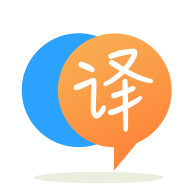
[英]Incompatible pointer types assigning to 'NSString *' from 'NSMutableArray *'
[英]Incompatible pointer types assigning to “UITextField” from NSString
我試圖在productdetailview對象中獲取標簽的值,並將其寫在位於edit product對象上的文本字段中。 有人可以讓我知道問題是什么嗎?
謝謝。
編輯:下面添加了更多代碼以解決標簽到文本字段的問題。
產品.h
#import <Foundation/Foundation.h>
@interface Product : NSObject
@property (strong, nonatomic) NSString *name;
@property (strong, nonatomic) NSString *description;
@property (strong, nonatomic) NSString *product_id;
@property (strong, nonatomic) NSString *model;
@property (strong, nonatomic) NSString *sku;
@property (strong, nonatomic) NSString *quantity;
@property (strong, nonatomic) NSURL *imageUrl;
@property (strong, nonatomic) NSString *price;
@property (strong, nonatomic) NSString *weight;
@property (strong, nonatomic) NSString *length;
@property (strong, nonatomic) NSString *width;
@property (strong, nonatomic) NSString *height;
@property (strong, nonatomic) NSString *status;
@property (strong, nonatomic) NSString *date_added;
@property (strong, nonatomic) NSString *viewed;
#pragma mark -
#pragma mark Class Methods
-(id) initWithName: (NSString*)pName andProductDescription: (NSString *)pDesc andProductId: (NSString *)pId andModelNumber: (NSString*)mNumber andStoreKeepingUnit:(NSString*)storeKu andProductQuantity:(NSString*)pQuantity andProductImage:(NSURL*)pImage andProductPrice:(NSString*)pPrice andWeight:(NSString*)pWeight andLength:(NSString*)pLength andWidth:(NSString*)pWidth andHeight:(NSString*)pHeight andProductStatus:(NSString*)pStatus andDateAdded:(NSString*)pDate andProductViewed:(NSString*)pViewed;
@end
產品
#import "Product.h"
@implementation Product
@synthesize product_id, date_added, height, weight, width, length, sku, status, imageUrl, model, price, quantity, viewed, name, description;
-(id) initWithName: (NSString*)pName andProductDescription: (NSString *)pDesc andProductId: (NSString *)pId andModelNumber: (NSString*)mNumber andStoreKeepingUnit:(NSString*)storeKu andProductQuantity:(NSString*)pQuantity andProductImage:(NSURL*)pImage andProductPrice:(NSString*)pPrice andWeight:(NSString*)pWeight andLength:(NSString*)pLength andWidth:(NSString*)pWidth andHeight:(NSString*)pHeight andProductStatus:(NSString*)pStatus andDateAdded:(NSString*)pDate andProductViewed:(NSString*)pViewed
{
self = [super init];
if (self)
{
name=pName;
description=pDesc;
product_id= pId;
model=mNumber;
sku=storeKu;
quantity=pQuantity;
imageUrl=pImage;
price=pPrice;
weight=pWeight;
length=pLength;
width=pWidth;
height=pHeight;
status=pStatus;
date_added=pDate;
viewed=pViewed;
}
return self;
}
@end
// // ProductDetailViewController.h
//
#import <UIKit/UIKit.h>
#import "Product.h"
#import "ProductEditViewController.h"
@interface ProductDetailViewController : UIViewController
@property(nonatomic, strong) IBOutlet UILabel *ProductNameLabel;
@property(nonatomic, retain) IBOutlet UITextView *ProductDescLabel;
@property(nonatomic, strong) IBOutlet UILabel *ProductIdLabel;
@property(nonatomic, strong) IBOutlet UILabel *ProductImageUrl;
@property(nonatomic, strong) IBOutlet UILabel *ProductModelLabel;
@property(nonatomic, strong) IBOutlet UILabel *ProductSkuLabel;
@property(nonatomic, strong) IBOutlet UILabel *ProductQuantLabel;
@property(nonatomic, strong) IBOutlet UILabel *ProductPriceLabel;
@property(nonatomic, strong) IBOutlet UILabel *ProductWeightLabel;
@property(nonatomic, strong) IBOutlet UILabel *ProductLenghtLabel;
@property(nonatomic, strong) IBOutlet UILabel *ProductWidthLabel;
@property(nonatomic, strong) IBOutlet UILabel *ProductHeightLabel;
