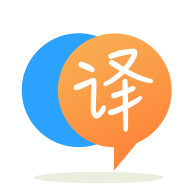
[英]Why i get error diamond operator is not supported in -source 1.5 in Java?
[英]Agenda code compilation via maven error: AgendaSample1Impl.java:[47,81] error: diamond operator is not supported in -source 1.5
我正在嘗試通過maven編譯JFXtras項目(默認JFXtras議程項目中給出的示例),但出現上述錯誤:
AgendaSample1Impl.java:[47,81] error: diamond operator is not supported in -source 1.5
XML如下:
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<groupId>Agenda</groupId>
<artifactId>Agenda</artifactId>
<version>1.0-SNAPSHOT</version>
<packaging>jar</packaging>
<name>Agenda</name>
<url>http://maven.apache.org</url>
<properties>
<project.build.sourceEncoding>UTF-8</project.build.sourceEncoding>
</properties>
<dependencies>
<dependency>
<groupId>junit</groupId>
<artifactId>junit</artifactId>
<version>3.8.1</version>
<scope>test</scope>
</dependency>
<dependency>
<groupId>org.jfxtras</groupId>
<artifactId>jfxtras-labs</artifactId>
<version>8.0-r1</version>
</dependency>
</dependencies>
</project>
java / maven版本如下:
Apache Maven 2.2.1 (rdebian-14)
Java version: 1.8.0_05
Java home: /usr/lib/jvm/java-8-oracle/jre
Default locale: en_US, platform encoding: UTF-8
OS name: "linux" version: "3.13.0-24-generic" arch: "i386" Family: "unix"
所說的JFXtras代碼看起來像這樣:
package agenda;
import java.io.IOException;
import javafx.application.Application;
import javafx.fxml.FXML;
import javafx.fxml.FXMLLoader;
import javafx.scene.Parent;
import javafx.scene.Scene;
import javafx.scene.layout.StackPane;
import javafx.stage.Stage;
public class AgendaSample1 extends Application {
@FXML
public static StackPane rootAgenda;
@Override
public void start(Stage primaryStage) throws IOException {
Parent root = FXMLLoader.load(getClass().getResource("AgendaSample1.fxml"));
rootAgenda.getChildren().add(new AgendaSample1Impl().lAgenda);
Scene scene = new Scene(root, 490, 490);
primaryStage.setTitle("Hello World!");
primaryStage.setScene(scene);
primaryStage.show();
}
public static void main(String[] args) {
launch(args);
}
}
package agenda;
import java.text.DateFormat;
import java.text.SimpleDateFormat;
import java.util.Calendar;
import java.util.Collection;
import java.util.GregorianCalendar;
import java.util.HashMap;
import java.util.Locale;
import java.util.Map;
import java.util.Random;
import java.util.concurrent.atomic.AtomicBoolean;
import javafx.beans.binding.Bindings;
import javafx.beans.value.ChangeListener;
import javafx.beans.value.ObservableValue;
import javafx.collections.FXCollections;
import javafx.collections.ListChangeListener;
import javafx.collections.ObservableList;
import javafx.scene.control.ListCell;
import javafx.scene.control.ListView;
import javafx.scene.layout.GridPane;
import javafx.scene.paint.Color;
import javafx.scene.shape.Rectangle;
import javafx.util.Callback;
import jfxtras.labs.scene.control.Agenda;
import jfxtras.labs.scene.control.Agenda.Appointment;
public class AgendaSample1Impl {
final Agenda lAgenda;
public AgendaSample1Impl() {
GridPane lGridPane = new GridPane();
lGridPane.setMaxSize(Integer.MAX_VALUE, Integer.MAX_VALUE);
AgendaSample1.rootAgenda.getChildren().add(lGridPane);
int lRowIdx = 0;
{
lAgenda = new Agenda();
lAgenda.setPrefSize(600, 600);
lAgenda.setMaxSize(Integer.MAX_VALUE, Integer.MAX_VALUE);
lGridPane.add(lAgenda, 0, lRowIdx, 2, 1);
lRowIdx++;
// setup appointment groups
final Map<String, Agenda.AppointmentGroup> lAppointmentGroupMap = new HashMap<>();
lAppointmentGroupMap.put("group0", new Agenda.AppointmentGroupImpl().withStyleClass("group0"));
