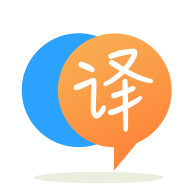
[英]Relative path to external dependencies in Slim PHP using $app->render()
[英]Shopify private app- php
更新了shopify.php
<?php
include('shopify_api_config.php');
class ShopifyClient {
public $shop_domain;
private $token;
private $api_key;
private $secret;
private $last_response_headers = null;
public function __construct($shop_domain, $token, $api_key, $secret) {
$this->name = "ShopifyClient";
$this->shop_domain = 'https://@4ef34cd22b136c1a7b869e77c8ce8b3c:@fb2b17c283a27c65e4461d0ce8e5871b@discountshop-8.myshopify.com';
$this->token = $token;
$this->api_key = '4ef34cd22b136c1a7b869e77c8ce8b3c';
$this->secret = '28cdbeb0b925bba5b8c9a60cfbb8c3cb';
$client = new ShopifyClient($shop_domain, $token, $api_key, $secret);
}
// Get the URL required to request authorization
public function getAuthorizeUrl($scope, $redirect_url='') {
$url = "http://{$this->shop_domain}/admin/oauth/authorize?client_id={$this->api_key}&scope=" . urlencode($scope);
if ($redirect_url != '')
{
$url .= "&redirect_uri=" . urlencode($redirect_url);
}
return $url;
}
// Once the User has authorized the app, call this with the code to get the access token
public function getAccessToken($code) {
// POST to POST https://SHOP_NAME.myshopify.com/admin/oauth/access_token
$url = "https://{$this->shop_domain}/admin/oauth/access_token";
$payload = "client_id={$this->api_key}&client_secret={$this->secret}&code=$code";
$response = $this->curlHttpApiRequest('POST', $url, '', $payload, array());
$response = json_decode($response, true);
if (isset($response['access_token']))
return $response['access_token'];
return '';
}
public function callsMade()
{
return $this->shopApiCallLimitParam(0);
}
public function callLimit()
{
return $this->shopApiCallLimitParam(1);
}
public function callsLeft($response_headers)
{
return $this->callLimit() - $this->callsMade();
}
public function call($method, $path, $params=array())
{
$baseurl = "https://{$this->shop_domain}/";
$url = $baseurl.ltrim($path, '/');
$query = in_array($method, array('GET','DELETE')) ? $params : array();
$payload = in_array($method, array('POST','PUT')) ? stripslashes(json_encode($params)) : array();
$request_headers = in_array($method, array('POST','PUT')) ? array("Content-Type: application/json; charset=utf-8", 'Expect:') : array();
// add auth headers
$request_headers[] = 'X-Shopify-Access-Token: ' . $this->token;
$response = $this->curlHttpApiRequest($method, $url, $query, $payload, $request_headers);
$response = json_decode($response, true);
if (isset($response['errors']) or ($this->last_response_headers['http_status_code'] >= 400))
throw new ShopifyApiException($method, $path, $params, $this->last_response_headers, $response);
return (is_array($response) and (count($response) > 0)) ? array_shift($response) : $response;
}
public function validateSignature($query)
{
if(!is_array($query) || empty($query['signature']) || !is_string($query['signature']))
return false;
foreach($query as $k => $v) {
if($k == 'signature') continue;
$signature[] = $k . '=' . $v;
}
sort($signature);
$signature = md5($this->secret . implode('', $signature));
return $query['signature'] == $signature;
}
private function curlHttpApiRequest($method, $url, $query='', $payload='', $request_headers=array())
{
$url = $this->curlAppendQuery($url, $query);
$ch = curl_init($url);
$this->curlSetopts($ch, $method, $payload, $request_headers);
$response = curl_exec($ch);
$errno = curl_errno($ch);
$error = curl_error($ch);
curl_close($ch);
if ($errno) throw new ShopifyCurlException($error, $errno);
