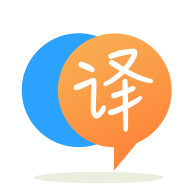
[英]How to Convert array of CSV formatted Strings into an array of JSON objects
[英]How to create an array of objects that have strings from a CSV file of strings?
我有一些代碼從一個csv文件讀取,該文件充滿了很多人的姓氏,名字和出生年份。 在csv文件中看起來像這樣:
nameLast nameFirst birthYear
Santos Valerio 1972
Tekulve Kent 1947
Valentine Fred 1935
我有一個叫做人的類,人的類也有這三個值。 我想創建一個人類類的數組,並將來自csv文件的所有數據傳遞給它。 這樣,如果數組的第一個實例在[0]處將具有三個值,則兩個代表名稱,一個代表年份。 這是我到目前為止的代碼:
package testing.csv.files;
import java.io.File;
import java.io.FileNotFoundException;
import java.util.Scanner;
public class Human {
String lastName;
String firstName;
String birthYear;
public static void main(String[] args) {
//.csv comma separated values
String fileName = "C:/Users/Owner/Desktop/Data.csv";
File file = new File(fileName); // TODO: read about File Names
try {
Scanner inputStream = new Scanner(file);
inputStream.next(); //Ignore first line of titles
while (inputStream.hasNext()){
String data = inputStream.next(); // gets a whole line
String[] values = data.split(",");
System.out.println(values[2]);
}
inputStream.close();
} catch (FileNotFoundException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
}
}
由於值[2]是出生年份,因此我現在可以打印出所有的出生年份。 我以為我可以改變路線
String[] values = data.split(",");
讀書
Human[] values = data.split(",");
然后,它將自動將值lastName,firstName和birthyear分配給數組中每個對象的正確位置。 但是,這樣做只會產生錯誤消息
incompatible types: String[] cannot be converted to Human[]
我試過也改變了這一行從
String data = inputStream.next(); // gets a whole line
至
Human data = inputStream.next(); // gets a whole line
但我收到相同的錯誤消息。
那我在做什么錯? 也許我沒有像我以前認為的那樣正確地定義類,或者我的方法有更多錯誤嗎? 請讓我知道你的想法。
在String類中split
的方法返回String數組,而不是Human
數組。 要創建Human
數組,您需要將從split
返回的String數組解析為人類需要的值。
class Human{
String firstName;
String lastName;
int birthYear;
public Human(String first, String last, int birthYear){
this.firstName = first;
this.lastName = last;
this.birthYear = birthYear;
}
}
int numHumansInCSV = //How many humans are in your file?
Human[] humanArray = new Human[numHumansInCSV];
int index = 0;
while (inputStream.hasNext()){
String data = inputStream.next(); // gets a whole line
String[] values = data.split(",");
String lastName = values[0];
String firstName = values[1];
int birthYear = Integer.parseInt(values[2]);
Human human = new Human(firstName, lastName, birthYear);
//put human in human array.
humanArray[index] = human;
index += 1;
}
如果您可以使用List
這樣的數據結構,那將比數組更合適,因為您可能不知道CSV文件包含多少個人。
而且,此解析代碼實際上應該在其自己的類中。 如果我正在寫這篇文章,這就是我要做的。
public class HumanCSVReader{
public List<Human> read(String csvFile, boolean expectHeader) throws IOException{
File file = new File(csvFile);
Scanner scanner = new Scanner(file);
int lineNumber = 0;
List<Human> humans = new List<Human>();
while(scanner.hasNextLine()){
if(expectHeader && lineNumber==0){
++lineNumber;
continue;
}
String line = scanner.nextLine();
String csv = line.split(",");
if(csv.length!=3)
throw new IOException("Bad input in "+csvFile+", line "+lineNumber);
String firstName = list[0];
String lastName = list[1];
try{
int birthYear = Integer.parseInt(list[2]);
}catch(NumberFormatException e){
throw new IOException("Bad birth year in "+csvFile+", line "+lineNumber);
}
Human human = new Human(fistName, lastName, birthYear);
humans.add(human);
++lineNumber;
}
return humans;
}
}
只需使用它來加載您的人類數組即可。 它甚至會處理一些基本的驗證。
首先,您的Human類需要一個構造函數。 插入以下方法。
public Human(String[] str) {
lastName = str[0];
firstName = str[1];
birthYear = str[2];
}
現在,您可以使用以下方法來獲取Human對象,而不是字符串數組:
Human someHuman = new Human(data.split(","));
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.