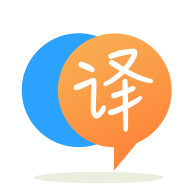
[英]c++ . I'm trying to get user input by using array inside switch but when i run the code it's showing segmentation fault?
[英]C++ Code compiles but I get a segmentation fault when running it. I think I'm mangling linked lists
大家好,先在這里發帖。 所以它進入周末,我無法訪問我的課程講師。 這是我們第一個使用鏈表的項目,我對它進行了很好的處理。 錯誤是,我很確定,在 sl_list.cpp output << temp -> contents();
第 53 行output << temp -> contents();
這是為了訪問節點的內容,在這種情況下是一個 int,並將它存儲在一個 stringstream 變量中,以便我可以稍后輸出整個內容。 顯然我做得不對。 我一直在嘗試修復它一天半的大部分時間,但我只是不知道我做錯了什么。 所有以“sl”開頭的文件都是我的,export_18.cpp 是我的導師創建的測試代碼。 如果我注釋掉有問題的函數,我的代碼將按預期編譯和運行。 這里有人能告訴我我搞砸了什么嗎? 它可能很容易被發現。 謝謝你。
#include "sl_list.h"
#include <iostream>
#include <sstream>
using namespace std;
using std::stringstream;
SLList::SLList() { // Sets head_ to NULL and size_ to 0
head_ = NULL;
size_ = 0;
}
SLList::~SLList() { // Destructor for SLList
}
void SLList::InsertHead(int number) { // Creates new dynamic SLNode
SLNode* to_insert = new SLNode(number);
to_insert -> set_next_node(head_);
head_ = to_insert;
size_++;
}
void SLList::RemoveHead() { // Remove the SLNode from the list
if (head_ != NULL) { // Check for NULL
SLNode* temp = head_; // Storage for temp location
head_ = head_ -> next_node(); // Set head to next_node
delete temp; // Delete temp storage
size_--; // Decrement size
}
}
void SLList::Clear() { // Clear the entire contents of the list
if (head_ != NULL) { // Check for NULL
while (head_ != NULL) { // Check for NULL
SLNode* temp = head_; // Storage for temp location
head_ = head_ -> next_node(); // Set head to next_node
delete temp; // Delete temp storage
size_--; // Decrement size
}
}
}
unsigned int SLList::size() const { // return size_
return size_;
}
string SLList::ToString() const { // returns the contents of all nodes
if (head_ != NULL) { // Check for NULL
stringstream output; // Storage for stringstream
SLNode* temp = head_; // Storage for temp location
while (temp != NULL) { // Check for NULL
temp = temp -> next_node(); // Set head to next_node
output << temp -> contents(); // Dumping temp into output
if (temp != NULL) { // Add a space and comma to the end of output
output << ", ";
}
}
return output.str(); // Return output
} else {
return ""; // If head_ was NULL return empty string
}
}
#ifndef SL_LIST_H
#define SL_LIST_H
#include "sl_node.h"
#include <iostream>
using std::string;
class SLList {
public:
SLList(); // Default constructor
~SLList(); // Default destructor
void InsertHead(int number); // Creates new dynamic SLNode
void RemoveHead(); // Remove the SLNode from the list
void Clear(); // clear the entire contents of the list
unsigned int size() const; // Return size_
string ToString() const; // returns the contents of all nodes
private:
SLNode* head_; // Storage for the next node locations
unsigned int size_; // Storage for the size
};
#endif /* SL_LIST.H */
#include "sl_node.h"
#include <iostream>
SLNode::SLNode() { // Default constructor
next_node_ = NULL;
contents_ = 0;
}
SLNode::SLNode(unsigned int contents) { // overloaded constructor
next_node_ = NULL;
contents_ = contents;
}
SLNode::~SLNode() { // Empty destructor
}
void SLNode::set_contents(int contents) { // Set contents_ to contents
contents_ = contents;
}
int SLNode::contents() const { // Return contents_
return contents_;
}
void SLNode::set_next_node(SLNode* next_node) { // Sets location of next node
next_node_ = next_node;
}
