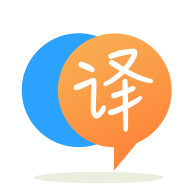
[英]How to combine paintComponent(Graphics g) with MouseListener and MouseMotionListener
[英]How to draw with MouseListener and MouseMotionListener
我正在創建一個程序,您可以使用MouseListener和MouseMotionListener在JPanel中繪制矩形,但是當我運行該程序時,出現了nullPointer異常,該異常是由於未輸入任何參數來繪制矩形而引起的。 問題在於程序如何不允許用戶在屏幕上繪制矩形以提供矩形的參數。
截至目前,我僅嘗試繪制1個矩形,但最后程序將需要繪制多個矩形,因此在這方面的任何幫助都將非常有用。
這是代碼:
public class RectangleFrame extends JFrame implements ActionListener {
JPanel buttonPanel;
JButton saveImage;
JButton clearImage;
JCheckBox intersections;
JCheckBox union;
JPanel drawingArea;
public RectangleFrame()
{
super();
setTitle("Rectangles");
setSize(600,600);
setResizable(false);
this.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
buttonPanel = new JPanel();
buttonPanel.setBorder(BorderFactory.createLineBorder(Color.black));
this.add(buttonPanel, BorderLayout.SOUTH);
intersections = new JCheckBox("Draw Intersections");
buttonPanel.add(intersections);
union = new JCheckBox("Draw Union");
buttonPanel.add(union);
saveImage = new JButton("Save Image");
saveImage.setMargin(new Insets(0,0,0,0));
buttonPanel.add(saveImage);
clearImage = new JButton("Clear Image");
clearImage.setMargin(new Insets(0,0,0,0));
buttonPanel.add(clearImage);
drawingArea = new RectanglePanel();
drawingArea.setBorder(BorderFactory.createLineBorder(Color.blue));
this.add(drawingArea, BorderLayout.CENTER);
drawingArea.setVisible(true);
drawingArea.addMouseListener((MouseListener) drawingArea);
drawingArea.addMouseMotionListener((MouseMotionListener) drawingArea);
}
@Override
public void actionPerformed(ActionEvent arg0)
{
// TODO Auto-generated method stub
}
}
class RectanglePanel extends JPanel implements MouseListener, MouseMotionListener {
Rectangle rectangle;
int x2, y2;
public RectanglePanel()
{
super();
}
@Override
public void mouseDragged(MouseEvent arg0)
{
// TODO Auto-generated method stub
}
@Override
public void mouseMoved(MouseEvent arg0)
{
// TODO Auto-generated method stub
}
@Override
public void mouseClicked(MouseEvent arg0)
{
// TODO Auto-generated method stub
}
@Override
public void mouseEntered(MouseEvent arg0)
{
// TODO Auto-generated method stub
}
@Override
public void mouseExited(MouseEvent arg0)
{
// TODO Auto-generated method stub
}
@Override
public void mousePressed(MouseEvent arg0)
{
rectangle.setX(arg0.getX());
rectangle.setY(arg0.getY());
repaint();
}
@Override
public void mouseReleased(MouseEvent arg0)
{
x2 = arg0.getX();
y2 = arg0.getY();
rectangle.setWidth(Math.abs(rectangle.getX() - x2));
rectangle.setHeight(Math.abs(rectangle.getY() - y2));
repaint();
}
@Override
public void paintComponent(Graphics g)
{
super.paintComponent(g);
g.setColor(Color.BLUE);
g.fillRect(rectangle.getX(), rectangle.getY(), rectangle.getWidth(), rectangle.getHeight());
}
}
您需要在mousePressed上創建一個新的Rectangle對象,然后將其分配給矩形變量。 然后,您可以在mouseDragged方法中更改其狀態。
或更妙的是,使用在鼠標事件上設置的Point對象:
即
// variable declarations
Point initialPoint = null;
Rectangle rectangle = null;
@Override
public void mousePressed(MouseEvent mEvt) {
initialPoint = mEvt.getPoint();
rectangle = null;
repaint();
}
mouseDragged(MouseEvent mEvt) {
// use initialPoint, mEvt.getPoint(),
// Math.abs(...), Math.min(...), and Math.max(...)
// to calculate x, y, w, and h
rectangle = new Rectangle(x, y, w, h);
repaint();
}
同樣在paintComponent中,如果不為null,則僅繪制矩形。
@Override
public void paintComponent(Graphics g) {
super.paintComponent(g);
if (rectangle != null) {
Graphics2D g2 = (Graphics2D) g;
g2.setColor(Color.BLUE);
g2.fill(rectangle);
}
}
至於,
截至目前,我僅嘗試繪制1個矩形,但最后程序將需要繪制多個矩形,因此在這方面的任何幫助都將非常有用。
這很容易。 創建一個ArrayList<Rectangle>
,然后在mouseReleased上將創建的Rectangle對象放入List中。 在paintComponent方法中,使用for循環遍歷列表,繪制其中包含的每個Rectangle。
查看“ 自定義繪畫方法”中的兩個解決方案,這些解決方案使您可以繪制矩形:
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.