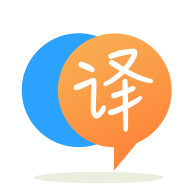
[英]html 5 canvas javascript rain animation(how to implement efficiently and easily!)
[英]rain drop animation on html canvas
我試圖在canvas上創建水滴下降效果。我使用array創建了一些樣本滴。為它設置動畫我的意思是改變它們的位置我引入了一個setTimeout函數。它適用於單個水滴。因為我的畫布上有多個滴它不再工作.drops仍然是靜止的。如果setTimeout函數可用於動畫所有單獨的drop?
這里是小提琴jsfiddle
單個拖放單滴動畫的動畫效果
<html>
<head>
</head>
<body>
<script>
function makeit(){
var canvas=document.getElementById("mycanvas");
var ctx=canvas.getContext('2d');
canvas.width=600;
canvas.height=600;
canvas.style.border="1px solid black";
var drop=function (x,y){
ctx.save();
ctx.fillStyle="orange";
ctx.fillRect(0,0,canvas.width,canvas.height);
ctx.fill();
ctx.fillStyle="red";
ctx.moveTo(x-5,y);
ctx.lineTo(x,y-7);
ctx.lineTo(x+5,y);
ctx.arc(x,y,5,0,Math.PI);
ctx.closePath();
ctx.fill();
ctx.restore();
y=y+3;
if(y==canvas.height){
y=0;
}
var m=setTimeout('drop('+x+','+y+')',20);
}
var xpos=[10,20,30,40,50,60,70,70,76];
var ypos=[30,20,60,80,76,90,30,40,79];
for(i=0;i<xpos.length;i++){
drop(xpos[i],ypos[i]);
}
}
window.onload=makeit;
</script>
<canvas id="mycanvas" ></canvas>
</body>
</html>
以下是我將如何做你想做的事情:
function makeit() {
// These variables can be used in the drawing functions
var canvas = document.getElementById("mycanvas");
var ctx = canvas.getContext('2d');
var drops = [];
// Init canvas & drops
init();
// Make the 3rd drop falls
var fall = setInterval(function () {
updateDrop(3);
}, 200);
// Make the 7th drop falls
var fall2 = setInterval(function () {
updateDrop(7);
}, 400);
// Stop the 3rd drop at anytime with this code :
// clearInterval(fall);
// Functions
function init() {
canvas.width = 600;
canvas.height = 600;
canvas.style.border = "1px solid black";
// Draw background
drawBackground();
// Draw drops
var xpos = [10, 20, 30, 40, 50, 60, 70, 70, 76];
var ypos = [30, 20, 60, 80, 76, 90, 30, 40, 79];
for (i = 0; i < xpos.length; i++) {
drops.push(drawDrop(xpos[i], ypos[i]));
}
}
function drawBackground() {
ctx.fillStyle = "orange";
ctx.fillRect(0, 0, canvas.width, canvas.height);
ctx.fill();
}
function drawDrop(x, y) {
ctx.beginPath();
ctx.fillStyle = "red";
ctx.moveTo(x - 5, y);
ctx.lineTo(x, y - 7);
ctx.lineTo(x + 5, y);
ctx.arc(x, y, 5, 0, Math.PI);
ctx.closePath();
ctx.fill();
return {
'x': x,
'y': y
};
}
function updateDrop(dropNumber) {
var dropNumber = dropNumber - 1; //Because 0 is first
// Update position
if (drops[dropNumber].y >= canvas.height) {
drops[dropNumber].y = 0;
} else {
drops[dropNumber].y += 3;
}
//Draw background
drawBackground();
//Draw drops
for (i = 0; i < drops.length; i++) {
drawDrop(drops[i].x, drops[i].y);
}
}
}
window.onload = makeit;
然后,如果你想阻止掉落的第三滴,你可以執行這一行:
clearInterval(fall);
只需看看JSFiddle: http : //jsfiddle.net/Oliboy50/QHRaS/5/
在繪制線之前不要忘記ctx.beginPath()
,否則你將無法清除它們。
這是使用window.requestAnimationFrame為drop創建動畫的另一個選項:
定義一個Drop偽類,它知道它的x,y位置和它下降的速度
添加Drop方法以重繪畫布上的拖放並增加其Y值以使其下降。
使用window.requestAnimationFrame創建一個動畫循環,以(1)通過填充橙色背景來清除畫布,(2)使每個下降落下並(3)重繪畫布上的每個下落。
帶注釋的代碼和演示: http : //jsfiddle.net/m1erickson/m8UPC/
<!doctype html>
<html>
<head>
<link rel="stylesheet" type="text/css" media="all" href="css/reset.css" /> <!-- reset css -->
<script type="text/javascript" src="http://code.jquery.com/jquery.min.js"></script>
<style>
body{ background-color: ivory; }
canvas{border:1px solid red;}
</style>
<script>
$(function(){
var canvas=document.getElementById("canvas");
var ctx=canvas.getContext("2d");
// a flag to let you stop the animation
var ok2animate=true;
// a Drop pseudo-class
// Properties: current x,y & the speed at which this drop falls
function Drop(){
this.x=Math.random()*(canvas.width-20);
this.y=-Math.random()*20;
this.fallRate=1;
}
// draw this drop on the canvas
Drop.prototype.draw=function(){
ctx.beginPath();
ctx.moveTo(this.x-5,this.y);
ctx.lineTo(this.x,this.y-7);
ctx.lineTo(this.x+5,this.y);
ctx.arc(this.x,this.y,5,0,Math.PI);
ctx.closePath();
ctx.fill();
return(this);
}
// make this drop "fall"
Drop.prototype.fall=function(){
this.y+=this.fallRate;
return(this);
}
// an animation loop to make some test drops fall
function animate(){
// request another animation frame
if(ok2animate){
requestAnimationFrame(animate);
}
// fill the canvas with the orange background
ctx.fillStyle="orange";
ctx.fillRect(0,0,canvas.width,canvas.height)
// make all drops fall and then redraw them
ctx.fillStyle="red";
for(var i=0;i<drops.length;i++){
drops[i].fall().draw();
}
}
// let the user stop the animation
$("#stop").click(function(){
ok2animate=false;
});
// an array of objects each representing 1 drop
var drops=[];
// add some test drops
for(var i=0;i<10;i++){
drops.push(new Drop());
}
// start the animation
requestAnimationFrame(animate);
}); // end $(function(){});
</script>
</head>
<body>
<button id="stop">Stop</button><br>
<canvas id="canvas" width=300 height=300></canvas>
</body>
</html>
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.