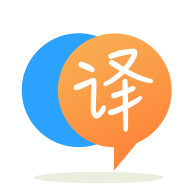
[英]How can I draw a semi transparent coloured mask over an already drawn to SWT canvas?
[英]How can I draw with canvas over an xml background?
我正在為一個簡單的研究項目工作,但我一直堅持這個問題。 我正在構建“蛇和梯子”應用程序,並且試圖使我的播放器(PNG圖像)在板上動態地運動。
我希望動畫發生在我在xml文件中定義的游戲棋盤背景上,但我做不到。
我要附加的程序無法正常工作,我不知道為什么。 另外,我需要添加考慮xml背景的部分,該部分丟失了,如果有人可以幫助我解決此問題,我將不勝感激。
提前致謝。
板子xml文件(game.xml):
<?xml version="1.0" encoding="utf-8"?>
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:background="@drawable/easymap"
android:orientation="vertical" >
<TextView
android:id="@+id/whitePlayer"
android:layout_width="32dp"
android:layout_height="32dp"
android:background="@drawable/white"
android:visibility="gone" />
<TextView
android:id="@+id/blackPlayer"
android:layout_width="32dp"
android:layout_height="32dp"
android:background="@drawable/black"
android:visibility="gone" />
<Button
android:id="@+id/btRoll"
android:layout_width="160dp"
android:layout_height="50dp"
android:layout_alignParentLeft="true"
android:layout_alignTop="@+id/cubePic"
android:background="@drawable/buttonshape"
android:text="Roll"
android:textColor="#FFFFFF" />
<TextView
android:id="@+id/cubePic"
android:layout_width="100dp"
android:layout_height="100dp"
android:layout_alignParentBottom="true"
android:layout_alignParentRight="true"
android:layout_marginBottom="42dp"
android:layout_marginRight="22dp"
android:background="@drawable/cube" />
<TextView
android:id="@+id/tvTurn"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_alignBottom="@+id/cubePic"
android:layout_alignRight="@+id/btRoll"
android:text="Your turn!"
android:textColor="@color/green"
android:textSize="32dp"
android:textStyle="italic" />
</RelativeLayout>
The java class:
package com.example.snakesnladders;
import android.app.Activity;
import android.content.Context;
import android.graphics.Bitmap;
import android.graphics.BitmapFactory;
import android.graphics.Canvas;
import android.os.Bundle;
import android.util.Log;
import android.view.SurfaceHolder;
import android.view.SurfaceView;
import android.view.View;
import android.view.View.OnClickListener;
import android.widget.Button;
import android.widget.TextView;
public class GFX_Game extends Activity implements OnClickListener {
TextView whitePlayer, blackPlayer;
Button roll;
TextView cube, map1, map2, map3, label;
boolean yourTurn = true;
MyBringBack ourView;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
ourView = new MyBringBack(this);
setContentView(ourView);
init();
}
private void init() {
cube = (TextView) findViewById(R.id.cubePic);
roll = (Button) findViewById(R.id.btRoll);
label = (TextView) findViewById(R.id.tvTurn);
roll.setOnClickListener(this);
}
@Override
protected void onDestroy() {
super.onDestroy();
}
@Override
public void onClick(View view) {
int rand = (int) (Math.random() * 6) + 1;
}
class MyBringBack extends SurfaceView implements Runnable {
SurfaceHolder ourHolder;
Thread ourThread = null;
Bitmap backGround, playerB, playerW;
boolean isRunning = true;
public MyBringBack(Context context) {
super(context);
playerW = BitmapFactory.decodeResource(getResources(),
R.id.whitePlayer);
ourHolder = getHolder();
ourThread = new Thread(this);
ourThread.start();
}
@Override
public void run() {
while (isRunning) {
if (!ourHolder.getSurface().isValid())
continue;
Canvas canvas = ourHolder.lockCanvas();
canvas.drawBitmap(playerW, 0, 0, null);
ourHolder.unlockCanvasAndPost(canvas);
}
}
}
}
更改后的內部類:(我已經擴展了View,從Viev類覆蓋了onDraw方法,但仍然無法正常工作)另外,我想借鑒我在game.xml文件中創建的現有xml布局。
class MyBringBack extends View {
Bitmap playerW;
public MyBringBack(Context context) {
super(context);
playerW = BitmapFactory.decodeResource(getResources(),
R.id.whitePlayer);
}
@Override
protected void onDraw(Canvas canvas) {
// TODO Auto-generated method stub
super.onDraw(canvas);
canvas.drawBitmap(playerW,0,0,null);
}
}
創建一個自定義視圖,如下所示。 如果您的根視圖是RelativeLayout,並且需要在其上繪制,請擴展一個RelativeLayout
public final class MyRelativeLayout extends RelativeLayout {
Bitmap playerW;
public MyRelativeLayout(Context context) {
super(context);
init(context);
}
public MyRelativeLayout(Context context, AttributeSet attrs) {
super(context, attrs);
init(context);
}
public MyRelativeLayout(Context context, AttributeSet attrs, int style) {
super(context, atts, style);
init(context);
}
private void init(final Context context) {
playerW = BitmapFactory.decodeResource(context.getResources(),
R.id.whitePlayer);
}
@Override
protected void onDraw(Canvas canvas) {
super.onDraw(canvas);
canvas.drawBitmap(playerW,0,0,null);
}
}
然后在xml中使用它,如下所示
<?xml version="1.0" encoding="utf-8"?>
<your.package.name.package.MyRelativeLayout
xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent">
...
all the stuff here
<your.package.name.package.MyRelativeLayout>
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.