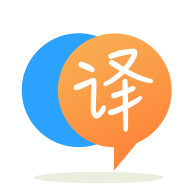
[英]Java StackOverflowError caused by recursion when running program for long periods of time
[英]How to avoid StackOverflowError caused by the number of recursion calls in Java?
我想解決這個問題: https : //www.hackerrank.com/challenges/find-median ,即在未排序的數組中找到中位數元素。 為此,我執行快速選擇算法。
我的程序可以在我的計算機上正常運行。 但是,當我在系統中提交時,它給了我StackOverflowError。 我認為這是由於遞歸調用的深度。 我猜我進行了太多的遞歸調用,而不是Java所允許的(錯誤是由具有10001數字的測試用例引起的)。 有人可以建議我如何避免這種情況嗎?
這是我的代碼:
import java.io.BufferedReader;
import java.io.IOException;
import java.io.InputStreamReader;
public class FindMedian {
static int res;
public static void main(String[] args) throws IOException {
BufferedReader br = new BufferedReader(
new InputStreamReader(System.in));
String line1 = br.readLine();
int N = Integer.parseInt(line1);
String[] line2 = br.readLine().split(" ");
int[] arr = new int[N];
for (int i = 0; i < N; i++) {
arr[i] = Integer.parseInt(line2[i]);
}
selectKth(arr, 0, N - 1);
System.out.println(res);
}
public static void selectKth(int[] arr, int start, int end) {
// it is written to select K-th element but actually
// it selects the median element
if (start >= end) {
res = arr[start];
return;
}
int pivot = arr[start];
int n = arr.length - 1;
int i = start + 1;
int j = end;
while (i <= j) {
while (arr[i] <= pivot) {
i++;
}
while (pivot < arr[j]) {
j--;
}
if (i < j) {
int tmp = arr[i];
arr[i] = arr[j];
arr[j] = tmp;
i++;
j--;
}
}
int tmp = arr[j];
// j is the index of the last element which is bigger or equal to pivot
arr[j] = pivot;
arr[start] = tmp;
if (n / 2 <= j) {
selectKth(arr, start, j);
} else {
selectKth(arr, i, end);
}
}
}
IT科學的基本概念是用迭代代替遞歸。 通過使用迭代,您將永遠不會遇到“遞歸太深”的錯誤。 每個問題都可以通過遞歸或迭代來解決。
詳細信息請參見我的鏈接。 http://www.refactoring.com/catalog/replaceRecursionWithIteration.html
由於您僅在其最末端調用selectKth
(實際上已經是尾部遞歸了),因此將遞歸展開為迭代很簡單。
func foo(param) {
if (param == bar) //bailout condition
return;
x = doStuff(param); //body of recursive method
foo(x); //recursive call
}
可以改寫為
func foo(param) {
x = param;
while ( x != bar ) {
x = doStuff( x );
}
}
在你的情況下,唯一棘手的比特是最后if
:
if(n/2 <= j){
selectKth(arr, start, j);
}
else{
selectKth(arr, i, end);
}
因此,您的方法將如下所示:
public static void selectKth(int[] arr, int start, int end) { //it is written seclect K-th element but actually it selects the median element
while( start < end ) {
//method body as is up to the last `if`
if(n/2 <= j) { //instead of recursion we just adjust start and end, then loop
end = j;
}
else{
start = i;
}
}
res = arr[start];
}
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.