list($message_headers, $message_body) = preg_split("/\r\n\r\n|\n\n|\r\r/", $response, 2);
$this->last_response_headers = $this->curlParseHeaders($message_headers);
return $message_body;
}
private function curlAppendQuery($url, $query)
{
if (empty($query)) return $url;
if (is_array($query)) return "$url?".http_build_query($query);
else return "$url?$query";
}
private function curlSetopts($ch, $method, $payload, $request_headers)
{
curl_setopt($ch, CURLOPT_HEADER, true);
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
curl_setopt($ch, CURLOPT_FOLLOWLOCATION, true);
curl_setopt($ch, CURLOPT_MAXREDIRS, 3);
curl_setopt($ch, CURLOPT_SSL_VERIFYPEER, true);
curl_setopt($ch, CURLOPT_SSL_VERIFYHOST, 2);
curl_setopt($ch, CURLOPT_USERAGENT, 'ohShopify-php-api-client');
curl_setopt($ch, CURLOPT_CONNECTTIMEOUT, 30);
curl_setopt($ch, CURLOPT_TIMEOUT, 30);
curl_setopt ($ch, CURLOPT_CUSTOMREQUEST, $method);
if (!empty($request_headers)) curl_setopt($ch, CURLOPT_HTTPHEADER, $request_headers);
if ($method != 'GET' && !empty($payload))
{
if (is_array($payload)) $payload = http_build_query($payload);
curl_setopt ($ch, CURLOPT_POSTFIELDS, $payload);
}
}
private function curlParseHeaders($message_headers)
{
$header_lines = preg_split("/\r\n|\n|\r/", $message_headers);
$headers = array();
list(, $headers['http_status_code'], $headers['http_status_message']) = explode(' ', trim(array_shift($header_lines)), 3);
foreach ($header_lines as $header_line)
{
list($name, $value) = explode(':', $header_line, 2);
$name = strtolower($name);
$headers[$name] = trim($value);
}
return $headers;
}
private function shopApiCallLimitParam($index)
{
if ($this->last_response_headers == null)
{
throw new Exception('Cannot be called before an API call.');
}
$params = explode('/', $this->last_response_headers['http_x_shopify_shop_api_call_limit']);
return (int) $params[$index];
}
}
class ShopifyCurlException extends Exception { }
class ShopifyApiException extends Exception
{
protected $method;
protected $path;
protected $params;
protected $response_headers;
protected $response;
function __construct($method, $path, $params, $response_headers, $response)
{
$this->method = $method;
$this->path = $path;
$this->params = $params;
$this->response_headers = $response_headers;
$this->response = $response;
parent::__construct($response_headers['http_status_message'], $response_headers['http_status_code']);
}
function getMethod() { return $this->method; }
function getPath() { return $this->path; }
function getParams() { return $this->params; }
function getResponseHeaders() { return $this->response_headers; }
function getResponse() { return $this->response; }
}
?>
我在shopify商店中安裝了一個私人應用程序以下載csv文件,安裝后,單擊“下載”按鈕時,它將顯示為
Fatal error: Uncaught exception 'ShopifyCurlException' with message 'Could not
resolve host: http:; Host not found' in C:\xampp\htdocs\cat\lib\shopify.php:102
Stack trace: #0 C:\xampp\htdocs\cat\lib\shopify.php(67): ShopifyClient->curlHttpApiRequest('GET', 'https://http://...', Array, Array, Array)
#1 C:\xampp\htdocs\cat\index-oauth.php(26): ShopifyClient->call('GET', 'admin/orders.js...', Array)
#2 {main} thrown in C:\xampp\htdocs\cat\lib\shopify.php on line 102.
我不知道如何解決。 如果有人知道,請幫助。 謝謝!。
我有同樣的問題。 嘗試這個。
在構造函數中,將以下參數作為$ shop_domain傳遞。
https:// @apikey:@password@hostname
將@ apiKey,@ password和主機名替換為您的值。 還要在主機名中保留“ @”。
I.e. @yourshop.com
$shop_domain = 'https://@apikey:@password@hostname';
$token = 'your token';
$key = 'your key';
$secret = 'your secret';
$client = new ShopifyClient($shop_domain, $token, $api_key, $secret);
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.