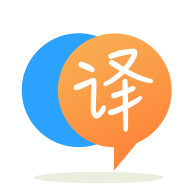
[英]Need to find the character most frequent in a string and return the number of times it appears
[英]Java program to find the character that appears the most number of times in a String?
所以這是到目前為止我得到的代碼...
import java.util.Scanner;
class count{
public static void main(String args[]){
Scanner s=new Scanner(System.in);
System.out.println("Enter a string");
String sent=s.nextLine();
int len = sent.length();
int arr[]=new int[len];
int count=1;
char ch[] = new char[len];
for(int i = 0; i <= len-1; i ++)
{
ch[i] = sent.charAt(i);
}
for(int j= 0;j<=len-1;j++){
for(int k=0;k<=len-1;k++){
if(ch[j]==ch[k]){
arr[j]= count++;
}
}
}
int max=arr[0];
for(int z=1;z<=len-1;z++){
if(count>max)
max=count;
}
System.out.println(max);
System.out.println("The character that appears the most number of times is " +arr[max]);
}
}
我得到計數以顯示每個字符出現在字符串中的次數,但我無法將其與數組中的其余元素進行比較。
出現的次數存儲在數組'arr []'中,如何找到該數組中的最大整數? 而且,如何顯示出現次數最多的字符?
此后,代碼的邏輯不起作用,
int max=arr[0];
關於做什么的任何想法?
Collections
。 簡單容易。 HashMap <Character,Integer>
。 String
。 對於字符串中的每個字符,檢查它是否在映射中可用(作為鍵)。 如果是,則增加計數,否則將字符添加到地圖中作為鍵並將計數設置為0。 String sent = "asdAdFfaedfawghke4";//s.nextLine();
int length = sent.length();
char frequentChar = ' ';
int maxLength = 0;
for (int i = 0; i < length; i++) {
char currentChar = sent.charAt(0);
sent = sent.replaceAll(currentChar + "", "");//remove all charactes from sent
if (maxLength < (length - sent.length())) {
frequentChar=currentChar;
maxLength = length - sent.length();
}
System.out.println("Char : " + currentChar + " Occurance " + (length - sent.length()));
length = sent.length();
}
System.out.println("Max Occurance : " + maxLength);
輸出:
Char : a Occurance 3
Char : s Occurance 1
Char : d Occurance 3
Char : A Occurance 1
Char : F Occurance 1
Char : f Occurance 2
Char : e Occurance 2
Frequent Char : a
Max Occurance : 3
提示:在構建HashMap的同時,您可以存儲最大值和相應的字符。 仍然是O(n),但是代碼會更簡潔。
首先初始化arr []即
for(int i=0;i<len-1;i++{
arr[i]=0;
}
然后 :
for(int j= 0;j<=len-1;j++){
count=0;
for(int k=0;k<=len-1;k++){
if(ch[j]==ch[k]){
arr[j]= count++;
}
}
}
您可以使用以下邏輯來獲取出現的最大字符數。 下面的程序將整個字符串轉換為大寫字母,之后將計算出現的最大字符數,並在upperCase中打印字符。
import java.util.Scanner;
class Mostchar{
public static void main(String args[]){
Scanner s=new Scanner(System.in);
System.out.println("Enter a string");
String sent=s.nextLine();
sent = sent.toUpperCase();
System.out.println(sent);
int len = sent.length();
int arr[]=new int[26];
int max=0;
char ch=0;
for(int i=0;i<len;i++)
{
arr[sent.charAt(i)-65] = arr[sent.charAt(i)-65] + 1;
if(arr[sent.charAt(i)-65]>max)
{
max = arr[sent.charAt(i)-65];
ch=sent.charAt(i);
}
}
System.out.println("Max occured char is: "+ch+" , times: "+max);
}
}
我會做這樣的事情
String str = "yabbadabbadoo";
Hashtable<Integer, Integer> ht = new Hashtable<Integer, Integer>() ;
for (int x = 0; x < str.length(); x++) {
Integer idx = (int) str.charAt(x);
Integer got = ht.get(idx);
if (got == null) {
ht.put(idx, 1);
} else {
ht.put(idx, got.intValue() + 1);
}
}
int max = 0;
char ch = '-';
Enumeration<Integer> keys = ht.keys();
while (keys.hasMoreElements()) {
Integer idx = keys.nextElement();
int count = ht.get(idx);
if (count > max) {
ch = (char) idx.intValue();
max = count;
}
}
System.out.print("[" + ch + "]");
System.out.println(" Max " + max);
注意大寫和小寫字符
每次k迭代后,變量count都需要重置該值,因此它看起來像這樣
for(int j= 0;j<=len-1;j++){
for(int k=0;k<=len-1;k++){
if(ch[j]==ch[k]){
arr[j]= count++;
}
}count=1;
}
此外,當您嘗試在數組中查找最大值時,這樣做會更容易且更正確。
int max=0;
for(int z=1;z<=len-1;z++){
if(arr[z]>arr[max])
max=z;
}
您正在執行的操作(而不是重置計數后的操作)將存儲第一個字符的出現計數,然后將其與否進行比較。 最后一個字符出現的次數,如果還有更多,則最后一個字符本身出現。
假設您有以下示例:
BACAVXARB
那么您的第一個數組ch的輸出將是:
ch[0]=B
ch[1]=A
ch[2]=C
ch[3]=A
ch[4]=V
ch[5]=X
ch[6]=A
ch[7]=R
ch[8]=B
那么下一個輸出將是:
arr[0]=2
arr[1]=3
arr[2]=1
arr[3]=3
arr[4]=1
arr[5]=1
arr[6]=3
arr[7]=1
arr[8]=2
但是該輸出將是錯誤的,因為計數器不會重置,因此我們這樣做:
for(int j= 0;j<=len-1;j++){
for(int k=0;k<=len-1;k++){
if(ch[j]==ch[k]){
arr[j]= count++;
}
}
count=1;
}
然后,您的最大變量將是:
max=2
因此,在您的最后一個問題上,我使用自己的變量做了這個:
int newCounter;
for(i=0; i<= len-1; i++){
if(arr[i]>max){
max = arr[i];
newCounter = i; //We create this new variable, to save the position, so we will always have the same counter on arr[i] and ch[i]
}
}
所有缺少的部分是:
System.out.println("The character that repeats the most is: " + ch[newCounter]);
輸出應該是:重復次數最多的字符是:A
希望這可以幫助
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.