lAppointmentGroupMap.put("group1", new Agenda.AppointmentGroupImpl().withStyleClass("group1"));
lAppointmentGroupMap.put("group2", new Agenda.AppointmentGroupImpl().withStyleClass("group2"));
lAppointmentGroupMap.put("group3", new Agenda.AppointmentGroupImpl().withStyleClass("group3"));
lAppointmentGroupMap.put("group4", new Agenda.AppointmentGroupImpl().withStyleClass("group4"));
lAppointmentGroupMap.put("group5", new Agenda.AppointmentGroupImpl().withStyleClass("group5"));
lAppointmentGroupMap.put("group6", new Agenda.AppointmentGroupImpl().withStyleClass("group6"));
lAppointmentGroupMap.put("group7", new Agenda.AppointmentGroupImpl().withStyleClass("group7"));
lAppointmentGroupMap.put("group8", new Agenda.AppointmentGroupImpl().withStyleClass("group8"));
lAppointmentGroupMap.put("group9", new Agenda.AppointmentGroupImpl().withStyleClass("group9"));
lAppointmentGroupMap.put("group10", new Agenda.AppointmentGroupImpl().withStyleClass("group10"));
lAppointmentGroupMap.put("group11", new Agenda.AppointmentGroupImpl().withStyleClass("group11"));
lAppointmentGroupMap.put("group12", new Agenda.AppointmentGroupImpl().withStyleClass("group12"));
lAppointmentGroupMap.put("group13", new Agenda.AppointmentGroupImpl().withStyleClass("group13"));
lAppointmentGroupMap.put("group14", new Agenda.AppointmentGroupImpl().withStyleClass("group14"));
lAppointmentGroupMap.put("group15", new Agenda.AppointmentGroupImpl().withStyleClass("group15"));
lAppointmentGroupMap.put("group16", new Agenda.AppointmentGroupImpl().withStyleClass("group16"));
lAppointmentGroupMap.put("group17", new Agenda.AppointmentGroupImpl().withStyleClass("group17"));
lAppointmentGroupMap.put("group18", new Agenda.AppointmentGroupImpl().withStyleClass("group18"));
lAppointmentGroupMap.put("group19", new Agenda.AppointmentGroupImpl().withStyleClass("group19"));
lAppointmentGroupMap.put("group0", new Agenda.AppointmentGroupImpl().withStyleClass("group20"));
lAppointmentGroupMap.put("group1", new Agenda.AppointmentGroupImpl().withStyleClass("group21"));
lAppointmentGroupMap.put("group2", new Agenda.AppointmentGroupImpl().withStyleClass("group22"));
lAppointmentGroupMap.put("group3", new Agenda.AppointmentGroupImpl().withStyleClass("group23"));
for (String lId : lAppointmentGroupMap.keySet()) {
Agenda.AppointmentGroup lAppointmentGroup = lAppointmentGroupMap.get(lId);
lAppointmentGroup.setDescription(lId);
lAgenda.appointmentGroups().add(lAppointmentGroup);
}
// accept new appointments
lAgenda.createAppointmentCallbackProperty().set(new Callback<Agenda.CalendarRange, Agenda.Appointment>() {
public Agenda.Appointment call(Agenda.CalendarRange calendarRange) {
return new Agenda.AppointmentImpl()
.withStartTime(calendarRange.getStartCalendar())
.withEndTime(calendarRange.getEndCalendar())
.withSummary("new")
.withDescription("new")
.withAppointmentGroup(lAppointmentGroupMap.get("group1"));
}
});
lAgenda.selectedAppointments().addListener(new ListChangeListener<Appointment>() {
@Override
public void onChanged(Change<? extends Appointment> c) {
Collection<Appointment> selectedAppointments = lAgenda.selectedAppointments();
ObservableList<Appointment> observableList = FXCollections.observableArrayList();
Bindings.bindContent(observableList, lAgenda.selectedAppointments());
ListView<Appointment> listView = new ListView<>(lAgenda.selectedAppointments());
listView.getSelectionModel().selectedItemProperty().addListener(
