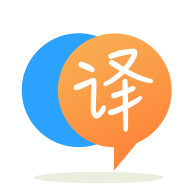
[英]Why does this window.open work on Chrome and FF but not IE?
[英]How to force open PDF on chrome/FF/IE PHP
<?PHP
require("../fpdf/fpdf.php");
include("db.classes.php");
$g = new DB();
$g->connection();
class PDF extends FPDF {
function header() {
$this->SetXY(10,0);
$this->image('../images/homeHeader.png');
}
function Footer()
{
// Go to 1.5 cm from bottom
$this->SetY(-15);
// Select Arial italic 8
$this->SetFont('Arial','I',8);
// Print centered page number
$this->Cell(0,10,'All Rights Reserved 2014',0,0,'C');
}
function BuildTable($header,$data) {
//Colors, line width and bold font
$this->SetFillColor(255,0,0);
$this->SetTextColor(255);
$this->SetDrawColor(128,0,0);
$this->SetLineWidth(.3);
$this->SetFont('','B');
//Header
// make an array for the column widths
$w=array(80,25,35,50,35,110);
// send the headers to the PDF document
for($i=0;$i<count($header);$i++)
$this->Cell($w[$i],7,$header[$i],1,0,'C',1);
$this->Ln();
//Color and font restoration
$this->SetFillColor(175);
$this->SetTextColor(0);
$this->SetFont('');
//now spool out the data from the $data array
$fill=true; // used to alternate row color backgrounds
foreach($data as $row)
{
// set colors to show a URL style link
$this->Cell($w[0],6,$row[0],'LR',0,'L',$fill);
// restore normal color settings
$this->SetTextColor(0);
$this->SetFont('');
$this->Cell($w[1],6,$row[1],'LR',0,'C',$fill);
$this->Cell($w[2],6,$row[2],'LR',0,'C',$fill);
$this->Cell($w[3],6,$row[3],'LR',0,'C',$fill);
$this->Cell($w[4],6,$row[4],'LR',0,'C',$fill);
$this->Cell($w[5],6,$row[5],'LR',0,'C',$fill);
$this->Ln();
// flips from true to false and vise versa
$fill =! $fill;
}
$this->Cell(array_sum($w),0,'','T');
}
}
$sql = "";
$result = "";
$plate = "";
if($_POST)
{
$vn = $g->clean($_POST["name"]);
$d1 = $g->clean($_POST["d1"]);
$d2 = $g->clean($_POST["d2"]);
$plate = $vn;
if($vn == "" && $d1 == "" && $d2 == "")
{
$sql = "SELECT vehiclelog_name, vehiclelog_plate, vehiclelog_date, vehiclelog_reftype, vehiclelog_refid, vehiclelog_description FROM vehiclelogs";
$result = mysqli_query($g->connection(), $sql)
or die( "Could not execute sql: $sql");
}
else if(!$vn == "" && $d1 == "" && $d2 == "")
{
$sql = "SELECT vehiclelog_name, vehiclelog_plate, vehiclelog_date, vehiclelog_reftype, vehiclelog_refid, vehiclelog_description FROM vehiclelogs
WHERE vehiclelog_plate='$vn'";
$result = mysqli_query($g->connection(), $sql)
or die( "Could not execute sql: $sql");
}
else if($vn == "" && !$d1 == "" && !$d2 == "")
{
$da1 = date('Y-m-d', strtotime($d1));
$da2 = date('Y-m-d', strtotime($d2));
$sql = "SELECT vehiclelog_name, vehiclelog_plate, vehiclelog_date, vehiclelog_reftype, vehiclelog_refid, vehiclelog_description FROM vehiclelogs
WHERE vehiclelog_date BETWEEN '$da1' AND '$da2'";
$result = mysqli_query($g->connection(), $sql)
or die( "Could not execute sql: $sql");
}
else if (!$vn == "" && !$d1 == "" && !$d2 == "")
{
$da1 = date('Y-m-d', strtotime($d1));
$da2 = date('Y-m-d', strtotime($d2));
$sql = "SELECT vehiclelog_name, vehiclelog_plate, vehiclelog_date, vehiclelog_reftype, vehiclelog_refid, vehiclelog_description FROM vehiclelogs
WHERE vehiclelog_date BETWEEN '$da1' AND '$da2' AND vehiclelog_plate='$vn'";
$result = mysqli_query($g->connection(), $sql)
or die( "Could not execute sql: $sql");
}
}
$getInfo = mysqli_query($g->connection(), "SELECT vehiclelog_name, vehiclelog_plate FROM vehiclelogs WHERE vehiclelog_plate='$vn'");
// build the data array from the database records.
While($row = mysqli_fetch_array($result)) {
$data[] = array($row['vehiclelog_name'], $row['vehiclelog_plate'], $row['vehiclelog_date'], $row['vehiclelog_reftype'],
$row['vehiclelog_refid'], $row['vehiclelog_description']);
}
$plateNo = "";
$model = "";
while($row = mysqli_fetch_array($getInfo))
{
$plateNo = $row['vehiclelog_plate'];
$model = $row['vehiclelog_name'];
}
// start and build the PDF document
$pdf = new PDF();
//Column titles
$header=array('Model','Plate', 'Date', 'Reference Type', 'Reference ID', 'Description');
$pdf->SetFont('Arial','',14);
$pdf->AddPage('L', 'Legal');
$pdf->SetXY(20,20);
$pdf->image('../images/DCWD Watermark.png');
$pdf->SetY(35);
// call the table creation method
$pdf->SetFont('Arial','B',16);
$pdf->Cell(0,10,$plateNo);
$pdf->SetY(45);
$pdf->SetFont('Arial','B',16);
$pdf->Cell(0,10,$model);
$pdf->SetXY(310, 45);
$pdf->SetFont('Arial','B',16);
$pdf->Cell(0,10,date("m/d/Y"));
$pdf->SetY(55);
$pdf->BuildTable($header,$data);
$pdf->Output();
?>
上面是單擊PRINT按鈕后調用的PHP,我創建的數據通過AJAX從我的輸入字段發送,然后查詢並打印為PDF 。
如何強制打開用FPDF制作的PDF文件? 每次我單擊PRINT按鈕時,它都隨IDM一起下載,但是當我關閉IDM的瀏覽器集成時,單擊“打印”不會發生任何事情。
將您的最后一行更改為:
$pdf->Output('', 'I');
第一個參數是您要賦予文件的名稱,第二個參數是文件的提供方式。
“我”會將pdf內聯發送到瀏覽器。
其他選項如下
I: send the file inline to the browser (default). The plug-in is used if available. The name given by name is used when one selects the "Save as" option on the link generating the PDF.
D: send to the browser and force a file download with the name given by name.
F: save to a local server file with the name given by name.
S: return the document as a string (name is ignored).
FI: equivalent to F + I option
FD: equivalent to F + D option
E: return the document as base64 mime multi-part email attachment (RFC 2045)
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.