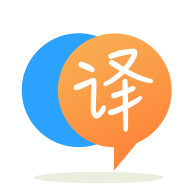
[英]How to display images from SDcard to Gallery in Android emulator
[英]Read Images From Emulator sdcard folder -Android
我嘗試在我的應用中從sdcard文件夾中讀取圖像。 但是,在平板電腦設備中,該任務沒有錯誤地完成了,它列出了Gridview文件夾中的所有圖像,而在Emulator中是BUT,只是空的Gridview列表出現了,盡管該文件夾和圖片位於模擬器sdcard上,如下圖所示!
用於創建文件夾和讀取圖片的類:
實用程序代碼:
package com.example.imageslider.helper;
import java.io.File;
import java.io.FileOutputStream;
import java.io.OutputStream;
import java.util.ArrayList;
import java.util.Locale;
import android.annotation.SuppressLint;
import android.app.AlertDialog;
import android.app.ProgressDialog;
import android.content.Context;
import android.content.Intent;
import android.database.Cursor;
import android.graphics.Bitmap;
import android.graphics.Point;
import android.graphics.drawable.BitmapDrawable;
import android.graphics.drawable.Drawable;
import android.os.AsyncTask;
import android.os.Environment;
import android.view.Display;
import android.view.WindowManager;
import android.widget.Toast;
import com.example.classes.DBItems;
import com.example.classes.ProjectEngine;
import com.example.diwansalesorder.MainScreen;
import com.example.diwansalesorder.R;
import com.example.imageslider.GridViewActivity;
public class Utils {
private final Context _context;
ArrayList<String> allData = new ArrayList<String>();
// Progress Dialog
private ProgressDialog pDialog;
ArrayList<String> filePaths;
File sdCard, directory;
File[] listFiles;
// constructor
public Utils(Context context) {
this._context = context;
}
/*
* Reading file paths from SDCard
*/
public ArrayList<String> getFilePaths() {
filePaths = new ArrayList<String>();
boolean success = true; // to check if directory created ot not
readAllItems();
sdCard = Environment.getExternalStorageDirectory();
directory = new File(sdCard.getAbsolutePath() + "/DiwanAppPictures");
if (!directory.exists()) {
success = directory.mkdirs();
}
if (success) {
// check for directory
if (directory.isDirectory()) {
// getting list of file paths
listFiles = directory.listFiles();
// Check for count
if (listFiles.length > 0) {
new LoadAllPictures().execute();
} else {
new LoadAllPictures2().execute();
}
} else {
AlertDialog.Builder alert = new AlertDialog.Builder(_context);
alert.setTitle("Error!");
alert.setMessage(AppConstant.PHOTO_ALBUM
+ " directory path is not valid! Please set the image directory name AppConstant.java class");
alert.setPositiveButton("OK", null);
alert.show();
}
} else {
Toast.makeText(_context,
" can't create " + AppConstant.PHOTO_ALBUM + " folder !!",
Toast.LENGTH_LONG).show();
Intent prevIntent = new Intent(_context, MainScreen.class);
_context.startActivity(prevIntent);
}
return filePaths;
}
/*
* Check supported file extensions
*
* @returns boolean
*/
private boolean IsSupportedFile(String filePath) {
String ext = filePath.substring((filePath.lastIndexOf(".") + 1),
filePath.length());
if (AppConstant.FILE_EXTN
.contains(ext.toLowerCase(Locale.getDefault())))
return true;
else
return false;
}
/*
* getting screen width
*/
@SuppressLint("NewApi")
public int getScreenWidth() {
int columnWidth;
WindowManager wm = (WindowManager) _context
.getSystemService(Context.WINDOW_SERVICE);
Display display = wm.getDefaultDisplay();
final Point point = new Point();
try {
display.getSize(point);
} catch (java.lang.NoSuchMethodError ignore) { // Older device
point.x = display.getWidth();
point.y = display.getHeight();
}
columnWidth = point.x;
return columnWidth;
}
// Function to read all items from data base
private void readAllItems() {
final DBItems dbI = new DBItems(_context);
try {
dbI.open();
Cursor allTitles = dbI.getAllAccounts();
allTitles.moveToFirst();
do {
String Item = allTitles.getString(1);
String Name = allTitles.getString(2);
String Cprice = allTitles.getString(3);
String Ccurr = allTitles.getString(4);
String Sprice = allTitles.getString(5);
String Scurr = allTitles.getString(6);
String Qnty = allTitles.getString(7);
String IncVat = allTitles.getString(8);
String StQnty = allTitles.getString(9);
String MinQnty = allTitles.getString(10);
String MaxQnty = allTitles.getString(11);
String Class = allTitles.getString(12);
String StkLoc = allTitles.getString(13);
String currQnty = allTitles.getString(14);
String VatDate = allTitles.getString(15);
String Ttime = allTitles.getString(16);
allData.add(Item + "!" + Name + "!" + Cprice + "!" + Ccurr
+ "!" + Sprice + "!" + Scurr + "!" + Qnty + "!"
