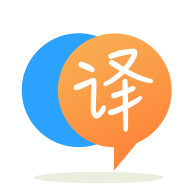
[英]How to bind CheckBox in multipleChoice ListView to boolean value with SimpleCursorAdapter?
[英]how to bind a checkbox to a listview
我有一個列表視圖,在每一行上都包含一個帶有復選框的文本視圖,因此當選中該復選框並且我們在列表視圖中向下滾動時,該復選框實例將從一個地方移到另一個地方(重用..),我還選中了幾個復選框要解決我試圖將復選框綁定到列表視圖但無法正常工作的問題,我的代碼是:
SimpleCursorAdapter adapter =new SimpleCursorAdapter(this,R.layout.rating,cu,new String[]{"Title","Favorites"}, new int[]{R.id.text1,R.id.bt_rating},CursorAdapter.FLAG_REGISTER_CONTENT_OBSERVER);
listv.setAdapter(adapter);
adapter.setViewBinder(new SimpleCursorAdapter.ViewBinder(){
/** Binds the Cursor column defined by the specified index to the specified view */
public boolean setViewValue(View view, Cursor cursor, int columnIndex){
if(view.getId() == R.id.bt_rating){
((CheckBox)view).setChecked(Boolean.valueOf(cursor.getString(cursor.getColumnIndex("Favorites"))));
((CheckBox)view).setOnCheckedChangeListener(myCheckChangList);
return true; //true because the data was bound to the view
}
return false;
}
});
OnCheckedChangeListener myCheckChangList = new OnCheckedChangeListener() {
public void onCheckedChanged(CompoundButton buttonView,
boolean isChecked) {
buttonView.setChecked(isChecked);
}
};
我的listview行內容的xml代碼是:
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="horizontal" >
<CheckBox
android:id="@+id/bt_rating"
android:focusable="false"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_gravity="center_vertical"
android:button="@android:drawable/btn_star"/>
<TextView
android:id="@+id/text1"
android:layout_width="fill_parent"
android:layout_height="wrap_content"
android:textSize="@dimen/fsinlistview"
/>
</LinearLayout>
在任何Listview中,視圖都被重用。 當您滾動瀏覽列表時,那些在屏幕上上下滾動的內容將被回收,並與下面提供的更新信息一起使用。
您需要使用稀疏數組來跟蹤復選框。 用戶觸摸每個數組時,將數組中的索引標記為“ cherked / unchecked”。 然后,您可以根據數組中的值設置復選框的狀態。
這是一個較舊的應用程序的示例代碼,該應用程序既執行“全選”復選框,又管理所有已選中和未選中的列表。 這是我寫的一個課堂出勤應用程序,因此教師選擇在課堂上的“所有”,然后取消選擇那些不存在的應用程序要容易得多。
我在該列表視圖中有兩個復選框,以及兩個稀疏數組,即itemCheckedHere和itemCheckedLate(無論學生是在上課還是遲到)。
public class MyDataAdapter extends SimpleCursorAdapter {
private Cursor c;
private Context context;
private Long classnum;
private gradeBookDbAdapter mDbHelper;
public static final int LATE=2;
public static final int ATTEND=1;
int idxCol;
int idx;
// itemChecked will store the position of the checked items.
public MyDataAdapter(Context context, int layout, Cursor c, String[] from,
int[] to, Long mRowId) {
super(context, layout, c, from, to);
this.c = c;
this.context = context;
mDbHelper = new gradeBookDbAdapter(context);
mDbHelper.open();
classnum = mRowId;
c.moveToFirst();
}
public class ViewHolder{
public TextView text;
public TextView text2;
public ImageView image;
public CheckBox here;
public CheckBox late;
}
public View getView(final int pos, View inView, ViewGroup parent) {
Bitmap bm;
ImageView studentPhoto;
View vi=inView;
final ViewHolder holder;
if (inView == null) {
LayoutInflater inflater = (LayoutInflater) context
.getSystemService(Context.LAYOUT_INFLATER_SERVICE);
vi = inflater.inflate(R.layout.show_attendance, null);
holder=new ViewHolder();
holder.text=(TextView)vi.findViewById(R.id.stuname);
holder.text2=(TextView)vi.findViewById(R.id.stuIndex);
holder.image=(ImageView)vi.findViewById(R.id.icon);
holder.here=(CheckBox)vi.findViewById(R.id.attend);
holder.late=(CheckBox)vi.findViewById(R.id.late);
vi.setTag(holder);
}
else
holder=(ViewHolder)vi.getTag();
c.moveToPosition(pos);
int index = c.getColumnIndex(gradeBookDbAdapter.KEY_NAME);
String name = c.getString(index);
holder.text.setText(name);
index = c.getColumnIndex(gradeBookDbAdapter.KEY_ROWID);
String Index = c.getString(index);
