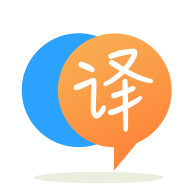
[英]Should a std::vector of objects use pointers, references, or nothing?
[英]How to store pointers (or references) to objects in a std::set
C++11 STL 中是否有任何適當的方法將對象指針存儲在std::set
,並通過對象的operator <
方法對它們進行正確排序?
當然,可以編寫我自己的Compare
類型並將其作為第二個模板參數傳遞給set
,但我認為 STL 會提供更方便的方法。
一些谷歌搜索顯示std::reference_wrapper
,在我看來應該允許這樣的代碼:
#include <functional>
#include <set>
struct T {
int val;
bool operator <(T& other) {
return (this->val < other.val);
}
};
int main() {
std::set<std::reference_wrapper<T>> s;
T a{5};
s.insert(a);
}
但實際上,這會導致編譯器錯誤:
clang++ -std=c++11 -Wall -Wextra -pedantic test.cpp -o test
In file included from test.cpp:1:
In file included from /usr/bin/../lib64/gcc/x86_64-unknown-linux-gnu/4.8.2/../../../../include/c++/4.8.2/functional:49:
/usr/bin/../lib64/gcc/x86_64-unknown-linux-gnu/4.8.2/../../../../include/c++/4.8.2/bits/stl_function.h:235:20: error: invalid operands to binary expression ('const std::reference_wrapper<T>'
and 'const std::reference_wrapper<T>')
{ return __x < __y; }
~~~ ^ ~~~
(gcc 錯誤類似,但要長得多)
您需要使小於運算符成為非成員,並為其提供const
引用參數:
struct T {
int val;
};
bool operator <(const T& lhs, const T& rhs) {
return (lhs.val < rhs.val);
}
這允許在<
運算符的 LHS 和 RHS 上從std::reference_wrapper<T>
到T
的隱式轉換,而成員版本只允許在 RHS 上進行隱式轉換。 二元運算符的 LHS 和 RHS 之間的對稱性是將它們實現為非成員的經典論據之一。
方法調用的對象不能隱式轉換,因此需要將比較實現為自由函數,才能使用從reference_wrapper<T> 到T 的轉換。
正確的方法是為我們的 MyStruct 創建一個專門的std::less
。
namespace std
{
template<> struct less<MyStruct>
{
bool operator() (const MyStruct& lhs, const MyStruct& rhs) const
{
return lhs.a < rhs.a;
}
};
}
記住std::set
默認使用std::less
來比較兩個元素。
在標題<set>
定義
template<
class Key,
class Compare = std::less<Key>,
class Allocator = std::allocator<Key>
> class set;
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.