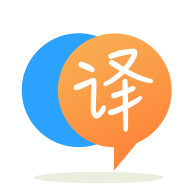
[英]javax.mail.AuthenticationFailedException: 535-5.7.8 Username and Password not accepted
[英]Could not connect to the message store javax.mail.AuthenticationFailedException: [AUTH] Username and password not accepted
嗨,我正在嘗試使用JavaMail在電子郵件中下載附件
此站點的參考: 使用JavaMail下載電子郵件中的附件
但是,我收到以下錯誤:
Could not connect to the message store javax.mail.AuthenticationFailedException: [AUTH] Username and password not accepted.
下面是代碼:
public class EmailAttachmentReceiver {
private String saveDirectory;
/**
* Sets the directory where attached files will be stored.
* @param dir absolute path of the directory
*/
public void setSaveDirectory(String dir) {
this.saveDirectory = dir;
}
/**
* Downloads new messages and saves attachments to disk if any.
* @param host
* @param port
* @param userName
* @param password
*/
public void downloadEmailAttachments(String host, String port,
String userName, String password) {
Properties properties = new Properties();
// server setting
properties.put("mail.pop3.host", host);
properties.put("mail.pop3.port", port);
// SSL setting
properties.setProperty("mail.pop3.socketFactory.class",
"javax.net.ssl.SSLSocketFactory");
properties.setProperty("mail.pop3.socketFactory.fallback", "false");
properties.setProperty("mail.pop3.socketFactory.port",
String.valueOf(port));
Session session = Session.getDefaultInstance(properties);
try {
// connects to the message store
Store store = session.getStore("pop3");
store.connect(userName, password);
// opens the inbox folder
Folder folderInbox = store.getFolder("INBOX");
folderInbox.open(Folder.READ_ONLY);
// fetches new messages from server
Message[] arrayMessages = folderInbox.getMessages();
for (int i = 0; i < arrayMessages.length; i++) {
Message message = arrayMessages[i];
Address[] fromAddress = message.getFrom();
String from = fromAddress[0].toString();
String subject = message.getSubject();
String sentDate = message.getSentDate().toString();
String contentType = message.getContentType();
String messageContent = "";
// store attachment file name, separated by comma
String attachFiles = "";
if (contentType.contains("multipart")) {
// content may contain attachments
Multipart multiPart = (Multipart) message.getContent();
int numberOfParts = multiPart.getCount();
for (int partCount = 0; partCount < numberOfParts; partCount++) {
MimeBodyPart part = (MimeBodyPart)
multiPart.getBodyPart(partCount);
if (Part.ATTACHMENT.equalsIgnoreCase(part.getDisposition())) {
// this part is attachment
String fileName = part.getFileName();
attachFiles += fileName + ", ";
part.saveFile(saveDirectory + File.separator + fileName);
} else {
// this part may be the message content
messageContent = part.getContent().toString();
}
}
if (attachFiles.length() > 1) {
attachFiles = attachFiles.substring(0, attachFiles.length() - 2);
}
} else if (contentType.contains("text/plain")
|| contentType.contains("text/html")) {
Object content = message.getContent();
if (content != null) {
messageContent = content.toString();
}
}
// print out details of each message
System.out.println("Message #" + (i + 1) + ":");
System.out.println("\t From: " + from);
System.out.println("\t Subject: " + subject);
System.out.println("\t Sent Date: " + sentDate);
System.out.println("\t Message: " + messageContent);
System.out.println("\t Attachments: " + attachFiles);
}
// disconnect
folderInbox.close(false);
store.close();
} catch (NoSuchProviderException ex) {
System.out.println("No provider for pop3.");
ex.printStackTrace();
} catch (MessagingException ex) {
System.out.println("Could not connect to the message store");
ex.printStackTrace();
} catch (IOException ex) {
ex.printStackTrace();
}
}
/**
* Runs this program with Gmail POP3 server
*/
public static void main(String[] args) {
String host = "pop.gmail.com";
String port = "995";
String userName = "your_email";
String password = "your_password";
String saveDirectory = "E:/Attachment";
EmailAttachmentReceiver receiver = new EmailAttachmentReceiver();
receiver.setSaveDirectory(saveDirectory);
receiver.downloadEmailAttachments(host, port, userName, password);
}
}
import com.packaging.dbconnection.Database;
import java.io.File;
import java.io.IOException;
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.PreparedStatement;
import java.sql.ResultSet;
import java.sql.SQLException;
import java.util.Properties;
import java.util.logging.Level;
import java.util.logging.Logger;
import javax.mail.Address;
import javax.mail.Folder;
import javax.mail.Message;
import javax.mail.MessagingException;
import javax.mail.Multipart;
import javax.mail.NoSuchProviderException;
import javax.mail.Part;
import javax.mail.Session;
import javax.mail.Store;
import javax.mail.internet.InternetAddress;
import javax.mail.internet.MimeBodyPart;
import javax.mail.search.FromTerm;
import javax.mail.search.SearchTerm;
/**
* This program demonstrates how to download e-mail messages and save
* attachments into files on disk.
