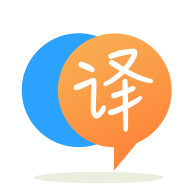
[英]Java - Find specific files (based on conditions) inside a given folder
[英]Find specific folder in Java
我正在尋找Java中特定文件夾的路徑,作為更大程序的一部分。
我所擁有的是一個遞歸函數,該函數檢查起始目錄中包含的每個文件,如果找到我要查找的文件夾,它將路徑作為字符串分配給變量。 以下代碼有效:
//root is the starting directory and name is the folder I am looking for
private void findDir(File root, String name)
{
if (root.getName().equals(name))
{
toFind = root.getAbsolutePath();
}
File[] files = root.listFiles();
if(files != null)
{
for (File f : files)
{
if(f.isDirectory())
{
findDir(f, name);
}
}
}
}
這可行,但是我不喜歡必須使用'toFind'變量的事實。 我的問題是有沒有辦法使該方法返回String而不是void? 找到要查找的文件后,這也將使程序不必檢查系統中的所有其他文件。
我在想類似的事情,但是即使找到該文件夾,以下代碼也將返回null。
private String findDir(File root, String name)
{
if (root.getName().equals(name))
{
return root.getAbsolutePath();
}
File[] files = root.listFiles();
if(files != null)
{
for (File f : files)
{
if(f.isDirectory())
{
return findDir(f, name);
}
}
}
return null; //???
}
這是因為在沒有子目錄樹的第一個目錄將返回null
因您指定如果結果的事實listFiles()
為null
,返回null
整個遞歸。 這不是立即顯而易見的,但是可以通過更改for循環中的行為來解決。 您應該測試結果是否為null
,而不是直接在for循環內返回結果,如果是,則繼續。 但是,如果結果為非null,則可以向上傳播結果。
private String findDir(File root, String name)
{
if (root.getName().equals(name))
{
return root.getAbsolutePath();
}
File[] files = root.listFiles();
if(files != null)
{
for (File f : files)
{
if(f.isDirectory())
{
String myResult = findDir(f, name);
//this just means this branch of the
//recursion reached the end of the
//directory tree without results, but
//we don't want to cut it short here,
//we still need to check the other
//directories, so continue the for loop
if (myResult == null) {
continue;
}
//we found a result so return!
else {
return myResult;
}
}
}
}
//we don't actually need to change this. It just means we reached
//the end of the directory tree (there are no more sub-directories
//in this directory) and didn't find the result
return null;
}
編輯 :根據蜘蛛的鮑里斯(Boris)的建議,我們實際上可以精簡if
語句,以避免continue
語句的笨拙性質,並使代碼更具體一點。 代替:
if (myResult == null) {
continue;
}
else {
return myResult;
}
我們可以直接滑入它的位置:
if (myResult != null) {
return myResult;
}
它將使用完全相同的邏輯進行評估,並且所需的總體代碼更少。
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.