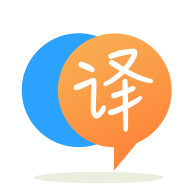
[英]Call a method from a class on something created by a different method in the same class?
[英]call all method of class that start with something
假設我們有一個這樣的類:
class Test(object):
def __init__(self):
pass
def fetch_a(self):
print "a"
def fetch_b(self):
print "b"
我想調用這個類的所有函數,這些函數以init函數中的“fetch”開頭。 我怎么做這項工作
你可以這樣做:
class Test(object):
def __init__(self):
for i in dir(self):
if i.startswith('fetch'):
result = getattr(self, i)()
def fetch_a(self):
print "a"
def fetch_b(self):
print "b"
>>> a = Test()
a
b
>>>
如果你只想調用以fetch
開頭的方法而不是變量,那么這就可以了:
class Test(object):
def __init__(self):
for i in dir(self):
result = getattr(self, i)
if i.startswith('fetch') and hasattr(result, '__call__'):
result()
我會這樣做:
def __init__(self):
wanted = [m for m in dir(Test) if m.startswith('fetch') and
hasattr(getattr(self, m), '__call__')]
for at in wanted:
end = getattr(self, at)()
所有答案都假設任何帶有fetch
的方法都是一種方法; 但這不能保證。 考慮這個例子:
class Foo(object):
fetch_a = 'hello'
def fetch_b(self):
return 'b'
你最終會得到TypeError: 'str' object is not callable
:
>>> a = Foo()
>>> for i in dir(a):
... if i.startswith('fetch'):
... print(getattr(a, i)())
...
Traceback (most recent call last):
File "<stdin>", line 3, in <module>
TypeError: 'str' object is not callable
您還需要檢查屬性是否為方法。 由於方法實現__call__
,您可以在檢查中使用它:
>>> for i in dir(a):
... if i.startswith('fetch') and hasattr(getattr(a, i), '__call__'):
... print(getattr(a, i)())
...
b
你也可以使用callable()
:
>>> for i in dir(a):
... if i.startswith('fetch') and callable(getattr(a, i)):
... print(getattr(a, i)())
...
b
此方法在Python 2.6中引入,在Python 3.0中刪除 ,然后在Python 3.2中重新引入。 所以要注意你的Python版本。
另一種方法是使用Python 2.1中引入的inspect
模塊中的 isfunction
:
>>> bar = lambda x: x
>>> callable(bar)
True
>>> import inspect
>>> inspect.isfunction(bar)
True
您可以嘗試使用dir:
class Test(object):
def __init__(self):
for name in dir(Test):
if len(name)>4 and name[:5] == "fetch":
eval("self." + name + "()")
def fetch_a(self):
print "a"
def fetch_b(self):
print "b"
z = Test()
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.