@property(nonatomic, strong) IBOutlet UILabel *ProductStatusLabel;
@property(nonatomic, strong) IBOutlet UILabel *ProductDateAddedLabel;
@property(nonatomic, strong) IBOutlet UILabel *ProductViewedLabel;
@property(nonatomic, strong) IBOutlet UIImageView *productDetailImage;
@property(nonatomic, strong) ProductDetailViewController *productEdit;
@property(nonatomic, strong) Product * currentProduct;
#pragma mark -
#pragma mark Methods
-(void)getProduct:(id)productObject;
-(void)setProductLabels;
@end
// // ProductDetailViewController.m
#import "ProductDetailViewController.h"
#import "ProductEditViewController.h"
@interface ProductDetailViewController ()
@end
@implementation ProductDetailViewController
@synthesize ProductNameLabel, ProductDescLabel, ProductIdLabel, ProductModelLabel, ProductSkuLabel, ProductQuantLabel, ProductImageUrl, ProductPriceLabel, ProductWeightLabel, ProductLenghtLabel, ProductWidthLabel, ProductHeightLabel, ProductStatusLabel, ProductDateAddedLabel, ProductViewedLabel, productEdit;
- (id)initWithNibName:(NSString *)nibNameOrNil bundle:(NSBundle *)nibBundleOrNil
{
self = [super initWithNibName:nibNameOrNil bundle:nibBundleOrNil];
if (self) {
// Custom initialization
}
return self;
}
- (void)viewDidLoad
{
[super viewDidLoad];
// Do any additional setup after loading the view.
[self setProductLabels];
}
- (void)didReceiveMemoryWarning
{
[super didReceiveMemoryWarning];
// Dispose of any resources that can be recreated.
}
#pragma mark-
#pragma mark Methods
-(void)getProduct:(id)productObject{
_currentProduct = productObject;
}
-(void)setProductLabels{
ProductNameLabel.text = _currentProduct.name;
ProductDescLabel.text = _currentProduct.description;
ProductIdLabel.text = _currentProduct.product_id;
ProductModelLabel.text = _currentProduct.model;
ProductSkuLabel.text = _currentProduct.sku;
ProductQuantLabel.text = _currentProduct.quantity;
ProductPriceLabel.text = _currentProduct.price;
ProductWeightLabel.text = _currentProduct.weight;
ProductLenghtLabel.text = _currentProduct.length;
ProductWidthLabel.text = _currentProduct.width;
ProductHeightLabel.text = _currentProduct.height;
ProductStatusLabel.text = _currentProduct.status;
ProductDateAddedLabel.text = _currentProduct.date_added;
ProductViewedLabel.text = _currentProduct.viewed;
}
-(void) productEdit:(UILabel *) productNameLabel {
if (productNameLabel.tag == 1)
{
productEdit.ProductNameLabel.text = ProductNameLabel.text;
}
}
@end
//
ProductsViewController.h
//
#import <UIKit/UIKit.h>
@interface ProductsViewController : UITableViewController
//nsmutable array becuase it changes
@property (nonatomic, strong) NSMutableArray *jsonProductArray;
@property (nonatomic, strong) NSMutableArray *productsArray;
@property (strong, nonatomic) NSURL *productImage;
#pragma mark -
#pragma mark Class Methods
-(void) retrieveProductData;
@end
//
ProductsViewController.m
//
#import "ProductsViewController.h"
#import "Product.h"
#import "ProductDetailViewController.h"
#define getDataURL @"http://gkhns-macbook-pro.local/gj-4.php"
@interface ProductsViewController ()
@end
@implementation ProductsViewController
@synthesize jsonProductArray, productsArray;
- (id)initWithStyle:(UITableViewStyle)style
{
self = [super initWithStyle:style];
if (self) {
// Custom initialization
}
return self;
}
- (void)viewDidLoad
{
[super viewDidLoad];
// Uncomment the following line to preserve selection between presentations.
// self.clearsSelectionOnViewWillAppear = NO;
// Uncomment the following line to display an Edit button in the navigation bar for this view controller.
