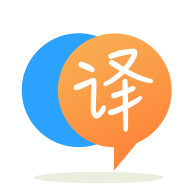
[英]Why is the repaint() method not calling my paintComponent() method?
[英]Why is repaint() not reaching the paintComponent() method?
我一直在學校為AP Java做一個旋轉線項目。 所有的支持類都是完全完成的(由我的合作伙伴完成),但由於我的paintComponent()
方法完全不起作用,我還沒有完成我的顯示。
我已經尋求幫助,但還沒有人能夠找到錯誤。 我所需要的只是讓這些多邊形在屏幕上繪畫,我可以完成剩下的工作。
這是我到目前為止:
imports...
public class Display extends JFrame {
protected int screenHeightD = 600;
protected int screenWidthD = 700;
private ArrayList <Rotator> planesOnBoard = new ArrayList ();
private Timer timer;
private boolean timerOn = true;
private int timerDuration = 300;
private int rotateDegValue = 5;
PlaneFacilitator painter = new PlaneFacilitator();
JFrame display = new JFrame ("Rotating Line");
public class PlaneFacilitator extends JComponent {
// takes the double arrays sent by the getXArray/getYArray
public int [] convertXcrds ( double [] xCrdsD ) {
int [] xCrds = new int[xCrdsD.length];
for ( int i = 0 ; i < xCrdsD.length - 1; i++) {
double e = xCrdsD [i];
xCrds [i] = (int) Math.round (e);
}
return xCrds;
}
public int [] convertYcrds ( double [] yCrdsD ) {
int [] yCrds = new int[yCrdsD.length];
for ( int i = 0 ; i < yCrdsD.length - 1; i++) {
double e = yCrdsD [i];
yCrds [i] = (int) Math.round (e);
}
return yCrds;
}
// paints the entire set of planes
public void paintComponent (Graphics g){
System.out.println("Painted");
planesOnBoard.add(0, new Rotator (screenWidthD / 2, screenHeightD / 2, new Dimension (screenHeightD, screenWidthD)));
planesOnBoard.get(0).addPolygon(Color.black, 1, 0, true);
for ( Rotator target : planesOnBoard) {
for ( int polyIndex = 0; polyIndex < target.getNumPolygons(); polyIndex++ ) {
double[] xCrdsD = target.getXArray(polyIndex);
double[] yCrdsD = target.getYArray(polyIndex);
g.setColor(target.getColor(polyIndex));
g.drawPolyline(convertXcrds(xCrdsD), convertYcrds(yCrdsD), xCrdsD.length);
}
g.setColor(Color.green);
g.drawRect(target.getXOrigin() - 2, target.getYOrigin() - 2, 5, 5);
}
}
}
// ctor for display, creates the frame...
public Display () {
display.setLayout(null);
display.setSize(screenWidthD, screenHeightD);
display.setTitle("Rotating Line");
display.add(painter);
System.out.println(screenWidthD + " " + screenHeightD);
JButton startB = new JButton ("Start");
JButton pauseB = new JButton ("Pause");
ActionListener pauseBL = new pauseB();
pauseB.addActionListener(pauseBL);
ActionListener startBL = new startB();
startB.addActionListener(startBL);
startB.setBounds(10, 10, 150, 20);
pauseB.setBounds(170, 10, 150, 20);
display.add(startB);
display.add(pauseB);
display.addMouseListener(new DropDownMenuListener());
display.getContentPane().setBackground(Color.WHITE);
display.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
display.setVisible(true);
display.setBounds(0, 0, screenWidthD, screenHeightD);
display.setResizable(false);
display.getContentPane().repaint();
}
// creates a dropdown menu that appears whenever you click on the screen.
class DropDownMenu extends JPopupMenu {
public DropDownMenu(){
JMenuItem addPent = new JMenuItem ("Add a Pentagon");
JMenuItem addQuad = new JMenuItem ("Add a Quadrilateral");
JMenuItem addTri = new JMenuItem ("Add a Triangle");
JMenuItem addLine = new JMenuItem ("Add a Line");
JMenuItem reverseRot = new JMenuItem ("Reverse the Rotation of the plane");
JMenuItem addNewPlane = new JMenuItem ("Add a new plane here");
add(addPent);
add(addQuad);
add(addTri);
add(addLine);
add(reverseRot);
add(addNewPlane);
addNewPlane.addActionListener(new addPlaneListener());
addLine.addActionListener(new addLineListener());
addTri.addActionListener(new addTriListener());
addQuad.addActionListener(new addQuadListener());
addPent.addActionListener(new addPentListener());
reverseRot.addActionListener(new addReverseRotateListener());
}
}
// Lots an lots of listeners...
int xCrdMenu;
int yCrdMenu;
class DropDownMenuListener extends MouseAdapter {
public void mousePressed(MouseEvent e){
if (e.isPopupTrigger())
xCrdMenu = e.getX();
yCrdMenu = e.getY();
doPop(e);
}
public void mouseReleased(MouseEvent e){
if (e.isPopupTrigger())
doPop(e);
}
private void doPop(MouseEvent e){
DropDownMenu menu = new DropDownMenu();
menu.show(e.getComponent(), e.getX(), e.getY());
}
}
// defines the behavior of the startB
// starts the timer...rotates x degrees each time
class startB implements ActionListener {
public void actionPerformed(ActionEvent e) {
timer = new Timer();
timerOn = true;
(timer).scheduleAtFixedRate(new TimerTask()
{
public void run() {
System.out.println("Time");
for ( Rotator target : planesOnBoard) {
for ( int polyIndex = 0; polyIndex < target.getNumPolygons(); polyIndex++ ) {
target.rotate ( rotateDegValue, polyIndex );
}
} painter.repaint();
}
}
, (long) timerDuration, (long) timerDuration);
}
}
// defines the behavior for the pause button
class pauseB implements ActionListener {
public void actionPerformed(ActionEvent e) {
if(timerOn == true){
System.out.println("Pause");
timer.cancel();;
timerOn = false;
}
}
}
class addPlaneListener implements ActionListener {
public void actionPerformed(ActionEvent e) {
//JFrame source = (JFrame) e.getSource();
Plane added = new Plane (getContentPane().getY(), getContentPane().getX(),getContentPane().getY(),getContentPane().getX());
planesOnBoard.add(new Rotator (added));
added.setOrigin(xCrdMenu, yCrdMenu);
painter.repaint();
System.out.println("Plane");
}
}
class addLineListener implements ActionListener {
public void actionPerformed(ActionEvent e) {
planesOnBoard.get(0).addPolygon( new Polygon (Color.black, 1, 180, true, getContentPane().getY(), getContentPane().getX(),getContentPane().getY(),getContentPane().getX()));
painter.repaint();
System.out.println("Line");
}
}
class addTriListener implements ActionListener {
public void actionPerformed(ActionEvent e) {
planesOnBoard.get(0).addPolygon(Color.black, 3, 60, true);
painter.repaint();
}
}
class addQuadListener implements ActionListener {
public void actionPerformed(ActionEvent e) {
planesOnBoard.get(0).addPolygon(Color.black, 4, 90, true);
painter.repaint();
}
}
class addPentListener implements ActionListener {
public void actionPerformed(ActionEvent e) {
planesOnBoard.get(0).addPolygon(Color.black, 5, 108, true);
painter.repaint();
}
}
class addReverseRotateListener implements ActionListener {
public void actionPerformed(ActionEvent e) {
rotateDegValue *= -1;
}
}
public static void main ( String [] args ) {
Display experiment = new Display();
}
}
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.