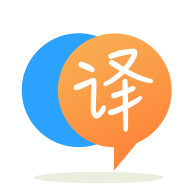
[英]regular expression alphanumeric characters - allowing hyphens and underscores
[英]Regular Expression for alphanumeric and underscores
我想要一個正則表達式來檢查字符串是否只包含大寫和小寫字母、數字和下划線。
要匹配僅包含這些字符(或空字符串)的字符串,請嘗試
"^[a-zA-Z0-9_]*$"
這適用於 .NET 正則表達式,可能也適用於許多其他語言。
分解它:
^ : start of string
[ : beginning of character group
a-z : any lowercase letter
A-Z : any uppercase letter
0-9 : any digit
_ : underscore
] : end of character group
* : zero or more of the given characters
$ : end of string
如果您不想允許空字符串,請使用 + 而不是 *。
正如其他人指出的那樣,一些正則表達式語言有[a-zA-Z0-9_]
的簡寫形式。 在 .NET regex 語言中,您可以打開 ECMAScript 行為並使用\\w
作為速記(產生^\\w*$
或^\\w+$
)。 請注意,在其他語言中,默認情況下,在 .NET 中, \\w
范圍更廣,並且也會匹配其他類型的 Unicode 字符(感謝 Jan 指出這一點)。 因此,如果您真的只想匹配這些字符,那么使用顯式(更長)的形式可能是最好的。
這里有很多冗長,我非常反對,所以,我的結論性答案是:
/^\w+$/
\\w
相當於[A-Za-z0-9_]
,這幾乎是您想要的。 (除非我們在混合中引入 unicode)
使用+
量詞,您將匹配一個或多個字符。 如果您也想接受空字符串,請改用*
。
您想檢查每個字符是否符合您的要求,這就是我們使用的原因:
[A-Za-z0-9_]
你甚至可以使用速記版本:
\w
這是等效的(在某些正則表達式中,因此請確保在使用前進行檢查)。 然后要指示整個字符串必須匹配,請使用:
^
要指示字符串必須以該字符開頭,然后使用
$
表示字符串必須以該字符結尾。 然后使用
\w+ or \w*
表示“1 或更多”,或“0 或更多”。 綜合起來,我們有:
^\w*$
嗯...問題:它是否需要至少有一個字符? 它可以是一個空字符串嗎?
^[A-Za-z0-9_]+$
將至少做一個大寫或小寫字母數字或下划線。 如果它可以是零長度,那么只需將 + 替換為 *
^[A-Za-z0-9_]*$
編輯:
如果需要包含變音符號(例如 cedilla - ç),那么您需要使用與上述相同的單詞 character,但包括變音符號:
^\w+$
或者
^\w*$
雖然它比\\w
更冗長,但我個人欣賞完整 POSIX 字符類名稱( http://www.zytrax.com/tech/web/regex.htm#special )的可讀性,所以我會說:
^[[:alnum:]_]+$
但是,雖然上述鏈接中的文檔指出\\w
將“匹配 0 - 9、A - Z 和 a - z(相當於 POSIX [:alnum:]])范圍內的任何字符”,但我還沒有發現這一點是真實的。 無論如何都不能使用grep -P
。 如果使用[:alnum:]
,則需要明確包含下划線,但如果使用\\w
則不需要。 您無法通過簡短而甜蜜的方式擊敗以下內容:
^\w+$
除了可讀性之外,使用 POSIX 字符類 ( http://www.regular-expressions.info/posixbrackets.html ) 意味着您的正則表達式可以處理非 ASCII 字符串,而基於范圍的正則表達式不會這樣做,因為它們依賴ASCII 字符的基本順序可能與其他字符集不同,因此將排除一些您可能想要捕獲的非 ASCII 字符(例如 – 字母)。
怎么樣:
^([A-Za-z]|[0-9]|_)+$
...如果你想明確,或者:
^\w+$
...如果你喜歡簡潔(Perl 語法)。
在計算機科學中,字母數字值通常表示第一個字符不是數字而是字母或下划線。 此后,字符可以是0-9
、 AZ
、 az
或下划線 ( _
)。
以下是您將如何做到這一點:
在php下測試:
$regex = '/^[A-Za-z_][A-Za-z\d_]*$/'
或者拿這個
^[A-Za-z_][A-Za-z\d_]*$
並將其放入您的開發語言中。
使用前瞻來做“至少一個”的事情。 相信我,這要容易得多。
這是一個需要 1-10 個字符的示例,其中至少包含一位數字和一個字母:
^(?=.*\d)(?=.*[A-Za-z])[A-Za-z0-9]{1,10}$
注意:本可以使用 \\w 但隨后 ECMA/Unicode 考慮會增加 \\w “單詞字符”的字符覆蓋率。
這對我有用,在 O'Reilly 的“掌握正則表達式”中找到了這個:
/^\w+$/
解釋:
驗證自己:
const regex = /^\\w+$/; const str = `nut_cracker_12`; let m; if ((m = regex.exec(str)) !== null) { // The result can be accessed through the `m`-variable. m.forEach((match, groupIndex) => { console.log(`Found match, group ${groupIndex}: ${match}`); }); }
試試我為字符串制作的這些多語言擴展。
IsAlphaNumeric - 字符串必須包含至少 1 個字母(Unicode 范圍內的字母,在 charSet 中指定)和至少 1 個數字(在 numSet 中指定)。 此外,字符串應僅包含字母和數字。
IsAlpha - 字符串應至少包含 1 個 alpha(在指定的語言字符集中)並且僅包含 alpha。
IsNumeric - 字符串應至少包含 1 個數字(在指定的語言 numSet 中)並且僅包含數字。
可以指定所需語言的 charSet/numSet 范圍。 Unicode 范圍可在以下鏈接中找到:
http://www.ssec.wisc.edu/~tomw/java/unicode.html
應用程序接口:
public static bool IsAlphaNumeric(this string stringToTest)
{
//English
const string charSet = "a-zA-Z";
const string numSet = @"0-9";
//Greek
//const string charSet = @"\u0388-\u03EF";
//const string numSet = @"0-9";
//Bengali
//const string charSet = @"\u0985-\u09E3";
//const string numSet = @"\u09E6-\u09EF";
//Hindi
//const string charSet = @"\u0905-\u0963";
