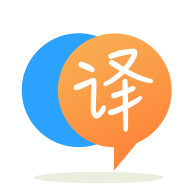
[英]Android tabs The specified child already has a parent. You must call removeView() on the child's parent first
[英]Android: You must call removeView() on the child's parent first 2
我在Android
創建動態添加行的表時遇到問題。 錯誤消息是:
指定的孩子已經有一個父母。 您必須先在孩子的父母上調用removeView()
但為什么?
void setCalendario(List<ArrayList<String>> l){
TableLayout table = (TableLayout)findViewById(R.id.list_tableLayout1);
TableRow tr = new TableRow(this);
tr.removeAllViews();
tr.setPadding(0,10,0,10);
tr.setLayoutParams(new LayoutParams(LayoutParams.MATCH_PARENT, LayoutParams.WRAP_CONTENT));
TextView tv_item1 = new TextView(this);
TextView tv_item2 = new TextView(this);
TextView tv_item3 = new TextView(this);
for (ArrayList<String> al : l){
int i = 0;
for(String s : al){
if (i == 0){
i++;
tv_item1.setText(s);
tv_item1.setGravity(Gravity.CENTER);
}
if (i == 1){
tv_item2.setText(s);
tv_item2.setGravity(Gravity.CENTER);
i++;
}
if (i == 2){
tv_item3.setText(s);
tv_item3.setGravity(Gravity.CENTER);
tr.addView(tv_item1);
tr.addView(tv_item2);
tr.addView(tv_item3);
table.addView(tr, new TableLayout.LayoutParams(LayoutParams.MATCH_PARENT, LayoutParams.WRAP_CONTENT));
}
}
}
}
xml代碼:
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context="com.MSca.gorhinos.Calendario$PlaceholderFragment" >
<TableLayout
android:id="@+id/list_tableLayout1"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_marginTop="10dp"
android:stretchColumns="0,1,2,3" >
<TableRow
android:id="@+id/tableRow1"
android:layout_width="wrap_content"
android:layout_height="wrap_content">
<TextView
android:id="@+id/textView1"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:gravity="center"
android:textColor="#000000"/>
<TextView
android:id="@+id/textView2"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:gravity="center"
android:textColor="#000000"/>
<TextView
android:id="@+id/textView3"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:gravity="center"
android:textColor="#000000"/>
</TableRow>
</TableLayout>
</RelativeLayout>
因此,您一次創建了tv_item1,tv_item2和tv_item3。 然后為所有ArrayList循環添加此視圖
tr.addView(tv_item1);
tr.addView(tv_item2);
tr.addView(tv_item3);
在第二次迭代中,您已經將tv_item1添加到tr中。 您想再做一次。 我想您只需要將此行轉移即可循環:
TableRow tr = new TableRow(this);
tr.removeAllViews();
tr.setPadding(0,10,0,10);
tr.setLayoutParams(new LayoutParams(LayoutParams.MATCH_PARENT,LayoutParams.WRAP_CONTENT));
TextView tv_item1 = new TextView(this);
TextView tv_item2 = new TextView(this);
TextView tv_item3 = new TextView(this);
您正在使用for循環將對TextView
的相同引用添加到TableRow
。 因此,在循環的下一個迭代中,將相同的對象再次添加到TableRow
(或TableLayout
)中! 那時他們已經有父母了。
嘗試在(外部)for循環內初始化TableRow
和TextView
對象。
編輯:修改您的代碼。
void setCalendario(List<ArrayList<String>> l) {
// Here we initialize the objects we re-initialize every iteration of the loop
TableLayout table = (TableLayout)findViewById(R.id.list_tableLayout1);
for (ArrayList<String> al : l) {
TableRow tr = new TableRow(this);
// I can't believe a freshly initialized TableRow object has views attached...
tr.removeAllViews();
tr.setPadding(0,10,0,10);
// Not sure why these layout params are needed already, as they are specified
// when adding this TableRow to the TableLayout object as well.
tr.setLayoutParams(new LayoutParams(LayoutParams.MATCH_PARENT, LayoutParams.WRAP_CONTENT));
TextView tv_item1 = new TextView(this);
TextView tv_item2 = new TextView(this);
TextView tv_item3 = new TextView(this);
int i = 0;
for(String s : al) {
if (i == 0) {
i++;
tv_item1.setText(s);
tv_item1.setGravity(Gravity.CENTER);
}
if (i == 1) {
tv_item2.setText(s);
tv_item2.setGravity(Gravity.CENTER);
i++;
}
if (i == 2) {
tv_item3.setText(s);
tv_item3.setGravity(Gravity.CENTER);
tr.addView(tv_item1);
tr.addView(tv_item2);
tr.addView(tv_item3);
table.addView(tr, new TableLayout.LayoutParams(LayoutParams.MATCH_PARENT, LayoutParams.WRAP_CONTENT));
}
}
}
}
因為您嘗試多次放置相同的textView。 您只能使用一次,因此必須一次又一次地實例化它:
// you remove the definition of the text views here and you put it inside the loop
for (ArrayList<String> al : l){
int i = 0;
for(String s : al){
TextView tv_item2 = new TextView(this);
TextView tv_item1 = new TextView(this);
TextView tv_item3 = new TextView(this);
if (i == 0){
i++;
tv_item1.setText(s);
tv_item1.setGravity(Gravity.CENTER);
}
if (i == 1){
tv_item2.setText(s);
tv_item2.setGravity(Gravity.CENTER);
i++;
}
if (i == 2){
tv_item3.setText(s);
tv_item3.setGravity(Gravity.CENTER);
tr.addView(tv_item1);
tr.addView(tv_item2);
tr.addView(tv_item3);
table.addView(tr, new TableLayout.LayoutParams(LayoutParams.MATCH_PARENT, LayoutParams.WRAP_CONTENT));
}
}
}
這不是最好的方法,您應該創建一個方法,將“ i”作為參數並返回TextView(具有重心...和您的工作人員)。
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.