// self.navigationItem.rightBarButtonItem = self.editButtonItem;
//refresh
[self retrieveProductData];
UIRefreshControl *refresh = [[UIRefreshControl alloc]init];
refresh.attributedTitle = [[NSAttributedString alloc] initWithString:@"Pull to refresh"] ;
[refresh addTarget:self action:@selector(refreshProductView:) forControlEvents:UIControlEventValueChanged];
self.refreshControl=refresh;
self.title = @"PRODUCTS";
}
- (void)didReceiveMemoryWarning
{
[super didReceiveMemoryWarning];
// Dispose of any resources that can be recreated.
}
#pragma mark - Table view data source
- (NSInteger)numberOfSectionsInTableView:(UITableView *)tableView
{
// Return the number of sections.
return 1;
}
- (NSInteger)tableView:(UITableView *)tableView numberOfRowsInSection:(NSInteger)section
{
// Return the number of rows in the section.
return productsArray.count;
}
- (UITableViewCell *)tableView:(UITableView *)tableView cellForRowAtIndexPath:(NSIndexPath *)indexPath
{
static NSString *CellIdentifier = @"productCell";
UITableViewCell *cell = [tableView dequeueReusableCellWithIdentifier:CellIdentifier forIndexPath:indexPath];
if(cell==nil){
cell=[[UITableViewCell alloc] initWithStyle:UITableViewCellStyleSubtitle reuseIdentifier:@"productCell"];
cell.selectionStyle=UITableViewCellSelectionStyleNone;
}
// Configure the cell...
//create product image
Product *productObject;
productObject = [productsArray objectAtIndex:indexPath.row];
NSData *imageData = [NSData dataWithContentsOfURL:_productImage];
UIImage *imageLoad = [UIImage imageWithData:imageData];
cell.textLabel.text=productObject.name;
//load product image
cell.imageView.image=imageLoad;
//accessory
cell.accessoryType = UITableViewCellAccessoryDisclosureIndicator;
return cell;
}
/*
// Override to support conditional editing of the table view.
- (BOOL)tableView:(UITableView *)tableView canEditRowAtIndexPath:(NSIndexPath *)indexPath
{
// Return NO if you do not want the specified item to be editable.
return YES;
}
*/
/*
// Override to support editing the table view.
- (void)tableView:(UITableView *)tableView commitEditingStyle:(UITableViewCellEditingStyle)editingStyle forRowAtIndexPath:(NSIndexPath *)indexPath
{
if (editingStyle == UITableViewCellEditingStyleDelete) {
// Delete the row from the data source
[tableView deleteRowsAtIndexPaths:@[indexPath] withRowAnimation:UITableViewRowAnimationFade];
}
else if (editingStyle == UITableViewCellEditingStyleInsert) {
// Create a new instance of the appropriate class, insert it into the array, and add a new row to the table view
}
}
*/
/*
// Override to support rearranging the table view.
- (void)tableView:(UITableView *)tableView moveRowAtIndexPath:(NSIndexPath *)fromIndexPath toIndexPath:(NSIndexPath *)toIndexPath
{
}
*/
/*
// Override to support conditional rearranging of the table view.
- (BOOL)tableView:(UITableView *)tableView canMoveRowAtIndexPath:(NSIndexPath *)indexPath
{
// Return NO if you do not want the item to be re-orderable.
return YES;
}
*/
#pragma mark - Navigation
//In a story board-based application, you will often want to do a little preparation before navigation
- (void)prepareForSegue:(UIStoryboardSegue *)segue sender:(id)sender
{
// Get the new view controller using [segue destinationViewController].
// Pass the selected object to the new view controller.
