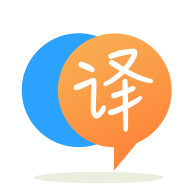
[英]Does a For-loop process a function inside the expression until the loop ends?
[英]Wait until all the function calls inside for-loop ends its execution - Javascript
我有一個函數,該函數在for循環中包含另一個函數調用。
outerFunction(){
for (var j = 0; j < geoAddress.length; j++) {
innerFunction(j);
}
}
我需要等到對innerFunction的所有調用完成。 如果需要並行執行這些功能,如何在JavaScript中實現呢?
檢出異步庫。 https://www.npmjs.org/package/async
查閱有關“ whilst”的文檔。 聽起來它確實可以滿足您的需求。 while(test,fn,callback)
var count = 0;
async.whilst(
function () { return count < 5; },
function (callback) {
count++;
setTimeout(callback, 1000);
},
function (err) {
// 5 seconds have passed
}
);
編輯-使用Q Promise庫以節點方式進行操作
如果您使用的是Q promise庫,請嘗試以下操作:
outerFunction(){
var promises = [];
for (var j = 0; j < geoAddress.length; j++) {
deferreds.push(innerFunction(j));
}
Q.all(promises).then(function(){
// do things after your inner functions run
});
}
即使您沒有使用這個特定的庫,原理也是一樣的。 應該像Q.denodify方法那樣,使函數返回一個Promise或將其包裝在Promise中,將所有調用推入一個Promise數組,然后將該數組傳遞給您的庫中相當於.when()(jQuery)或.all的等效項。 ()(Q Promise Library),然后在所有的諾言都解決后,使用.then()進行操作。
outerFunction() {
var done = 0;
function oneThreadDone() {
done++;
if (done === geoAddress.length) {
// do something when all done
}
}
for (var j = 0; j < geoAddress.length; j++) {
setTimeout(function() { innerFunction(j, oneThreadDone); }, 0);
}
}
並在inner_function內部調用oneThreadDone()函數(通過參數傳遞的引用)
如果您不想為此使用外部庫,則可以創建一個范圍對象,例如process
,它跟蹤仍在等待中的innerFunction
調用的數量,並在完成時調用外部回調cb
。
這樣做的目的是,您仍然可以獲得異步執行的好處,但是您只需確保在屬於outerFunction的所有innerFunction實際上完成之前,您的代碼就不會執行下一部分:
outerFunction(function() {
console.log("All done for outerFunction! - What you should do next?");
// This block is executed when all innerFunction calls are finished.
});
JavaScript:
// Example addresses
var geoAddress = ["Some address X", "Some address Y"];
var innerFunction = function(geoAddress, process) {
// Your work to be done is here...
// Now we use only setTimeout to demonstrate async method
setTimeout(function() {
console.log("innerFunction processing address: " + geoAddress);
// Ok, we update the process
process.done();
}, 500);
};
var outerFunction = function(cb) {
// Process object for tracking state of innerFunction executions
var process = {
// Total number of work ahead (number of innerFunction calls required).
count: geoAddress.length,
// Method which is triggered when some call of innerFunction finishes
done: function() {
// Decrease work pool
this.count--;
// Check if we are done & trigger a callback
if(this.count === 0) {
setTimeout(cb, 0);
}
}
};
for (var j = 0; j < geoAddress.length; j++) {
innerFunction(geoAddress[j], process);
}
};
// Testing our program
outerFunction(function() {
console.log("All done for outerFunction! - What you should do next?");
// This block is executed when all innerFunction calls are finished.
});
輸出:
innerFunction processing address: Some address X
innerFunction processing address: Some address Y
All done for outerFunction! - What you should do next?
這是js小提琴的例子
干杯。
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.