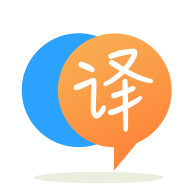
[英]How do I get the cursor to the TOP of a JTextArea inside a JScrollPane
[英]How do I update the text of a JTextArea inside of a JScrollPane?
正如問題所指出的,我在更新JScrollPane內部的JTextArea的文本時遇到了麻煩。
我能夠從JScrollPane的類型轉換getView()中獲取文本。 但是,我嘗試了以下更新JTextArea的方法。
((JTextArea)(chatWindow.getViewport().getView())).setText("Hello!");
其中chatWindow是JScrollPane。
我已經嘗試過了
chatWindowInsert.setText(processMessage());
其中chatWindowInsert是JScrollPane中的JTextArea
不幸的是,這兩個工作都沒有。
我沒有任何例外或懸空。
協助將不勝感激!
這是我完整的代碼。 如果我違反了一百萬種編程實踐,請原諒我。
public class ChatterBotClient extends JFrame{
/**
*
*/
private static final long serialVersionUID = 1L;
private static ChatterBotFactory chatterBotFactory;
private static ChatterBotSession chatterBotSession;
private static ChatterBot chatterBot;
public static JScrollPane chatWindow;
public static JTextField userInput;
public static JTextArea chatWindowInsert;
public ChatterBotClient()
{
try{
chatterBotFactory = new ChatterBotFactory();
chatterBot = chatterBotFactory.create(ChatterBotType.CLEVERBOT);
chatterBotSession = chatterBot.createSession();
}catch(Exception e)
{
e.printStackTrace();
System.out.println("Error :O");
JOptionPane.showMessageDialog(null,
"There has been a problem initializing the Bot. Please restart.");
}
initUI();
}
public void initUI()
{
//Define the mainPanel that everything goes into in the JFrame
JPanel mainPanel = new JPanel(new BorderLayout());
//Define the child panels of "mainPanel"
JPanel infoPanel = new JPanel(new FlowLayout());
JPanel chatHistoryPanel = new JPanel(new FlowLayout());
JPanel userInputAndButtonPanel = new JPanel(new FlowLayout());
setTitle("ChatterBot Chat Client");
setSize(600,300);
setResizable(false);
//Define each component
//Set properties of each component
JLabel infoLabel = new JLabel("Welcome to my Cleverbot Client! Please enjoy! :D");
infoLabel.setPreferredSize(new Dimension(550, 20));
infoLabel.setHorizontalTextPosition(SwingConstants.CENTER);
chatWindowInsert = new JTextArea();
chatWindowInsert.setWrapStyleWord(true);
chatWindow = new JScrollPane(chatWindowInsert);
chatWindow.setPreferredSize(new Dimension(500,225));
chatWindow.setPreferredSize(chatWindow.getPreferredSize());
userInput = new JTextField();
userInput.setPreferredSize(new Dimension(250, 25));
JButton enterBtn = new JButton("Send");
enterBtn.setPreferredSize(new Dimension(75, 25));
//Add each component to the required panels
infoPanel.add(infoLabel);
chatHistoryPanel.add(chatWindow);
userInputAndButtonPanel.add(userInput, BorderLayout.CENTER);
userInputAndButtonPanel.add(enterBtn, BorderLayout.EAST);
//Add the child panels to the mainPanel
mainPanel.add(infoPanel, BorderLayout.NORTH);
mainPanel.add(chatHistoryPanel, BorderLayout.CENTER);
mainPanel.add(userInputAndButtonPanel, BorderLayout.SOUTH);
//Now, add the appropriate listeners to your components
enterBtn.addActionListener(new ActionListener(){
@Override
public void actionPerformed(ActionEvent arg0) {
System.out.println("You clicked me! :D");
try {
processMessage();
} catch (Exception e) {
// TODO Auto-generated catch block
e.printStackTrace();
System.out.println("Something went wrong when you pressed the button! :O");
}
}
});
userInput.addActionListener(new ActionListener(){
@Override
public void actionPerformed(ActionEvent arg0)
{
chatWindow = new JScrollPane(new JTextArea(processMessage()));
}
});
//Tell the JFrame to display what we've made!
add(mainPanel);
}
public static String processMessage()
{
try {
String completeMessage = chatWindowInsert.getText();
completeMessage.concat("You: " + userInput.getText() + "\n");
String response = chatterBotSession.think(userInput.getText());
completeMessage.concat("Bot: " + response + "\n");
userInput.setText("");
return completeMessage;
} catch (Exception e) {
// TODO Auto-generated catch block
e.printStackTrace();
return "ERROR";
}
}
public static void main(String[] args)
{
SwingUtilities.invokeLater(new Runnable()
{
@Override
public void run()
{
ChatterBotClient cli = new ChatterBotClient();
cli.setVisible(true);
}
});
}
}
您聲明:
正如問題所指出的,我在更新JScrollPane內部的JTextArea的文本時遇到了麻煩。
我能夠從JScrollPane的類型轉換getView()中獲取文本。 但是,我嘗試了以下更新JTextArea的方法。
((JTextArea)(chatWindow.getViewport().getView())).setText("Hello!");
為什么要經歷所有這些易碎的代碼體操? 創建一個類級別的JTextArea實例字段會更簡單,更安全,更輕松,然后在JScrollPane中的GUI中顯示它,然后簡單地在該實例上獲取文本或設置文本。 沒有混亂,沒有大驚小怪,沒有錯誤。
如果您當前的程序無法做到這一點,那么您對我們的建議還不夠多,否則您將需要告訴我們更多信息。
編輯
回復您的評論:
具有同時引用JTextArea和JTextField的變量(只是為了弄清楚這一點),但是即使我使用上述適當的方法之一更改了任何一個變量,JTextArea內部的內容仍然不會改變。 除非我不明白你在說什么?
這表明,盡管您可能正在使用正確的變量,但您可能正在使用錯誤的引用。 也許您正在使用的變量沒有保存在當前顯示的GUI中。
但這只是SWAG工作(愚蠢的野驢猜測工作)而已。 請不要強迫我們猜測-編輯您的原始文章並向我們展示您的代碼(最好是sscce) ,向我們展示如何獲取這些變量的句柄以及如何知道它們屬於所顯示的 gui。
編輯2
關於您的最新代碼。 讓我們看一下這一行:
public static JTextArea chatWindowInsert;
編輯3
我現在看到,您將完全不同的JTextField放入了JScrollPane中,再也沒有將其放入GUI了,並且所有這些操作都可以在所有位置進行!
userInput.addActionListener(new ActionListener(){
@Override
public void actionPerformed(ActionEvent arg0)
{
chatWindow = new JScrollPane(new JTextArea(processMessage()));
}
});
建議:
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.