+ IncVat + "!" + StQnty + "!" + MinQnty + "!" + MaxQnty
+ "!" + Class + "!" + StkLoc + "!" + currQnty + "!"
+ VatDate + "!" + Ttime + "");
} while (allTitles.moveToNext());
dbI.close();
} catch (Exception e) {
e.printStackTrace();
dbI.close();
}
}
/**
* Background Async Task to Load all product by making HTTP Request
* */
class LoadAllPictures2 extends AsyncTask<String, String, String> {
/**
* Before starting background thread Show Progress Dialog
* */
@Override
protected void onPreExecute() {
super.onPreExecute();
pDialog = new ProgressDialog(_context);
pDialog.setMessage(ProjectEngine.fillDataMsg);
pDialog.setIndeterminate(false);
pDialog.setCancelable(false);
pDialog.show();
}
/**
* getting All products from url
* */
@Override
protected String doInBackground(String... args) {
// Load Image from res
try {
for (int i = 0; i < allData.size(); i++) {
OutputStream fOut = null;
File file = new File(directory,
allData.get(i).split("!")[0].toString() + ".jpeg");
if (file.exists())
file.delete();
file.createNewFile();
fOut = new FileOutputStream(file);
Drawable ditemp = _context.getResources().getDrawable(
R.drawable.nopicture);
Bitmap image = ((BitmapDrawable) ditemp).getBitmap();
image.compress(Bitmap.CompressFormat.JPEG, 100, fOut);
fOut.flush();
fOut.close();
}
} catch (Exception e) {
// Log.e("saveToExternalStorage()", e.getMessage());
}
return null;
}
/**
* After completing background task Dismiss the progress dialog
* **/
@Override
protected void onPostExecute(String file_url) {
try {
Thread.sleep(1000);
} catch (InterruptedException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
Intent prevIntent = new Intent(_context, GridViewActivity.class);
_context.startActivity(prevIntent);
pDialog.dismiss();
}
}
/**
* Background Async Task to Load all product by making HTTP Request
* */
class LoadAllPictures extends AsyncTask<String, String, String> {
/**
* Before starting background thread Show Progress Dialog
* */
@Override
protected void onPreExecute() {
super.onPreExecute();
pDialog = new ProgressDialog(_context);
pDialog.setMessage(ProjectEngine.fillDataMsg);
pDialog.setIndeterminate(false);
pDialog.setCancelable(false);
pDialog.show();
}
/**
* getting All products from url
* */
@Override
protected String doInBackground(String... args) {
// loop through all files
for (int i = 0; i < listFiles.length; i++) {
// get file path
String filePath = listFiles[i].getAbsolutePath();
// check for supported file extension
if (IsSupportedFile(filePath)) {
// Add image path to array list
filePaths.add(filePath);
}
}
return null;
}
/**
* After completing background task Dismiss the progress dialog
* **/
@Override
protected void onPostExecute(String file_url) {
pDialog.dismiss();
}
}
}
請任何幫助,
最有可能是存放圖片的目錄的路徑有問題。 請比較手機上的Environment.getExternalStorageDirectory()方法返回的值與模擬器上返回的值。 它們可能有所不同,因此您可能需要相應地移動圖像。
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.