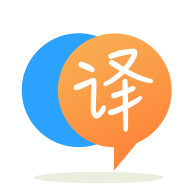
[英]Subtraction of date and time from file contents containing dates line by line
[英]Subtraction of Date and time
我正在嘗試從文件中減去連續兩行的日期和時間,例如:
String result1=line2-line1;
String result2=line4-line3;
// and so on.........
示例文件內容可能如下:
Thu Jan 16 2014 16:59:43.5020
Thu Jan 16 2014 16:59:43.5020
Thu Jan 16 2014 16:59:43.5020
Thu Jan 16 2014 16:59:43.5090
Thu Jan 16 2014 16:59:43.5100
Thu Jan 16 2014 16:59:43.5100
Thu Jan 16 2014 16:59:43.5170
Thu Jan 16 2014 16:59:43.9190
Thu Jan 16 2014 16:59:43.9200
Thu Jan 16 2014 16:59:43.9200
Thu Jan 16 2014 16:59:43.9200
Thu Jan 16 2014 16:59:43.9210
Thu Jan 16 2014 16:59:43.9210
Thu Jan 16 2014 16:59:43.9210
Thu Jan 16 2014 16:59:43.9210
Thu Jan 16 2014 16:59:43.9210
Thu Jan 16 2014 16:59:43.9220
Thu Jan 16 2014 16:59:43.9290
Thu Jan 16 2014 16:59:43.9290
Thu Jan 16 2014 16:59:43.9290
Thu Jan 16 2014 16:59:43.9330
Thu Jan 16 2014 16:59:44.0210
Thu Jan 16 2014 16:59:44.0210
Thu Jan 16 2014 16:59:44.0210
有潛移默化
DateFormat df = new SimpleDateFormat("EEE MMM dd yyyy HH:mm:ss.SSSS", Locale.ENGLISH);
System.out.println( df.parse(line2).getTime() - df.parse(line1).getTime() );
試試下面的代碼...
您可以根據需要實施基本邏輯
String string1 = "Oct 15, 2012 1:07:13 PM";
String string2 = "Oct 23, 2012 03:43:34 PM";
//here you want to mention the format that you want
SimpleDateFormat sdf = new SimpleDateFormat("MMM d, yyyy h:mm:ss a", Locale.ENGLISH);
Date date1 = sdf.parse(string1);
Date date2 = sdf.parse(string2);
//to found difference between two dates
long differenceInMillis = date2.getTime() - date1.getTime();
假設您已獲得文件的上下文。 字符串line1包含第一行中的文本,字符串line2包含第二行中的文本。
int datedifference=Integer.parseInt(line2.substring(8,10))-Integer.parseInt(line1.substring(8,10));
int yeardifference=Integer.parseInt(line2.substring(11,15))-Integer.parseInt(line1.substring(11,15));
int hourdifference=Integer.parseInt(line2.substring(16,18))-Integer.parseInt(line1.substring(16,18));
int minutedifference=Integer.parseInt(line2.substring(19,21))-Integer.parseInt(line1.substring(19,21));
int seconddifference=Integer.parseInt(line2.substring(22,23))-Integer.parseInt(line1.substring(22,23));
int lastthingdifference=Integer.parseInt(line2.substring(24,28))-Integer.parseInt(line1.substring(24,28));
然后,您獲得了所需的所有信息,只需按照自己喜歡的方式對其進行調整
注意:對於日期,您可能必須設置一個將天轉換成int的邏輯。
干杯
這也會修剪掉所有空白...我在這里得到了,您應該非常小心地提及要提取和減去的字符串索引,例如:
startIndex:starts from index 0(inclusive).
endIndex:starts from index 1(exclusive).
int datedifference = Integer.parseInt(line2.substring(8,10).trim())-Integer.parseInt(line1.substring(8,10).trim());
Duration.between( // Calculate and represent the span of time elapsed.
LocalDateTime.parse( // Parse the input string as a `LocalDateTime` because it lacks any indicator of time zone or offset-from-UTC.