new ChangeListener<Appointment>() {
public void changed(ObservableValue<? extends Appointment> ov,
Appointment old_val, Appointment new_val) {
System.out.println(new_val);
}
});
}
});
// initial set
Calendar lFirstDayOfWeekCalendar = getFirstDayOfWeekCalendar(lAgenda.getLocale(), lAgenda.getDisplayedCalendar());
int lYear = lFirstDayOfWeekCalendar.get(Calendar.YEAR);
int lMonth = lFirstDayOfWeekCalendar.get(Calendar.MONTH);
int lDay = lFirstDayOfWeekCalendar.get(Calendar.DATE);
lAgenda.appointments().addAll(
new Agenda.AppointmentImpl()
.withStartTime(new GregorianCalendar(lYear, lMonth, lDay, 8, 00))
.withEndTime(new GregorianCalendar(lYear, lMonth, lDay, 11, 30))
.withSummary("A")
.withDescription("A much longer test description")
.withAppointmentGroup(lAppointmentGroupMap.get("group7")), new Agenda.AppointmentImpl()
.withStartTime(new GregorianCalendar(lYear, lMonth, lDay, 8, 30))
.withEndTime(new GregorianCalendar(lYear, lMonth, lDay, 10, 00))
.withSummary("B")
.withDescription("A description 2")
.withAppointmentGroup(lAppointmentGroupMap.get("group8")), new Agenda.AppointmentImpl()
.withStartTime(new GregorianCalendar(lYear, lMonth, lDay, 8, 30))
.withEndTime(new GregorianCalendar(lYear, lMonth, lDay, 9, 30))
.withSummary("C")
.withDescription("A description 3")
.withAppointmentGroup(lAppointmentGroupMap.get("group9")), new Agenda.AppointmentImpl()
.withStartTime(new GregorianCalendar(lYear, lMonth, lDay, 9, 00))
.withEndTime(new GregorianCalendar(lYear, lMonth, lDay, 13, 30))
.withSummary("D")
.withDescription("A description 4")
.withAppointmentGroup(lAppointmentGroupMap.get("group7")), new Agenda.AppointmentImpl()
.withStartTime(new GregorianCalendar(lYear, lMonth, lDay, 10, 30))
.withEndTime(new GregorianCalendar(lYear, lMonth, lDay, 11, 00))
.withSummary("E")
.withDescription("A description 4")
.withAppointmentGroup(lAppointmentGroupMap.get("group7")), new Agenda.AppointmentImpl()
.withStartTime(new GregorianCalendar(lYear, lMonth, lDay, 12, 30))
.withEndTime(new GregorianCalendar(lYear, lMonth, lDay, 13, 30))
.withSummary("F")
.withDescription("A description 4")
.withAppointmentGroup(lAppointmentGroupMap.get("group7")), new Agenda.AppointmentImpl()
.withStartTime(new GregorianCalendar(lYear, lMonth, lDay, 13, 00))
.withEndTime(new GregorianCalendar(lYear, lMonth, lDay, 13, 30))
.withSummary("H")
.withDescription("A description 4")
.withAppointmentGroup(lAppointmentGroupMap.get("group7")), new Agenda.AppointmentImpl()
.withStartTime(new GregorianCalendar(lYear, lMonth, lDay, 14, 00))
.withEndTime(new GregorianCalendar(lYear, lMonth, lDay, 14, 45))
.withSummary("G")
.withDescription("A description 4")
.withAppointmentGroup(lAppointmentGroupMap.get("group7")), new Agenda.AppointmentImpl()
.withStartTime(new GregorianCalendar(lYear, lMonth, lDay, 15, 00))
.withEndTime(new GregorianCalendar(lYear, lMonth, lDay, 16, 00))
.withSummary("I")
.withDescription("A description 4")
.withAppointmentGroup(lAppointmentGroupMap.get("group7")), new Agenda.AppointmentImpl()
.withStartTime(new GregorianCalendar(lYear, lMonth, lDay, 15, 30))
.withEndTime(new GregorianCalendar(lYear, lMonth, lDay, 16, 00))
.withSummary("J")
.withDescription("A description 4")
.withAppointmentGroup(lAppointmentGroupMap.get("group7")) // -----
, new Agenda.AppointmentImpl()
.withStartTime(new GregorianCalendar(lYear, lMonth, lDay))
.withSummary("all day1")
.withDescription("A description")
.withAppointmentGroup(lAppointmentGroupMap.get("group7"))
