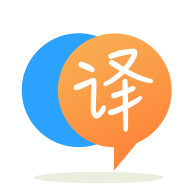
[英]Java Error java.lang.StringIndexOutOfBoundsException: String index out of range: 0
[英]“Exception:java.lang.StringIndexOutOfBoundsException: String index out of range”
這是問題所在:如果字符串“ cat”和“ dog”在給定的字符串中出現相同的次數,則返回true。 例如:catDog(“ catdog”)→true; catDog(“ catcat”)→false; catDog(“ 1cat1cadodog”)→true
public boolean catDog(String str) {
int countCat=0;
int countDog=0;
for (int i=0; i<str.length();i++)
{
if (str.charAt(i)== 'c'&& str.length()>=3)
{
if (str.substring(i,i+3).equals("cat"))
countCat++;
}
}
for (int i=0; i<str.length();i++)
{
if (str.charAt(i)== 'd' && str.length()>=3)
{
if (str.substring(i,i+3).equals("dog"))
countDog++;
}
}
if (countCat == countDog)
return true;
else
return false;
}
str.substring(i,i+3).equals("cat")
i
可能是最后一個,而i+3
會給出錯誤
在for
循環條件中,您正在檢查整個String的長度是否大於或等於3
而不是僅檢查從i
到結尾的部分。 嘗試一下
str.length() - i >= 3
代替
str.length() >= 3
為什么不簡單地使用StringUtils#countMatches
?
StringUtils.countMatches(myStr, "cat") == StringUtils.countMatches(myStr, "dog");
不要迷失在索引上。 但是,如果您不想使用此方法,則調試代碼是您可以做的最好的事情。
好的,這就是我可能會做的:
問題出在您的檢查str.length() >= 3
。 應該是i + str.length()
。
我還建議對代碼進行一些更改以消除重復。 在這里,我提取了計算子字符串出現次數的部分,並將其移至其自己的方法。 現在檢查貓數是否等於狗數的部分兩次調用了上述方法。
public static void main(String[] args) {
System.out.println(catDog("catdog"));
System.out.println(catDog("catcat"));
System.out.println(catDog("1cat1cadodog"));
System.out.println(catDog("catdogcatc"));//Would previously throw error.
}
public static boolean catDog(String str) {
int countCat = countAppearances(str, "cat");
int countDog = countAppearances(str, "dog");
return countCat == countDog;
}
private static int countAppearances(String str, String key) {
int count = 0;
for (int i = 0; i < str.length(); i++) {
if (str.charAt(i) == key.charAt(0) && i + key.length() <= str.length()) {
if (str.substring(i, i + key.length()).equals(key)) {
count++;
}
}
}
return count;
}
您需要先更新第一個條件,然后才能像下面這樣吐出字符串:
if (str.charAt(i)== 'c' && (str.length() - i) >= 3)
{
if (str.substring(i,i+3).equals("cat"))
countCat++;
}
public boolean catDog(String str) {
int catCount = 0, dogCount = 0;
//run a for loop to check cat count
//run loop till 2nd last character
for (int i = 0; i < str.length() - 2; i++) {
//now check if the charaters at positions matches "cat"
//if matches then increment cat count
if (str.charAt(i) == 'c' && str.charAt(i + 1) == 'a' && str.charAt(i + 2) == 't') {
catCount++;
} else if (str.charAt(i) == 'd' && str.charAt(i + 1) == 'o' && str.charAt(i + 2) == 'g') {
//else check if the charaters at positions matches "dog"
//if matches then increment dog count
dogCount++;
}
}
//check cat count and dog count
if (catCount == dogCount) {
return true;
} else {
return false;
}
}
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.