if ([[segue identifier] isEqualToString:@"pushProductDetailView"]){
NSIndexPath *indexPath = [self.tableView indexPathForSelectedRow];
Product* object = [productsArray objectAtIndex:indexPath.row];
[[segue destinationViewController] getProduct:object];
}
}
#pragma mark -
#pragma mark Class Methods
-(void) retrieveProductData{
NSURL * url = [NSURL URLWithString:getDataURL];
NSData * data = [NSData dataWithContentsOfURL:url];
jsonProductArray=[NSJSONSerialization JSONObjectWithData:data options:kNilOptions error:nil];
//products array
productsArray= [[NSMutableArray alloc]init];
//loop through the jason array
for (int i=0; i<jsonProductArray.count; i++)
{
//create the product object
NSString *pName = [[jsonProductArray objectAtIndex:i] objectForKey:@"name"];
NSString *pId = [[jsonProductArray objectAtIndex:i] objectForKey:@"product_id"];
NSString *pDesc = [[jsonProductArray objectAtIndex:i] objectForKey:@"description"];
NSString *mNumber = [[jsonProductArray objectAtIndex:i] objectForKey:@"model"];
NSString *storeKu = [[jsonProductArray objectAtIndex:i] objectForKey:@"sku"];
NSString *pQuantity = [[jsonProductArray objectAtIndex:i] objectForKey:@"quantity"];
//product image url
NSURL *pImage = [[jsonProductArray objectAtIndex:i ] objectForKey:@"image"];
NSString *pPrice = [[jsonProductArray objectAtIndex:i] objectForKey:@"price"];
NSString *pWeight = [[jsonProductArray objectAtIndex:i] objectForKey:@"weight"];
NSString *pLength = [[jsonProductArray objectAtIndex:i] objectForKey:@"length"];
NSString *pWidth = [[jsonProductArray objectAtIndex:i] objectForKey:@"width"];
NSString *pHeight = [[jsonProductArray objectAtIndex:i] objectForKey:@"height"];
NSString *pStatus = [[jsonProductArray objectAtIndex:i] objectForKey:@"status"];
NSString *pDate = [[jsonProductArray objectAtIndex:i] objectForKey:@"date_added"];
NSString *pViewed = [[jsonProductArray objectAtIndex:i] objectForKey:@"viewed"];
//add product to products array
[productsArray addObject:[[Product alloc]initWithName: (NSString*)pName andProductDescription: (NSString *)pDesc andProductId: (NSString *)pId andModelNumber: (NSString*)mNumber andStoreKeepingUnit:(NSString*)storeKu andProductQuantity:(NSString*)pQuantity andProductImage:(NSURL*)pImage andProductPrice:(NSString*)pPrice andWeight:(NSString*)pWeight andLength:(NSString*)pLength andWidth:(NSString*)pWidth andHeight:(NSString*)pHeight andProductStatus:(NSString*)pStatus andDateAdded:(NSString*)pDate andProductViewed:(NSString*)pViewed]];
}
//reload the view
[self.tableView reloadData];
}
//refresh products (pull to refresh)
-(void) refreshProductView:(UIRefreshControl *)refreshProducts{
refreshProducts.attributedTitle = [[NSAttributedString alloc] initWithString:@"Updating products..."];
NSLog(@"Refreshing products");
[self retrieveProductData];
NSDateFormatter *dateFormatter = [[NSDateFormatter alloc]init];
[dateFormatter setDateFormat:@"MMM d, h:m a"];
NSString *lastUpdatedProduct = [NSString stringWithFormat:@"Last updated on %@", [dateFormatter stringFromDate:[NSDate date] ]];
refreshProducts.attributedTitle = [[NSAttributedString alloc]initWithString:lastUpdatedProduct];
[refreshProducts endRefreshing];
}
@end
// // ProductEditViewController.h
//
#import <UIKit/UIKit.h>
@interface ProductEditViewController : UIViewController
@property (strong, nonatomic) IBOutlet UITextField *ProductNameEditField;
@property (weak, nonatomic) IBOutlet UILabel *ProductNameEdit;
@end
ProductEditViewController.m
#import "ProductEditViewController.h"
@interface ProductEditViewController ()
@end
@implementation ProductEditViewController
- (id)initWithNibName:(NSString *)nibNameOrNil bundle:(NSBundle *)nibBundleOrNil
{
self = [super initWithNibName:nibNameOrNil bundle:nibBundleOrNil];
if (self) {
// Custom initialization
}
return self;
}
- (void)viewDidLoad
{
[super viewDidLoad];
// Do any additional setup after loading the view.
}
- (void)didReceiveMemoryWarning
{
[super didReceiveMemoryWarning];
// Dispose of any resources that can be recreated.
}
/*
#pragma mark - Navigation
// In a storyboard-based application, you will often want to do a little preparation before navigation
- (void)prepareForSegue:(UIStoryboardSegue *)segue sender:(id)sender
{
// Get the new view controller using [segue destinationViewController].
// Pass the selected object to the new view controller.
}
*/
@end
ProductNameEditField不是NSString,所以這就是問題所在。
嘗試這個:
productEdit.ProductNameEditField.text = productLabel.text;
您正在將ProductNameField
(這是一個UITextField
)設置為productLabel.text
(這是NSString
),但是您不能這樣做,因為它們不是同一類型的對象。
您可能想要:
productEdit.ProductNameEditField.text = productLabel.text;
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.