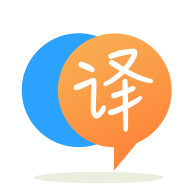
[英]Draw the zoomed & panned portion of a UIScrollview as a new UIImage
[英]Determining the center of a zoomed and panned image in UIScrollview
我正在為iPhone開發一個照片應用程序,該應用程序允許用戶使用相機拍攝照片或從“相機膠卷”中抓取一張照片,然后根據需要在UIScrollView中平移和縮放該圖像。 然后,用戶點擊按鈕以保存圖像。 我在使用key方法時遇到麻煩,該方法返回嵌入在scrollview中的imageview的可見區域的確切中心。 我需要這種方法來允許設備屏幕尺寸的變化(例如,iPhone 4 vs 5),以及源圖像的大小,縱橫比和縮放比例的變化。
舉例來說,我需要使它適用於以下情況:
另外,我也需要它來工作:
以下是我當前的代碼,該代碼試圖考慮屏幕尺寸和圖像尺寸的變化,但不適用於iPhone 4和5以及所有類型的圖像,例如縱向或橫向格式。 有沒有更簡單的方法來獲取滾動視圖的可見部分的中心點? 我需要對如何解釋和操作視圖的屬性(例如bounds.origin和bounds.size)以及如何在滾動視圖中使用內容大小和縮放比例有更清楚的了解。
我看過各種相似的問題,但似乎沒有一個問題足以說明設備或圖像縱橫比或大小的所有變化。 任何幫助將不勝感激!
類似問題
在UIScrollView內獲取UIImage可見部分的正確坐標
- (CGPoint)centerOfVisibleFrame:(UIImage *)image inScrollView:(UIScrollView *)scrollView
{
CGPoint frameCenter;
CGFloat zoomScale = scrollView.zoomScale;
// First determine the dimensions of the device
CGSize deviceFrameSize = [UIScreen mainScreen].bounds.size;
// Need to determine the scale factor for adjusting the image to full width/full height
CGFloat imageDeviceAspectFitScale;
// Compare the aspect ratio (height / width) of the device to the aspect ratio of the image
CGFloat deviceAspectRatio = deviceFrameSize.height / deviceFrameSize.width;
CGFloat imageAspectRatio = image.size.height / image.size.width;
// If the device's aspect ratio is greater than the image aspect ratio
if (deviceAspectRatio > imageAspectRatio)
{
// Set the imageDeviceAspectFitScale for full width
imageDeviceAspectFitScale = image.size.width / deviceFrameSize.width;
}
// Otherwise the image's aspect ratio is greater than the device aspect ratio
else
{
// Set the imageDeviceAspectFitScale for full height
imageDeviceAspectFitScale = image.size.height / deviceFrameSize.height;
}
// Create the frame for the image at full width or full height
CGSize imageAspectFitSize;
imageAspectFitSize.width = image.size.width / imageDeviceAspectFitScale;
imageAspectFitSize.height = image.size.height / imageDeviceAspectFitScale;
// Calculate the vertical and horizontal offset to adjust the coordinates of the
// image center to account for greater device height or device width
CGFloat verticalOffset = deviceFrameSize.height - imageAspectFitSize.height;
CGFloat horizontalOffset = deviceFrameSize.width - imageAspectFitSize.width;
if (self.debug) NSLog(@"verticalOffset = %f horizontalOffset = %f", verticalOffset, horizontalOffset);
if (self.debug) NSLog(@"image.size.width: %f image.size.height: %f", image.size.width, image.size.height);
if (self.debug) NSLog(@"scrollView.frame.size w: %f h: %f", scrollView.frame.size.width, scrollView.frame.size.height);
if (self.debug) NSLog(@"scrollView.bounds.size.width: %f scrollView.bounds.size.height: %f",
scrollView.bounds.size.width, scrollView.bounds.size.height);
if (self.debug) NSLog(@"scrollView.contentSize w=%f h=%f", scrollView.contentSize.width, scrollView.contentSize.height);
// imageRect represents the coordinate space for the image, adjusted for zoom scale
CGRect imageRect;
// First use the visible frame's origin to determine the top left corner of the visible rectangle
imageRect.origin.x = scrollView.contentOffset.x;
imageRect.origin.y = scrollView.contentOffset.y;
if (self.debug) NSLog(@"imageRect.origin x = %f y = %f", imageRect.origin.x, imageRect.origin.y);
// Adjust the image rect for zoom - Multiply by zoom scale
imageRect.size.width = image.size.width * zoomScale;
imageRect.size.height = image.size.height * zoomScale;
if (self.debug) NSLog(@"Zoomed imageRect.size width = %f height = %f", imageRect.size.width, imageRect.size.height);
// Then scale the image down to fit into the device frame
// Divide by the image device aspect fit scale
imageRect.size.width = imageRect.size.width / imageDeviceAspectFitScale;
imageRect.size.height = imageRect.size.height / imageDeviceAspectFitScale;
if (self.debug) NSLog(@"CVF Aspect fit imageRect.size width = %f height = %f", imageRect.size.width, imageRect.size.height);
// Then calculate the frame center by using the x and y dimensions of the DEVICE frame
frameCenter.x = imageRect.origin.x + (deviceFrameSize.width / 2);
frameCenter.y = imageRect.origin.y + (deviceFrameSize.height / 2);
// Scale back to original image dimensions from zoom
frameCenter.x = frameCenter.x / zoomScale;
frameCenter.y = frameCenter.y / zoomScale;
if (self.debug) NSLog(@"frameCenter.x = %f frameCenter.y = %f", frameCenter.x, frameCenter.y);
// Scale back up for the aspect fit scale
frameCenter.x = frameCenter.x * imageDeviceAspectFitScale;
frameCenter.y = frameCenter.y * imageDeviceAspectFitScale;
// Correct the coordinates for horizontal and vertical offset
frameCenter.x = frameCenter.x - (horizontalOffset);
frameCenter.y = frameCenter.y - (verticalOffset);
if (self.debug) NSLog(@"CVF frameCenter.x = %f frameCenter.y = %f", frameCenter.x, frameCenter.y);
return frameCenter;
}
基本上,這使您可以看到滾動視圖的矩形。
CGRect visibleRect = [yourScrollView convertRect:yourScrollView.bounds toView:yourImageview];
要么
CGRect visibleRect;
visibleRect.origin = scrollView.contentOffset;
visibleRect.size = scrollView.frame.size;
請查看此問題和答案以獲取更多詳細信息。 獲取UIScrollView的content的可見區域 。
只要您知道矩形,就可以輕松計算出中心點。
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.