*
* @author www.codejava.net
*
*/
public class EmailAttachmentReceiver {
private String saveDirectory;
/*vxcvxcv*/
/**
* Sets the directory where attached files will be stored.
* @param dir absolute path of the directory
*/
public void setSaveDirectory(String dir) {
this.saveDirectory = dir;
}
/**
* Downloads new messages and saves attachments to disk if any.
* @param host
* @param port
* @param userName
* @param password
*/
public void downloadEmailAttachments(String host, String port,
String userName, String password) {
PreparedStatement pst;
ResultSet rs;
Properties properties = new Properties();
properties.setProperty("mail.store.protocol", "imaps");
//session reading
// server setting
// properties.put("mail.pop3.host", host);
// properties.put("mail.pop3.port", port);
// SSL setting
// properties.setProperty("mail.pop3.socketFactory.class",
// "javax.net.ssl.SSLSocketFactory");
// properties.setProperty("mail.pop3.socketFactory.fallback", "false");
// properties.setProperty("mail.pop3.socketFactory.port",
// String.valueOf(port));
try {
// connects to the message store
Session session = Session.getDefaultInstance(properties);
Store store = session.getStore();
//store.connect(userName, password);
store.connect("imap.gmail.com", "yourmail@gmail.com", "yourpass");
//Folder inbox = store.getFolder("INBOX");
// inbox.open(Folder.READ_ONLY);
// opens the inbox folder
Folder folderInbox = store.getFolder("INBOX");
folderInbox.open(Folder.READ_ONLY);
// fetches new messages from server
SearchTerm sender = new FromTerm(new InternetAddress("xyz@gmail.com"));
// Message[] messages = inbox.search(sender);
Message[] arrayMessages = folderInbox.search(sender);
for (int i = 0; i < arrayMessages.length; i++) {
Message message = arrayMessages[i];
Address[] fromAddress = message.getFrom();
String from = fromAddress[0].toString();
String subject = message.getSubject();
String sentDate = message.getSentDate().toString();
String contentType = message.getContentType();
String messageContent = "";
// store attachment file name, separated by comma
String attachFiles = "";
if (contentType.contains("multipart")) {
// content may contain attachments
Multipart multiPart = (Multipart) message.getContent();
int numberOfParts = multiPart.getCount();
for (int partCount = 0; partCount < numberOfParts; partCount++) {
MimeBodyPart part = (MimeBodyPart) multiPart.getBodyPart(partCount);
if (Part.ATTACHMENT.equalsIgnoreCase(part.getDisposition())) {
// this part is attachment
String fileName = part.getFileName();
attachFiles += fileName + ", ";
part.saveFile(saveDirectory + File.separator + fileName);
} else {
// this part may be the message content
messageContent = part.getContent().toString();
}
}
if (attachFiles.length() > 1) {
attachFiles = attachFiles.substring(0, attachFiles.length() - 2);
}
} else if (contentType.contains("text/plain")
|| contentType.contains("text/html")) {
Object content = message.getContent();
if (content != null) {
messageContent = content.toString();
}
}
// print out details of each message
System.out.println("Message " + (i + 1) + ":");
System.out.println("\t From: " + from);
System.out.println("\t Subject: " + subject);
System.out.println("\t Recieved Date: " + sentDate);
System.out.println("\t Message: " + messageContent);
System.out.println("\t Attachments: " + attachFiles);
try{
String driver="com.mysql.jdbc.Driver";
String url="jdbc:mysql://localhost:3306/dbname";
String user="root";
String pass="";
Class.forName(driver);
Connection con=DriverManager.getConnection(url,user,pass);
pst=con.prepareStatement("insert into applications() VALUES (?,?,?,?,?)");
pst.setString(1,i+"1");
pst.setString(2,from);
pst.setString(3,subject);
pst.setString(4,sentDate);
pst.setString(5,attachFiles);
int j = pst.executeUpdate();
if(con!=null){
System.out.println("connected");
}
}
catch (ClassNotFoundException ex) {
Logger.getLogger(EmailAttachmentReceiver.class.getName()).log(Level.SEVERE, null, ex);
}
catch (SQLException ex) {
Logger.getLogger(EmailAttachmentReceiver.class.getName()).log(Level.SEVERE, null, ex);
}
}
// disconnect
folderInbox.close(false);
store.close();
} catch (NoSuchProviderException ex) {
System.out.println("No provider for pop3.");
ex.printStackTrace();
} catch (MessagingException ex) {
System.out.println("Could not connect to the message store");
ex.printStackTrace();
} catch (IOException ex) {
ex.printStackTrace();
}
}
/**
* Runs this program with Gmail POP3 server
*/
public static void main(String[] args) {
String host = "pop.gmail.com";
String port = "995";
String userName = "yourmail@gmail.com";
String password = "yourpass";
String saveDirectory = "D:/Attachment";
EmailAttachmentReceiver receiver = new EmailAttachmentReceiver();
receiver.setSaveDirectory(saveDirectory);
receiver.downloadEmailAttachments(host, port, userName, password);
}
}
在上面的代碼中,我給出了下載附件代碼,並以此將電子郵件也保存在數據庫中的D:/ Attachment文件夾中,因此希望您能找到想要的東西。
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.