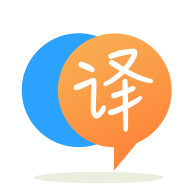
[英]Why does accept() block, when listen() is the very first involved in TCP?
[英]listen() does not block process
編輯:沒關系! 我花了數小時試圖獲取錯誤...我使用的是connect()而不是accept()...
編輯2:我想指定此代碼可能沒有意義,這是因為我目前正在研究該主題,因此您在本頁中找到的內容是我的第一個測試之一。 謝謝!
這是我正在編寫的一個非常簡單的服務器的代碼...我不明白為什么listen()
函數不會阻止直接進入connect()
的進程,而失敗卻給我錯誤22 - Invalid argument
。 有什么問題? 謝謝。
#include <stdio.h>
#include <sys/socket.h>
#include <sys/types.h>
#include <netinet/in.h>
#include <errno.h>
#define BUFMAXSIZE 1024
#define MAXLISTEN 10
#define SOCKERROR -1
int main(int argc, char *argv[]) {
int localPort;
int sockfd, sockbind, socklist, sockaccept;
struct sockaddr_in local, remote;
int remoteSize;
char readMsg[BUFMAXSIZE];
int totalReadLen, singleLoopRead;
char answer[BUFMAXSIZE];
int totalSentLen, singleLoopSent, answerLen;
// Checking parameters
if(argc < 2) {
printf("Usage: server LOCAL_PORT_NUMBER\n");
exit(1);
}
else {
localPort = atoi(argv[1]);
}
// Creating socket
printf("Creating socket...\n");
sockfd = socket(AF_INET, SOCK_STREAM, 0);
if(sockfd == SOCKERROR) {
printf("Error: could not open socket.\n");
exit(1);
}
printf("Socket created.\n");
// Setting local information
memset(&local, 0, sizeof(local));
local.sin_family = AF_INET;
local.sin_addr.s_addr = htonl(INADDR_ANY);
local.sin_port = htons(localPort);
// Binding
printf("Setting local information...\n");
sockbind = bind(sockfd, (struct sockaddr*) &local, sizeof(local));
if(sockbind == SOCKERROR) {
printf("Error: could not bind socket.\n");
exit(1);
}
printf("Socket binded.\n");
// Listening
printf("Listening on %d:%d...\n", ntohl(local.sin_addr.s_addr), ntohs(local.sin_port));
socklist = listen(sockfd, MAXLISTEN);
if(socklist == SOCKERROR) {
printf("Error: could not listen.\n");
exit(1);
}
// Accepting connection
printf("Accepting connection...\n");
memset(&remote, 0, sizeof(remote));
remoteSize = sizeof(remote);
sockaccept = connect(sockfd, (struct sockaddr*) &remote, &remoteSize);
if(sockaccept == SOCKERROR) {
printf("Error: could not accept connection.\n");
exit(1);
}
printf("Connection accepted.\n");
// Reading data
printf("Reading data...\n");
totalReadLen = 0;
singleLoopRead = 0;
while(BUFMAXSIZE > totalReadLen && (singleLoopRead = read(sockaccept, &(readMsg[totalReadLen]), BUFMAXSIZE - totalReadLen)) > 0) {
totalReadLen += singleLoopRead;
if(singleLoopRead < 0) {
printf("Error: could not read data.\n");
exit(1);
}
else {
printf("Data read: %d.\n", singleLoopRead);
}
}
printf("Read message: %s.", readMsg);
// Sending answer
printf("Sending answer...");
totalSentLen = 0;
singleLoopSent = 0;
strncpy(answer, "Data have been received.", BUFMAXSIZE - 1);
answerLen = strlen(answer);
fflush(stdout);
while((singleLoopSent = write(sockaccept, &(answer[totalSentLen]), answerLen - totalSentLen)) > 0) {
totalSentLen += singleLoopSent;
if(singleLoopSent < 0) {
printf("Error: could not send answer.\n");
fflush(stdout);
exit(1);
}
else {
printf("Total data sent: %d bytes.\n", totalSentLen);
}
}
fflush(stdout);
printf("Answer correctly sent.\n");
// Closing sockets
printf("Closing connection...\n");
close(sockaccept);
close(sockfd);
printf("Connection closed.\n");
return 0;
}
我不明白為什么listen()函數不會阻止該過程
因為它不是阻止功能。 它將端口置於LISTEN狀態。 它是accept()這是阻塞函數,您接下來應該調用它。
它直接進入connect(),失敗了,並給我錯誤22-無效的參數。
由於套接字處於LISTEN狀態,因為調用了listen() 。 您無法連接監聽插座。 您應該調用accept() 。
有什么問題?
問題是您的代碼沒有意義。
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.