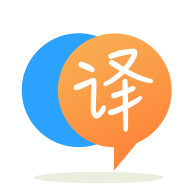
[英]Checking if an ArrayList contains another ArrayList as element
[英]Checking if ArrayList contains anything but one element
檢查arraylist是否包含一個(僅一個)值的最優雅方法是什么?
例如,如何檢查我的arraylist
是否僅包含零:
[0,0,0,0]; [0,0,0,0,0]; [0,0,0,0,0,0,0,0]; <-- All should return true.
我知道有很多方法可以做到這一點,包括非常基本的for-loop
但是是否有使用標准API的優雅的,可能是單行的解決方案?
您可以將元素添加到集合中,並使用類似以下內容的大小進行檢查-
public static void main(String[] args) {
if (new java.util.HashSet<Integer>(
Arrays.asList(new Integer[] { 0, 0, 0, 0 }))
.size() == 1
&& new java.util.HashSet<Integer>(
Arrays.asList(new Integer[] { 0, 0, 0, 0,
0 })).size() == 1
&& new java.util.HashSet<Integer>(
Arrays.asList(new Integer[] { 0, 0, 0, 0,
0, 0, 0, 0 })).size() == 1) {
System.out.println("All true");
} else {
System.out.println("Not true");
}
}
除非您向任何Integer[]
添加非零值,否則它將輸出All true
。
或者,作為通用方法
public static <T> boolean oneValue(java.util.List<T> in) {
// A one-liner.
return (in == null) ? false : new java.util.HashSet<T>(in).size() == 1;
}
的確,這些方法的效率不如簡單地遍歷List
,就像這樣-
public static <T> boolean oneValue(java.util.List<T> in) {
if (in == null)
return false;
T o = in.get(0);
for (int i = 1; i < in.size(); i++)
if (!in.get(i).equals(o))
return false;
return true;
}
我建議以下內容:
public boolean isAllSame(ArrayList<?> list){
Object first=list.get(0);
for(int i=1;i<list.size();i++){
if(!list.get(i).equals(first))return false;
}
return true;
}
(是的,這是一個for循環。但是,如前所述,它並不是那么簡單-在O(n)最差的情況下運行,而O(1)則是最好的情況。並且只有4行。)
將所有數組元素放在單個arraylist中,然后將其放在哈希集中並檢查大小。 如果size為1,則它將返回/打印為true。
Set<Integer> val= new HashSet<Integer>();
List<Integer> allVal= new ArrayList<Integer>();
allVal.addAll(Arrays.asList(new Integer[] { 0, 0, 0, 0 }));
allVal.addAll(Arrays.asList(new Integer[] { 0, 0, 0, 0,0 }));
allVal.addAll(Arrays.asList(new Integer[] { 0, 0, 0, 0,0, 0, 0, 0 }));
val.addAll(allVal);
if(val.size()==1)
System.out.println("All true");
else
System.out.println("More than 1 element present");
您可以嘗試以下方法:
List<Integer> lst = Arrays.asList(new Integer[] { 0, 0, 0, 0 });
int temp = Integer.parseInt(lst.toString().replaceAll(", ", "").replaceAll("\\[", "").replaceAll("]", "").trim());
if(temp == 0) {
System.out.println("All zeros");
}
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.