//const string numSet = @"\u0966-\u096F";
return Regex.Match(stringToTest, @"^(?=[" + numSet + @"]*?[" + charSet + @"]+)(?=[" + charSet + @"]*?[" + numSet + @"]+)[" + charSet + numSet +@"]+$").Success;
}
public static bool IsNumeric(this string stringToTest)
{
//English
const string numSet = @"0-9";
//Hindi
//const string numSet = @"\u0966-\u096F";
return Regex.Match(stringToTest, @"^[" + numSet + @"]+$").Success;
}
public static bool IsAlpha(this string stringToTest)
{
//English
const string charSet = "a-zA-Z";
return Regex.Match(stringToTest, @"^[" + charSet + @"]+$").Success;
}
用法 :
//English
string test = "AASD121asf";
//Greek
//string test = "Ϡϛβ123";
//Bengali
//string test = "শর৩৮";
//Hindi
//string test = @"क़लम३७ख़";
bool isAlphaNum = test.IsAlphaNumeric();
以下正則表達式匹配字母數字字符和下划線:
^[a-zA-Z0-9_]+$
例如,在 Perl 中:
#!/usr/bin/perl -w
my $arg1 = $ARGV[0];
# check that the string contains *only* one or more alphanumeric chars or underscores
if ($arg1 !~ /^[a-zA-Z0-9_]+$/) {
print "Failed.\n";
} else {
print "Success.\n";
}
這應該適用於大多數情況。
/^[\\d]*[a-z_][az\\d_]*$/gi
我的意思是,
abcd True
abcd12 True
ab12cd True
12abcd True
1234 False
^ ... $
- 匹配開始和結束的模式[\\d]*
- 匹配零個或多個數字[a-z_]
- 匹配字母或下划線[az\\d_]*
- 匹配字母或數字或下划線/gi
- 跨字符串全局匹配且不區分大小寫對我來說,有一個問題是我想區分字母、數字和字母數字,因此為了確保字母數字字符串包含至少一個字母和至少一個數字,我使用了:
^([a-zA-Z_]{1,}\d{1,})+|(\d{1,}[a-zA-Z_]{1,})+$
對於那些正在尋找 unicode 字母數字匹配的人,您可能想要執行以下操作:
^[\p{L} \p{Nd}_]+$
進一步閱讀http://unicode.org/reports/tr18/和http://www.regular-expressions.info/unicode.html
這是您想要使用量詞指定至少 1 個字符且不超過 255 個字符的正則表達式
[^a-zA-Z0-9 _]{1,255}
我相信您沒有在比賽中使用拉丁語和 Unicode 字符。 例如,如果您需要使用 "ã" 或 "ü" 字符,則使用 "\\w" 將不起作用。
或者,您可以使用這種方法:
^[A-ZÀ-Ýa-zà-ý0-9_]+$
希望能幫助到你!
對於 Java,只允許不區分大小寫的字母數字和下划線。
public class RegExTest { public static void main(String[] args) { System.out.println("_C#".matches("^[a-zA-Z0-9_]+$")); } }
要檢查整個字符串並且不允許空字符串,請嘗試
^[A-Za-z0-9_]+$
^\\w*$
將適用於以下組合
1
123
1av
pRo
av1
所需格式 允許以下 3 個:
不允許其他格式:
validatePnrAndTicketNumber(){
let alphaNumericRegex=/^[a-zA-Z0-9]*$/;
let numericRegex=/^[0-9]*$/;
let numericdashRegex=/^(([1-9]{3})\-?([0-9]{10}))$/;
this.currBookingRefValue = this.requestForm.controls["bookingReference"].value;
if(this.currBookingRefValue.length == 14 && this.currBookingRefValue.match(numericdashRegex)){
this.requestForm.controls["bookingReference"].setErrors({'pattern': false});
}else if(this.currBookingRefValue.length ==6 && this.currBookingRefValue.match(alphaNumericRegex)){
this.requestForm.controls["bookingReference"].setErrors({'pattern': false});
}else if(this.currBookingRefValue.length ==13 && this.currBookingRefValue.match(numericRegex) ){
this.requestForm.controls["bookingReference"].setErrors({'pattern': false});
}else{
this.requestForm.controls["bookingReference"].setErrors({'pattern': true});
}
}
<input name="booking_reference" type="text" [class.input-not-empty]="bookingRef.value"
class="glyph-input form-control floating-label-input" id="bookings_bookingReference"
value="" maxlength="14" aria-required="true" role="textbox" #bookingRef
formControlName="bookingReference" (focus)="resetMessageField()" (blur)="validatePnrAndTicketNumber()"/>
這對我有用,你可以試試
[\\p{Alnum}_]
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.