.withWholeDay(true), new Agenda.AppointmentImpl()
.withStartTime(new GregorianCalendar(lYear, lMonth, lDay))
.withSummary("all day2")
.withDescription("A description")
.withAppointmentGroup(lAppointmentGroupMap.get("group8"))
.withWholeDay(true), new Agenda.AppointmentImpl()
.withStartTime(new GregorianCalendar(lYear, lMonth, lDay))
.withSummary("all day3")
.withDescription("A description3")
.withAppointmentGroup(lAppointmentGroupMap.get("group9"))
.withWholeDay(true), new Agenda.AppointmentImpl()
.withStartTime(new GregorianCalendar(lYear, lMonth, lDay + 1))
.withSummary("all day")
.withDescription("A description3")
.withAppointmentGroup(lAppointmentGroupMap.get("group3"))
.withWholeDay(true)
);
final String lIpsum = "Pellentesque habitant morbi tristique senectus et netus et malesuada fames ac turpis egestas. Vestibulum tortor quam, feugiat vitae, ultricies eget, tempor sit amet, ante. Donec eu libero sit amet quam egestas semper. Aenean ultricies mi vitae est. Mauris placerat eleifend leo. Quisque sit amet est et sapien ullamcorper pharetra. Vestibulum erat wisi, condimentum sed, commodo vitae, ornare sit amet, wisi. Aenean fermentum, elit eget tincidunt condimentum, eros ipsum rutrum orci, sagittis tempus lacus enim ac dui. Donec non enim in turpis pulvinar facilisis. Ut felis. Praesent dapibus, neque id cursus faucibus, tortor neque egestas augue, eu vulputate magna eros eu erat. Aliquam erat volutpat. Nam dui mi, tincidunt quis, accumsan porttitor, facilisis luctus, metus";
// day spanner
{
Calendar lStart = (Calendar) lFirstDayOfWeekCalendar.clone();
lStart.add(Calendar.SECOND, 5);
lStart.add(Calendar.DATE, 1);
Calendar lEnd = (Calendar) lStart.clone();
lEnd.add(Calendar.DATE, 2);
Agenda.Appointment lAppointment = new Agenda.AppointmentImpl()
.withStartTime(lStart)
.withEndTime(lEnd)
.withSummary(lIpsum.substring(0, new Random().nextInt(50)))
.withDescription(lIpsum.substring(0, 10 + new Random().nextInt(lIpsum.length() - 10)))
.withAppointmentGroup(lAppointmentGroupMap.get("group" + (new Random().nextInt(3) + 1)));
lAgenda.appointments().add(lAppointment);
}
// update range
final AtomicBoolean lSkippedFirstRangeChange = new AtomicBoolean(false);
lAgenda.calendarRangeCallbackProperty().set(new Callback<Agenda.CalendarRange, Void>() {
public Void call(Agenda.CalendarRange arg0) {
// the first change should not be processed, because it is set above
if (lSkippedFirstRangeChange.get() == false) {
lSkippedFirstRangeChange.set(true);
return null;
}
// add a whole bunch of random appointments
for (int i = 0; i < 20; i++) {
Calendar lFirstDayOfWeekCalendar = getFirstDayOfWeekCalendar(lAgenda.getLocale(), lAgenda.getDisplayedCalendar());
Calendar lStart = (Calendar) lFirstDayOfWeekCalendar.clone();
lStart.add(Calendar.DATE, new Random().nextInt(7));
lStart.add(Calendar.HOUR_OF_DAY, new Random().nextInt(24));
lStart.add(Calendar.MINUTE, new Random().nextInt(60));
Calendar lEnd = (Calendar) lStart.clone();
lEnd.add(Calendar.MINUTE, 15 + new Random().nextInt(24 * 60));
Agenda.Appointment lAppointment = new Agenda.AppointmentImpl()
.withStartTime(lStart)
.withEndTime(lEnd)
.withWholeDay(new Random().nextInt(50) > 40)
.withSummary(lIpsum.substring(0, new Random().nextInt(50)))
.withDescription(lIpsum.substring(0, new Random().nextInt(lIpsum.length())))
.withAppointmentGroup(lAppointmentGroupMap.get("group" + (new Random().nextInt(24))));
lAgenda.appointments().add(lAppointment);
}
return null;
}
});
