
at android.app.Instrumentation.callActivityOnCreate(Instrumentation.java:1047)
at android.app.ActivityThread.performLaunchActivity(ActivityThread.java:2627)
... 11 more
舊
你確定它不在下面嗎? 因為我沒有看到animation1變量的任何初始化。
animation1.setAnimationListener(new AnimationEvent());
試試這個改變:
private class CardsClicked implements View.OnClickListener
你的Animation animation1, animation2;
如果為null,則表示您尚未初始化實例的動畫。 這就是它給出空指針異常的原因。
確保在開始動畫之前給出動畫文件,如下所示:
// load the animation
animation1= AnimationUtils.loadAnimation(getApplicationContext(),
R.anim.<yourfileName>);
animation1.setAnimationListener(new AnimationEvent());
我希望你已經定義了一個偵聽器new AnimationEvent()
並實現了它的方法來啟動動畫。 因為您還沒有共享此偵聽器的任何代碼。
我發現了這個問題。 Landscape布局由layout-land
文件夾中的XML文件控制。 在該文件夾中,未提供LinearLayout
的ID。
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.