SLNode* SLNode::next_node() const { // Return next_node_
return next_node_;
}
#ifndef SL_NODE_H
#define SL_NODE_H
class SLNode {
public:
SLNode(); // Default constructor
SLNode(unsigned int contents); // Overloaded constructor
~SLNode(); // Empty destructor
void set_contents(int contents); // Set contents_ to contents
int contents() const; // Return contents_
void set_next_node(SLNode* next_node); // Sets location of next node
SLNode* next_node() const; // Return next_node_
private:
SLNode* next_node_; // Storage for the next node locations
int contents_; // Storage for the contents of the node
};
#endif /* SL_NODE.H */
#include "sl_list.h"
#include "sl_node.h"
#include <sstream>
#include <iostream>
using std::cout;
using std::endl;
using std::string;
using std::stringstream;
// For testing (DO NOT ALTER)
void Test(bool test, string more_info = "");
void UnitTest();
// Program Execution Starts Here
int main() {
// To test your code (DO NOT ALTER)
UnitTest();
// This ends program execution
return 0;
}
// For testing (DO NOT ALTER)
void UnitTest() {
string temp = "This unit test will test some of your code:\n";
cout << temp << string(temp.length() - 1, '-') << endl;
// Tests
SLList list;
stringstream full_list, half_list;
for (int i = 999; i > 0; i--) {
full_list << i << ", ";
if (i < 500)
half_list << i << ", ";
}
full_list << 0;
half_list << 0;
Test(list.size() == 0, "Default Constructor & size()");
Test(list.ToString() == "", "ToString()");
list.RemoveHead();
Test(list.size() == 0, "RemoveHead() & size()");
list.InsertHead(1);
Test(list.size() == 1, "InsertHead(1) & size()");
Test(list.ToString() == "1", "ToString()");
list.RemoveHead();
Test(list.size() == 0, "RemoveHead() & size()");
Test(list.ToString() == "", "ToString()");
list.InsertHead(10);
list.InsertHead(20);
Test(list.size() == 2, "InsertHead(10), InsertHead(20) & size()");
Test(list.ToString() == "20, 10", "ToString()");
list.RemoveHead();
Test(list.size() == 1, "RemoveHead() & size()");
Test(list.ToString() == "10", "ToString()");
list.InsertHead(5);
Test(list.size() == 2, "InsertHead(5) & size()");
Test(list.ToString() == "5, 10", "ToString()");
list.Clear();
Test(list.size() == 0, "Clear() & size()");
Test(list.ToString() == "", "ToString()");
for (unsigned int i = 0; i < 1000; i++)
list.InsertHead(i);
Test(list.size() == 1000, "InsertHead() \"HIGH LOAD\" & size()");
Test(list.ToString() == full_list.str(), "ToString()");
for (unsigned int i = 0; i < 500; i++)
list.RemoveHead();
Test(list.size() == 500, "RemoveHead() \"HIGH LOAD / 2\" & size()");
Test(list.ToString() == half_list.str(), "ToString()");
for (unsigned int i = 0; i < 600; i++)
list.RemoveHead();
Test(list.size() == 0, "RemoveHead() \"HIGH LOAD / 2\" & size()");
Test(list.ToString() == "", "ToString()");
cout << string(temp.length() - 1, '-') << endl;
cout << "Unit Test Complete!\n\n";
}
// For testing (DO NOT ALTER)
void Test(bool test, string more_info) {
static int test_number = 1;
if (test)
cout << "PASSSED TEST ";
else
cout << "FAILED TEST ";
cout << test_number << " " << more_info << "!\n";
test_number++;
}
在您的輸出循環中,您將 temp 移至下一個節點,然后再輸出其值。 只需交換這兩條線。
temp = temp -> next_node(); // Set head to next_node
output << temp -> contents(); // Dumping temp into output
應該成為
output << temp -> contents(); // Dumping temp into output
temp = temp -> next_node(); // Set head to next_node
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.