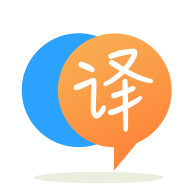
[英]How to redirect python output to both python console and a text file in Windows
[英]Python output on both console and file
我正在編寫代碼來分析 PDF 文件。 我想在控制台上顯示輸出以及在文件中復制輸出,我使用此代碼將輸出保存在文件中:
import sys
sys.stdout = open('C:\\users\\Suleiman JK\\Desktop\\file.txt',"w")
print "test"
但是我可以將輸出顯示到控制台中但不使用類,因為我不擅長它們嗎?
sys.stdout
可以指向任何具有 write 方法的對象,因此您可以創建一個同時寫入文件和控制台的類。
import sys
class LoggingPrinter:
def __init__(self, filename):
self.out_file = open(filename, "w")
self.old_stdout = sys.stdout
#this object will take over `stdout`'s job
sys.stdout = self
#executed when the user does a `print`
def write(self, text):
self.old_stdout.write(text)
self.out_file.write(text)
#executed when `with` block begins
def __enter__(self):
return self
#executed when `with` block ends
def __exit__(self, type, value, traceback):
#we don't want to log anymore. Restore the original stdout object.
sys.stdout = self.old_stdout
print "Entering section of program that will be logged."
with LoggingPrinter("result.txt"):
print "I've got a lovely bunch of coconuts."
print "Exiting logged section of program."
結果:
安慰:
Entering section of program that will be logged.
I've got a lovely bunch of coconuts.
Exiting logged section of program.
結果.txt:
I've got a lovely bunch of coconuts.
在某些情況下,這種方法可能比 codeparkle 更可取,因為您不必用logging.info
替換所有現有的print
。 只需將您想要登錄的所有內容放入with
塊即可。
(此答案使用 Python 3,如果您更喜歡 Python 2,則必須對其進行調整。)
首先導入 Python logging
包(以及用於訪問標准輸出流的sys
):
import logging
import sys
在入口點,為標准輸出流和輸出文件設置一個處理程序:
targets = logging.StreamHandler(sys.stdout), logging.FileHandler('test.log')
並將日志包配置為僅輸出沒有日志級別的消息:
logging.basicConfig(format='%(message)s', level=logging.INFO, handlers=targets)
現在你可以使用它:
>>> logging.info('testing the logging system')
testing the logging system
>>> logging.info('second message')
second message
>>> print(*open('test.log'), sep='')
testing the logging system
second message
您可以創建一個同時打印到控制台和文件的函數。 您可以通過切換標准輸出來實現,例如:
def print_both(file, *args):
temp = sys.stdout #assign console output to a variable
print ' '.join([str(arg) for arg in args])
sys.stdout = file
print ' '.join([str(arg) for arg in args])
sys.stdout = temp #set stdout back to console output
或使用文件寫入方法(我建議使用此方法,除非您必須使用 stdout)
def print_both(file, *args):
toprint = ' '.join([str(arg) for arg in args])
print toprint
file.write(toprint)
注意:
...像這樣:
print_both(open_file_variable, 'pass arguments as if it is', 'print!', 1, '!')
否則,您必須將所有內容都轉換為單個參數,即單個字符串。 它看起來像這樣:
print_both(open_file_variable, 'you should concatenate'+str(4334654)+'arguments together')
我仍然建議你學會正確使用類,你會從中受益匪淺。 希望這可以幫助。
我懶得寫一個函數,所以當我需要打印到控制台和文件時,我寫了這個快速且(不是那么)臟的代碼:
import sys
...
with open('myreport.txt', 'w') as f:
for out in [sys.stdout, f]:
print('some data', file=out)
print('some mre data', file=out)
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.