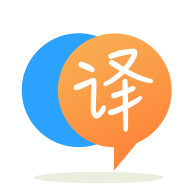
[英]largest palindrome number (it's the same number if you read it from left to right or right to left)
[英]see if string could be read the same way from both left and right using stack
我最近開始學習使用stack.my的問題是:我想從用戶那里獲取一個字符串並將其存儲在堆棧中,看看從左右讀取時該字符串是否相同,但是我不知道你可以幫我嗎? 到目前為止,這是我的程序:
#include <iostream>
#include <conio.h>
# define Size 100
using namespace std;
struct stack
{
int items[Size];
int MyTop;
};
int Empty(stack *s)
{
if(s->MyTop==-1)
return 1;
else
return 0;
}
void PushAndTest(int *Overflow,char x,stack *s)
{
if (s->MyTop==Size-1)
*Overflow=1;
else
{
*Overflow=0;
s->items[++(s->MyTop)]=x;
}
}
int PopAndTest(int *Underflow,char *x,stack *s)
{
if (Empty(s)!=1)
*Underflow=1;
else
{
*x=s->items[(s->MyTop)--];
*Underflow=0;
}
}
int TopAndTest(int *Underflow,char *x,stack *s)
{
if (s->MyTop==Size-1)
*Underflow=1;
else
{
*Underflow=0;
*x=s->items[(s->MyBottom)];
}
}
void Show(stack *s)
{
if(Empty(s)!=0)
cout<<"Underflow";
else
{
for(int i=0;i<=s->MyTop;i++)
{
cout<<s->items[i]<<endl;
}
}
}
int main()
{
int x,Underflow,Overflow,y,z;
stack s;
char ch,c;
s.MyTop=-1;
cout<<"Enter String: ";
while(cin.get( ch ) && ch != '\n')
{
PushAndTest(&Overflow,ch,&s);
}
return 0;
}
您需要從字符數組或std :: string類型的對象中讀取字符串,然后將其符號壓入堆棧,然后使用pop將字符串中的每個符號與堆棧中的對應符號進行比較。
至於您的代碼,則不得編譯並包含錯誤。 例如堆棧沒有數據成員MyBottom
了在函數中使用PushAndTest2
void PushAndTest2(int *Overflow,char x,stack *s)
{
s->items[--(s->MyBottom)]=x;
}
也不清楚函數主體中未使用的第二個參數char x
的平均值是什么(例如,對於函數PushAndTest
有效)
在函數PopAndTest
您嘗試在堆棧為空時返回一個元素
int PopAndTest(int *Underflow,char *x,stack *s)
{
if (Empty(s)!=1)
*Underflow=1;
else
{
*x=s->items[(s->MyTop)--];
*Underflow=0;
}
}
同樣也不清楚為什么您為什么將指向satck的指針作為函數的參數而不是使用引用。
例如,可以通過以下方式定義函數Empty
bool Empty( const stack &s )
{
return ( s.MyTop == -1 );
}
編輯:更新代碼后,我想指出一些函數具有未定義的行為。 例如,函數PopAndTest
具有返回類型int
時, PopAndTest
返回任何內容
int PopAndTest(int *Underflow,char *x,stack *s)
{
if (Empty(s)!=1)
*Underflow=1;
else
{
*x=s->items[(s->MyTop)--];
*Underflow=0;
}
}
我將枚舉定義為
enum class status { SUCCESS, UNDERFLOW, OVERFLOW };
並將其用作返回值。
status PopAndTest( stack &s )
{
if ( Empty( s ) ) return UNDERFLOW;
--s->MyTop;
return SUCCESS;
}
該函數不需要通過使用參數char *x
返回堆棧的元素。 只要有函數TopAndTest
即可將元素放在堆棧頂部。
要比較字符,可以使用以下方法。 假設字符數組str
包含一個字符串,並且您已經將其字符壓入堆棧。 那你可以寫
bool is_palindrome = true;
char *p = str;
while ( *p && is_palindrome )
{
char c;
is_palindrome = TopAndTest( s, &c ) == status::SUCCESS && *p++ == c );
PopAndTest( s );
}
我用了函數TopAndTest的聲明
status TopAndTest( stack &s, char *c );
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.