holder.text2.setText(Index);
bm = gradeBookDbAdapter.getStudentPhoto(name);
if (bm != null) {
holder.image.setImageBitmap(bm);
}
else {
// use icon image
holder.image.setImageResource(R.drawable.person_icon);
}
// pull out existing attend/late fields and set accordingly
int attend = c.getInt(c.getColumnIndex(gradeBookDbAdapter.KEY_ATTEND));
if(attend==1){
holder.here.setChecked(true);
itemCheckedHere.set(pos, true);
}
//else {
// holder.here.setChecked(false);
// itemCheckedHere.set(pos, false);
//}
int late = c.getInt(c.getColumnIndex(gradeBookDbAdapter.KEY_LATE));
if (late==1){
holder.late.setChecked(true);
itemCheckedLate.set(pos, true);
}
//else {
// holder.late.setChecked(false);
// itemCheckedLate.set(pos, false);
//}
if (selectAllTouched) {
if(selectAll){
holder.here.setChecked(true);
itemCheckedHere.set(pos, true);
int who= new Integer(holder.text2.getText().toString());
mDbHelper.updateAttend(who, classnum, ATTEND, 1, attendDate );
}
else{
holder.here.setChecked(false);
itemCheckedHere.set(pos, false);
int who = new Integer(holder.text2.getText().toString());
mDbHelper.updateAttend(who, classnum, ATTEND, 0, attendDate );
}
}
holder.here.setOnClickListener(new OnClickListener() {
public void onClick(View v) {
CheckBox cb = (CheckBox) v.findViewById(R.id.attend);
if (cb.isChecked()) {
itemCheckedHere.set(pos, true);
int Index = new Integer(holder.text2.getText().toString());
mDbHelper.updateAttend(Index, classnum, ATTEND, 1, attendDate );
} else if (!cb.isChecked()) {
itemCheckedHere.set(pos, false);
int Index = new Integer(holder.text2.getText().toString());
mDbHelper.updateAttend(Index, classnum, ATTEND, 0, attendDate );
}
}
});
holder.late.setOnClickListener(new OnClickListener() {
public void onClick(View v) {
CheckBox cb = (CheckBox) v.findViewById(R.id.late);
if (cb.isChecked()) {
itemCheckedLate.set(pos, true);
int Index = new Integer(holder.text2.getText().toString());
mDbHelper.updateAttend(Index, classnum, LATE, 1, attendDate );
} else if (!cb.isChecked()) {
itemCheckedLate.set(pos, false);
int Index = new Integer(holder.text2.getText().toString());
mDbHelper.updateAttend(Index, classnum, LATE, 0, attendDate );
}
}
});
holder.here.setChecked(itemCheckedHere.get(pos)); // this will Check or Uncheck the
holder.late.setChecked(itemCheckedLate.get(pos)); // this will Check or Uncheck the
// CheckBox in ListView
// according to their original
// position and CheckBox never
// loss his State when you
// Scroll the List Items.
return vi;
}
}
}
看來您的OnCheckedChangedListener
是這里的問題。 如果您查看代碼,則可以看到每個復選框都引用了同一偵聽器。 因此,當您選中一個框時,也將所有其他框都設置為選中狀態-而且您也不會更新后備數據。
您的OnCheckedChangedListener
不應更新復選框的視圖狀態-觸發回調是因為狀態已經更改。
因此,當用戶選中復選框時,您需要執行以下步驟:
您可以執行以下操作,用其表示的行的ID標記視圖:
public boolean setViewValue(View view, Cursor cursor, int columnIndex){
if(view.getId() == R.id.bt_rating){
view.setTag(cursor.getInt(cursor.getColumnIndex(SomeDBContract.ID)));
((CheckBox)view).setChecked(Boolean.valueOf(cursor.getString(cursor.getColumnIndex("Favorites"))));
((CheckBox)view).setOnCheckedChangeListener(myCheckChangList);
return true; //true because the data was bound to the view
}
return false;
}
然后,您可以在偵聽器中根據該ID更新數據庫:
CheckedChangeListener myCheckChangList = new OnCheckedChangeListener() {
public void onCheckedChanged(CompoundButton buttonView,
boolean isChecked) {
int rowId = (int) buttonView.getTag();
// Handle updating the database as per normal
updateSomeDbRowAsChecked(rowId, isChecked);
}
};
最后,一旦數據庫行更新,您將需要使用新的游標更新游標適配器:
myAdapter.swapCursor(newCursor);
您必須對所有這些進行調整以適合您的代碼,但這應該使您對解決此問題的一種方式有所了解。
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.