lAgenda.selectedAppointments().addListener(new ListChangeListener< Appointment>() {
public void onChanged(ListChangeListener.Change<? extends Appointment> c) {
while (c.next()) {
if (c.wasPermutated()) {
for (int i = c.getFrom(); i < c.getTo(); ++i) {
//permutate
}
} else if (c.wasUpdated()) {
//update item
} else {
for (Appointment a : c.getRemoved()) {
}
for (Appointment a : c.getAddedSubList()) {
printAppointment(a);
}
}
}
}
});
// ComboBox<String> lComboBox = new ComboBox<String>(FXCollections.observableArrayList("Single", "Range", "Multiple"));
// lComboBox.valueProperty().addListener(new ChangeListener<String>()
// {
// @Override
// public void changed(ObservableValue<? extends String> observableValue, String oldValue, String newValue)
// {
// if (newValue.startsWith("S")) lAgenda.setMode(Agenda.Mode.SINGLE);
// if (newValue.startsWith("R")) lAgenda.setMode(Agenda.Mode.RANGE);
// if (newValue.startsWith("M")) lAgenda.setMode(Agenda.Mode.MULTIPLE);
// }
// });
// lComboBox.setValue("Single");
// lComboBox.setPrefWidth(200);
// lGridPane.add(new Label("Mode:"), 0, lRowIdx);
// lGridPane.add(lComboBox, 1, lRowIdx);
// lRowIdx++;
}
}
/**
* get the calendar for the first day of the week
*/
static private Calendar getFirstDayOfWeekCalendar(Locale locale, Calendar c) {
// result
int lFirstDayOfWeek = Calendar.getInstance(locale).getFirstDayOfWeek();
int lCurrentDayOfWeek = c.get(Calendar.DAY_OF_WEEK);
int lDelta = 0;
if (lFirstDayOfWeek <= lCurrentDayOfWeek) {
lDelta = -lCurrentDayOfWeek + lFirstDayOfWeek;
} else {
lDelta = -lCurrentDayOfWeek - (7 - lFirstDayOfWeek);
}
c = ((Calendar) c.clone());
c.add(Calendar.DATE, lDelta);
return c;
}
static class ColorRectCell extends ListCell<String> {
@Override
public void updateItem(String item, boolean empty) {
super.updateItem(item, empty);
Rectangle rect = new Rectangle(100, 20);
if (item != null) {
rect.setFill(Color.web(item));
setGraphic(rect);
}
}
}
void printAppointment(Appointment a) {
System.out.println(a.getSummary());
System.out.println(a.getDescription());
DateFormat formatter = new SimpleDateFormat("yyyy. MMM EEE.d h:mma");
System.out.println(formatter.format(a.getStartTime().getTime()));
System.out.println(formatter.format(a.getEndTime().getTime()));
System.out.println(a.getAppointmentGroup());
System.out.println(a.getLocation());
this.daysBetween(a.getStartTime().getTimeInMillis(), a.getEndTime().getTimeInMillis());
}
private void daysBetween(long t1, long t2) {
int diff = (int) (t2 - t1);
long diffSeconds = diff / 1000;
long diffMinutes = diff / (60 * 1000);
long diffHours = diff / (60 * 60 * 1000);
long diffDays = diff / (24 * 60 * 60 * 1000);
System.out.println("diffSeconds difference = " + diffSeconds);
System.out.println("diffMinutes difference = " + diffMinutes);
System.out.println("diffHours difference = " + diffHours);
System.out.println("diffDays difference = " + diffDays);
}
}
Maven 2.2已停產,並且不再受支持-因此請不要使用它,而應使用當前版本(當前為Maven 3)。
您遇到的特定錯誤是因為您嘗試編譯的代碼使用Java 7功能,但是您的項目未設置為啟用這些功能(即使您使用Java 8運行)。
編譯器的source和target和target選項是在maven編譯器插件中設置的,該插件當前在其3.1版文檔中說明:
目前,默認源設置為1.5,默認目標設置為1.5,與運行Maven的JDK無關。 如果要更改這些默認值,則應設置源和目標。
有關如何為Java 8(您使用的Java版本)的編譯器源和目標配置maven的說明為:
<project>
[...]
<build>
[...]
<plugins>
<plugin>
<groupId>org.apache.maven.plugins</groupId>
<artifactId>maven-compiler-plugin</artifactId>
<version>3.1</version>
<configuration>
<source>1.8</source>
<target>1.8</target>
</configuration>
</plugin>
</plugins>
[...]
</build>
[...]
</project>
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.