"Thu Jan 16 2014 16:59:43.5020" , // Work with each pair of input strings.
DateTimeFormatter.ofPattern( "EEE MMM dd uuuu HH:mm:ss.SSSS" , Locale.US ) // Define a formatting pattern to match your input. Specify human language for translation via `Locale` object.
) ,
LocalDateTime.parse(
"Thu Jan 16 2014 16:59:43.5090" ,
DateTimeFormatter.ofPattern( "EEE MMM dd uuuu HH:mm:ss.SSSS" , Locale.US )
)
)
以行List
的形式讀取文件。
List< String > lines = Files.readAllLines( pathGoesHere ) ;
順便說一句,問題中使用的格式很糟糕。
只要有可能,請僅使用標准ISO 8601格式將日期時間值序列化為文本。
現代方法使用行業領先的java.time類, 該類取代了麻煩的舊遺留類,例如Date
/ Calendar
/ SimpleDateFormat
。
定義與每行匹配的格式化模式。 請注意Locale
參數,該參數指定在翻譯文本時要使用的人類語言和文化規范。
DateTimeFormatter f = DateTimeFormatter.ofPattern( "EEE MMM dd uuuu HH:mm:ss.SSSS" , Locale.US ) ; // Thu Jan 16 2014 16:59:43.5020
將每個輸入行解析為LocalDateTime
因為輸入缺少任何時區或UTC偏移量指示符。
LocalDateTime ldt = LocalDateTime.parse( "Thu Jan 16 2014 16:59:43.5020" , f );
ldt.toString():2014-01-16T16:59:43.502
循環輸入行,緩存每對,然后進行比較。 使用Duration
類計算並表示經過的Duration
跨度。
for( int index = 0 , index < lines.size() , index + 2 ) { // Increment by two, as we are processing pairs together.
String inputStart = lines.get( index ) ;
LocalDateTime start = LocalDateTime.parse( inputStart , f ) ;
String inputStop = lines.get( index + 1 ) ; // Move index to second of this pair of lines.
LocalDateTime stop = LocalDateTime.parse( inputStop , f ) ;
// Calculate the time elapsed using generic 24-hour based days, as we lack any time zone or offset-from-UTC.
Duration d = Duration.between( start , stop ) ;
}
您可以通過多種方式使用Duration
對象:
to…Part
方法)。 PnYnMnDTnHnMnS
的字符串,其中P
標記開始,而T
則將任何年月日與小時-分鍾-秒分開。 plus
/ minus
方法。 請注意,上述解決方案是不值得信任的一個LocalDateTime
不代表時刻,是不是在時間軸上的一個點。 要確定時刻,您必須將LocalDateTime
放在特定時區的上下文中。 在那之前,它沒有真正的意義。 Duration
計算是使用24小時通用天數進行的,而不考慮諸如夏時制(DST)之類的異常情況。
如果您肯定知道輸入數據的預期時區,則應用ZoneId
以獲得ZonedDateTime
。
以continent/region
的格式指定正確的時區名稱 ,例如America/Montreal
, Africa/Casablanca
或Pacific/Auckland
。 切勿使用EST
或IST
等3-4個字母的縮寫,因為它們不是真實的時區,不是標准化的,甚至不是唯一的(!)。
ZoneId z = ZoneId.of( "America/Montreal" ) ;
ZonedDateTime zdtStart = start.atZone( z ) ;
然后繼續計算Duration
。
Duration d = Duration.between( zdtStart , zdtStop ) ;
java.time框架內置於Java 8及更高版本中。 這些類取代了麻煩的舊的舊式日期時間類,例如java.util.Date
, Calendar
和SimpleDateFormat
。
現在處於維護模式的Joda-Time項目建議遷移到java.time類。
要了解更多信息,請參見Oracle教程 。 並在Stack Overflow中搜索許多示例和說明。 規格為JSR 310 。
您可以直接與數據庫交換java.time對象。 使用與JDBC 4.2或更高版本兼容的JDBC驅動程序 。 不需要字符串,不需要java.sql.*
類。
在哪里獲取java.time類?
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.