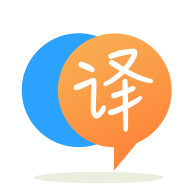
[英]Dynamically populate 3 dropdowns based on the selection in first dropdown
[英]Populate HTML/PHP Dropdown Based on First Dropdown Selection
我有 1 個用於類別(食物、飲料等)的下拉列表
在我的 MYSQL 表 (t_menu_category) 中,我有:
+----+---------------+-------------------+----------------------+
| ID | category_name | sub_category_name | category_description |
+----+---------------+-------------------+----------------------+
| 1 | Food | Curries | Spicy Curries |
| 2 | Food | Meat | Lamb, Pork, Chicken |
| 3 | Drinks | Alcohol | Fine Tasting Lager |
| 4 | Desserts | Cakes | Chocolate Cake |
+----+---------------+-------------------+----------------------+
我有第一個下拉列表顯示“category_name”的值,但我想要的是當我選擇食物時我希望第二個下拉框更新並只顯示“sub_category_name”的值,例如第一個選擇。 “食物”等於數據庫中的“食物”。
所以如果我在第一個下拉框中選擇了“食物”,第二個下拉框將只顯示“咖喱”和“肉”。
HTML:
<form method="post" action="<?php $_SERVER['PHP_SELF'] ?>">
<p>
<label for="item_name">Item Name</label>
<input id="item_name" name="item_name" required="required" type="text" placeholder="Item Name" />
</p>
<p>
<label for="item_description">Item Description</label>
<textarea rows="3" cols="100%" required="required" name="item_description">Item Description</textarea>
</p>
<p>
<label for="item_category">Item Category</label>
<select id="item_category" name="item_category" required="required">
<option selected="selected">-- Select Category --</option>
<?php
$sql = mysql_query("SELECT category_name FROM t_menu_category");
while ($row = mysql_fetch_array($sql)){
?>
<option value="<?php echo $row['category_name']; ?>"><?php echo $row['category_name']; ?></option>
<?php
// close while loop
}
?>
</select>
</p>
<p class="center"><input class="submit" type="submit" name="submit" value="Add Menu Item"/></p>
</form>
任何幫助都將受到高度贊賞:)
您可以使用請求創建一個 PHP 文件並使用 AJAX 調用它。
getSubCategory.php
<?php
$category = "";
if(isset($_GET['category'])){
$category = $_GET['category'];
}
/* Connect to the database, I'm using PDO but you could use mysqli */
$dsn = 'mysql:dbname=my_database;host=127.0.0.1';
$user = 'my_user';
$password = 'my_pass';
try {
$dbh = new PDO($dsn, $user, $password);
} catch (PDOException $e) {
echo 'Connection failed: ' . $e->getMessage();
}
$sql = 'SELECT sub_category_name as subCategory FROM t_menu_category WHERE category_name = :category';
$stmt = $dbh->prepare($sql);
$stmt->bindValue(':category', $category);
$stmt->execute();
return json_encode($stmt->fetchAll());
並添加一些 jquery 以在選擇類別時進行捕獲,並向服務器詢問相應的子類別:
<script>
$(document).ready(function () {
$('#item_category').on('change', function () {
//get selected value from category drop down
var category = $(this).val();
//select subcategory drop down
var selectSubCat = $('#item_sub_category');
if ( category != -1 ) {
// ask server for sub-categories
$.getJSON( "getSubCategory.php?category="+category)
.done(function( result) {
// append each sub-category to second drop down
$.each(result, function(item) {
selectSubCat.append($("<option />").val(item.subCategory).text(item.subCategory));
});
// enable sub-category drop down
selectSubCat.prop('disabled', false);
});
} else {
// disable sub-category drop down
selectSubCat.prop('disabled', 'disabled');
}
});
});
</script>
還要為您的第一個選項添加一個值:
<option value="-1" selected="selected">-- Select Category --</option>
我有一個簡單的解決方案來根據國家/地區選擇國家 php/javascript/mysql
MySQL表
country
country_code varhar(5)
country_name varchar(100)
state
country_code varhar(5)
state_code varchar(5)
country_name varchar(100)
main.php 文件中的國家/地區選擇
<html>
<body>
Country
<?php
$sql="SELECT * FROM country order by country_name";
$rs=$conn->Execute($sql);
echo '<select value="'.$country_code.'" name="country_code" id="country_list" onChange="stateList(this.value);" />';
echo '<option value="">--Select--</option>';
$rs->MoveFirst();
while (!$rs->EOF) {
echo '<option value="'.$rs->fields['country_code'].'"';
if ($rs->fields['country_code'] == $country_code) {echo " selected";}
echo '>'.$rs->fields['country_name'].'</option>';
$rs->MoveNext();
}
echo '</select>';
?>
State
<?php
$sql="SELECT * FROM state where contry_code = '$country_code' order by state_name";
$rs=$conn->Execute($sql);
echo '<select value="'.$state_code.'" name="state_code" id="state_list" />';
echo '<option value="">--Select--</option>';
$rs->MoveFirst();
while (!$rs->EOF) {
echo '<option value="'.$rs->fields['state_code'].'"';
if ($rs->fields['state_code'] == $state_code) {echo " selected";}
echo '>'.$rs->fields['state_name'].'</option>';
$rs->MoveNext();
}
echo '</select>';
?>
</body>
</html>
Java腳本
<script type="text/javascript">
function stateList(val) {
var select = document.getElementById( "state_list" );
var url = "get_statelist.php?country_code="+val;
$.ajax({
type: "GET",
url: url,
data:'',
success: function(data){
$("#state_list").html(data);
}
});
}
get_stataelist.php
<?php
session_start();
$country_code = $_GET['country_code'];
$conn = connect_db() //Make your own connection entry conn with Server,DB User ID, DB Password and DB Name
if ($country_code != "") {
$sql="SELECT * FROM state where coutry_code = '$country_code' order by state_name";
$rs=$conn->Execute($sql);
echo '<option value="">--Select--</option>';
$rs->MoveFirst();
while (!$rs->EOF) {
echo '<option value="'.$rs->fields['state_code'].'">'.$rs->fields['state_name']."</option>";
$rs->MoveNext();
}
}
?>
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.