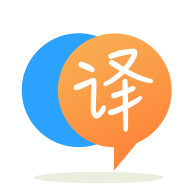
[英]Insert LocalStorage value into Form Input Field with Javascript
[英]Value from input field into array - Javascript, localstorage
我正在嘗試將輸入字段中的值插入到數組中,然后將其存儲在localstorage中,但問題是每次插入新值時它都會替換舊值,而我希望將其添加到現有值中。 忍受我,如果這是一個愚蠢的問題,我是一個新手:)
<html>
<body>
<form>
<input id="textInput" type=text>
<input type=button onclick="myFunction()" value="Add Number"/>
</form>
<div id="output"><div>
<script>
function myFunction() {
var sports = ["soccer", "baseball"];
var newSport = document.getElementById('textInput').value;
sports.push(newSport)
window.localStorage.setItem("sportskey", sports);
document.getElementById('output').innerHTML = window.localStorage.getItem('sportskey');
}
</script>
</body>
</html>
您的sports
變量是函數的本地變量,因此每次運行函數時都會重新初始化。 要修復它,請將聲明移到函數外部。
另外,正如@RocketHazmat指出的那樣,你只能存儲一個字符串。 因此,您的新代碼段應如下所示:
var sports = ["soccer", "baseball"]; //global scope
function myFunction() {
var newSport = document.getElementById('textInput').value;
sports.push(newSport)
window.localStorage.setItem("sportskey", JSON.stringify(sports)); // store as a string
document.getElementById('output').innerHTML = window.localStorage.getItem('sportskey');
}
問題在於變量的范圍。 您的sports數組必須在myFunction()函數之外聲明
<script>
var sports = ["soccer", "baseball"];
function myFunction() {
var newSport = document.getElementById('textInput').value;
sports.push(newSport );
var myString= JSON.stringify(sports);
window.localStorage.setItem("sportskey", myString);
document.getElementById('output').innerHTML = window.localStorage.getItem('sportskey');
}
</script>
每次調用myFunction()
時,您都在重新創建 sports
數組。 每次添加新元素時,都是從頭開始,添加第3個元素,忽略已添加的其他元素。
僅在localStorage
中不存在時才創建該數組。
PS您無法在本地存儲中存儲數組,只能存儲字符串。 您需要轉換為/從JSON轉換才能使其正常工作。
function myFunction() {
var sports = JSON.parse(window.localStorage.getItem('sportskey')) || ["soccer", "baseball"];
var newSport = document.getElementById('textInput').value;
sports.push(newSport)
var json = JSON.stringify(sports);
window.localStorage.setItem("sportskey", json);
document.getElementById('output').innerHTML = json;
}
演示: http : //jsfiddle.net/EhH8C/
Aaargh ......我的回答太遲了;)
是的,每次調用該方法時都要重新創建數組,並且在從localStore加載項目時應該在存儲項目時調用JSON.stringify(Array)和JSON.parse(string_from_localStore)。
但是你應該將stringify包裝在try和catch塊中,因為當json字符串格式不正確你的stringify方法崩潰函數並且它停止工作時,可能是某些原因。
var myFunc = function() {
//load it at first from localstorage
var sports = ["soccer", "baseball"];
var localSports = [];
try {
localSports = JSON.parse(window.localStorage.getItem('sportskey'));
if( localSports == null)
localSports = sports;
} catch(ex) {
console.log("something wrong");
localSports = sports; //fallback
}
var newSport = document.getElementById('textInput').value;
localSports.push(newSport); //this is an array
//insert the array with JSON.stringify so you can take it later out and rework with it
window.localStorage.setItem("sportskey", JSON.stringify(localSports));
output.innerHTML = window.localStorage.getItem('sportskey');
};
這是jsfiddle的鏈接:
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.