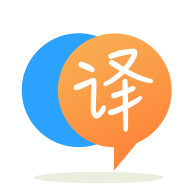
[英]How to convert a date a user selects in MM-dd-yyyy format to the format yyyy-MM-dd in Java
[英]Java - Convert String variable to Date + get today's date in format MM/dd/yyyy
快速背景:
我在Eclipse中為預訂應用程序創建了一個GUI。 在它上面,我有多個組合框用於到達和離開日期(月,日,年 - 兩者)。 我檢索了信息並將其綁定到String變量,因此它看起來像這樣:12/04/2014。
問題:
我一直在互聯網上搜索了一段時間,但似乎找不到任何清楚的東西。
請不要推薦任何附加組件或界面工具,因為我必須手動編寫所有這些內容,只提供現成的內容(如果有意義的話)。
謝謝!
---編輯---
我嘗試了下面提到的很多東西......
'今天的日期=新的日期()'在新的日期()下給我一條紅線,要求參數為int或long。
下面是我從組合框中匯總日期時的代碼。 dateFormat.parse(holder)獲得一個紅色下划線,提示使用try / catch添加throws聲明或環繞聲。 以下變量的數據類型是... index(int)。 holder(String)。 到達/離開(java.util.Date) - 無論出於何種原因,即使使用導入日期也不會接受日期,盡管它在我的酒店類中。 奇怪,是的,但不是我的主要焦點。
// Assignments index = view.getArriveMonthIndex() + 1; // Arrival date holder = index + "/" + view.getArriveDay() + "/" + view.getArriveYear(); arrival = dateFormat.parse(holder); index = view.getDepartMonthIndex() + 1; // Departure date holder = index + "/" + view.getDepartDay() + "/" + view.getDepartYear(); departure = dateFormat.parse(holder);
還有其他的東西可能會提及/顯示..在我課程的最開始,這是我一直在玩/嘗試的一些東西。
DateFormat dateFormat = new SimpleDateFormat("MM/dd/yyyy", Locale.US);
Calendar todaysDate = Calendar.getInstance();
String today = dateFormat.format(todaysDate.getTime());
^^剛剛測試了最后一部分,它可以工作。
我繼續為我的到達/離開變量加下划線的建議。 我的方法現在拋出ParseException。 還沒有找到一個好的描述 - 這究竟是什么意思? (非常新的,如果你不能告訴) - 備份。 將其改回原來的狀態。 這種方式需要在我調用reserveHotel()方法時拋出/捕獲。
您應該使用原始Date
對象作為UI組件中模型的數據,並使用渲染器顯示數據的值,最好是尊重用戶的區域設置。
這樣,您就不必在數據類型之間進行轉換
仔細查看如何使用組合框了解更多詳細信息
例如...
import java.awt.BorderLayout;
import java.awt.Component;
import java.awt.EventQueue;
import java.awt.GridBagLayout;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import java.text.DateFormat;
import java.util.Calendar;
import java.util.Date;
import javax.swing.DefaultComboBoxModel;
import javax.swing.DefaultListCellRenderer;
import javax.swing.JComboBox;
import javax.swing.JFrame;
import javax.swing.JList;
import javax.swing.UIManager;
import javax.swing.UnsupportedLookAndFeelException;
public class TestCombos {
public static void main(String[] args) {
new TestCombos();
}
public TestCombos() {
EventQueue.invokeLater(new Runnable() {
@Override
public void run() {
try {
UIManager.setLookAndFeel(UIManager.getSystemLookAndFeelClassName());
} catch (ClassNotFoundException | InstantiationException | IllegalAccessException | UnsupportedLookAndFeelException ex) {
}
DefaultComboBoxModel model = new DefaultComboBoxModel();
Calendar cal = Calendar.getInstance();
for (int index = 0; index < 100; index++) {
model.addElement(cal.getTime());
cal.add(Calendar.DATE, 1);
}
JComboBox comboBox = new JComboBox(model);
comboBox.setRenderer(new DefaultListCellRenderer(){
@Override
public Component getListCellRendererComponent(JList<?> list, Object value, int index, boolean isSelected, boolean cellHasFocus) {
if (value instanceof Date) {
value = DateFormat.getDateInstance(DateFormat.SHORT).format((Date)value);
}
return super.getListCellRendererComponent(list, value, index, isSelected, cellHasFocus);
}
});
comboBox.addActionListener(new ActionListener() {
@Override
public void actionPerformed(ActionEvent e) {
Object value = ((JComboBox)e.getSource()).getSelectedItem();
if (value instanceof Date) {
System.out.println("You have selected a Date value of " + value);
} else {
System.out.println("You selected something else");
}
}
});
JFrame frame = new JFrame("Testing");
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
frame.setLayout(new GridBagLayout());
frame.add(comboBox);
frame.pack();
frame.setLocationRelativeTo(null);
frame.setVisible(true);
}
});
}
}
今天的日期就像new Date()
一樣簡單
嘗試使用SimpleDateFormat類指定日期掩碼(“MM / dd / yyyy”)並將字符串轉換/解析為Date
。
然后與創建為Date dt = new Date ();
的Date
進行比較Date dt = new Date ();
見http://docs.oracle.com/javase/6/docs/api/java/util/Date.html#after(java.util.Date)
您可以使用以下內容將字符串解析為日期:
Date date = new SimpleDateFormat("MMMM d, yyyy", Locale.ENGLISH).parse(YOURDATE);
您可以使用以下內容獲取今天的日期:
Date date = new Date();
像這樣使用java SimpleDateFormat :
SimpleDateFormat simpleDateFormat = new SimpleDateFormat("MM/dd/yyyy");
String dateString = "12/04/2014";
Date myDate = simpleDateFormat.parse(dateString);
今天的日期可以通過以下方式獲得:
Date today = new Date();
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.