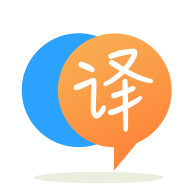
[英]How do I format input from a text file into a defaultdict in python
[英]How do I run a python file that takes a text file as input?
所以基本上我想在命令行中運行一個python文件,該文件讀取文本文件並顯示信息。
我想這個問題的一部分還在於文本文件是否應該與python文件位於同一目錄中?
這就是我所擁有的,我認為就代碼方面而言,這是正確的。
def print_words(filename):
input_file = open(filename, 'r')
print (input_file)
print_words(filename)
import sys
def print_words(file_name):
input_file = open(file_name, 'r')
lines = input_file.readlines()
lines = ' '.join(x for x in lines)
print(lines)
if __name__ == '__main__':
print_words(sys.argv[1])
該filename
可以是文件名的時候它是在同一文件夾中.py
文件。 否則,完整路徑作為filename
也將起作用。
編輯:sys.argv [1]將第二個命令行參數作為filename
。 這可以是絕對或相對filename
。
例如:在Windows中,當文件位於Python文件夾中時,可以在命令行中調用:c:\\ Python32> python print_words.py“ example.txt”
如果文件位於其他位置,則可以使用該文件:c:\\ Python32> python print_words.py“ C:\\ FOLDER \\ SUBFOLDER \\ example.txt”
編寫命令行腳本始於像您這樣的簡單要求(讓我僅輸入文件名),並很快導致更復雜的情況。
那時,某些命令行參數解析器很方便。
有很多選擇,其中一些是:
sys.argv
:不是命令行解析器,在更復雜的情況下會不足 argparse
:自Python 2.7起stdlib的一部分,但將需要多行額外代碼 plac
:非常有用的實用程序腳本,除非您遇到更復雜的情況 docopt
:到目前為止,我發現處理命令行解析的最好,最干凈的方法 docopt
命令行解析 首先安裝docopt:
$ pip install docopt
然后編寫腳本readfile.py
"""Read file (and possibly convert lines)
Usage:
readfile.py [(--uppercase|--lowercase|--swapcase)] [--reverse] <filename>...
readfile.py -h
Options:
-U --uppercase Convert text to uppercase
-L --lowercase Convert text to lowercase
-S --swapcase Swap character cases
-R --reverse Reverse text on each line
Reads file(s) <filename>, optionally convert lines to uppercase or lowercase and
possibly reverses text of each line.
Output is printed to stdout.
"""
import string
def get_convert_func(caseconvert="keep", reverse=False):
funcs = {"upper": string.upper, "lower": string.lower, "keep": lambda x: x}
casefunc = funcs[caseconvert]
if reverse:
return lambda line: "".join(list(casefunc(line))[::-1])
else:
return casefunc
def printfiles(filenames, convert_func):
for filename in filenames:
with open(filename) as f:
for line in f:
print convertfunc(line.strip("\n"))
到目前為止,我們為該文件和要調用的函數編寫了docstring。
readfile.py
繼續 但是真正的解析將到來(它必須在同一文件中,放在最后):
if __name__ == "__main__":
from docopt import docopt
args = docopt(__doc__)
print "args", args
filenames = args["<filename>"]
caseconvert = "keep"
if args["--uppercase"]:
caseconvert = "upper"
if args["--lowercase"]:
caseconvert = "lower"
reverse = args["--reverse"]
print "--------- Converted file content: ------------"
convertfunc = get_convert_func(caseconvert, reverse)
printfiles(filenames, convertfunc)
最后嘗試一下:
$ python readfile.py
Usage:
readfile.py [(--uppercase|--lowercase|--swapcase)] [--reverse] <filename>...
readfile.py -h
嘗試查看更多幫助:$ python readfile.py -h讀取文件(並可能轉換行)用法:readfile.py [(-大寫|-小寫| --swapcase)] [-反向] ... readfile .py -h
Options:
-U --uppercase Convert text to uppercase
-L --lowercase Convert text to lowercase
-S --swapcase Swap character cases
-R --reverse Reverse text on each line
Reads file(s) <filename>, optionally convert lines to uppercase or lowercase and
possibly reverses text of each line.
Output is printed to stdout.
並采取一些實際行動:
$ python readfile.py -UR readfile.py ../th.py
args {'--lowercase': False,
'--reverse': True,
'--swapcase': False,
'--uppercase': True,
'-h': False,
'<filename>': ['readfile.py', '../th.py']}
--------- Converted file content: ------------
)SENIL TREVNOC YLBISSOP DNA( ELIF DAER"""
:EGASU
...>EMANELIF< ]ESREVER--[ ])ESACPAWS--|ESACREWOL--|ESACREPPU--([ YP.ELIFDAER
H- YP.ELIFDAER
:SNOITPO
ESACREPPU OT TXET TREVNOC ESACREPPU-- U-
ESACREWOL OT TXET TREVNOC ESACREWOL-- L-
SESAC RETCARAHC PAWS ESACPAWS-- S-
....etc....
docopt
命令行解析規則的來源。 必須學習這些規則,但是它們並不是那么困難,並且它們能夠表達大量的不同組合。 docopt(__doc__)
正在解析所謂的docstring,它是從腳本文件的初始"""(some strint..."""
節中讀取的。 docopt
解析的結果打印在輸出上,以顯示您從解析命令行獲得的字典。 在生產代碼中,將其刪除。 docopt
導致具有非常易讀(且有效)的docstring作為腳本的基本組成部分。
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.