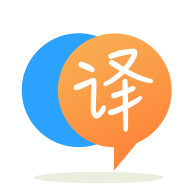
[英]Getting user's current location coordinates: MKMapItem vs CLLocationManager
[英]Get User's Current Location / Coordinates
如何存儲用戶的當前位置並在 map 上顯示位置?
我能夠在 map 上顯示預定義的坐標,我只是不知道如何從設備接收信息。
我也知道我必須將一些項目添加到 Plist 中。 我怎樣才能做到這一點?
要獲取用戶的當前位置,您需要聲明:
let locationManager = CLLocationManager()
在viewDidLoad()
您必須實例化CLLocationManager
類,如下所示:
// Ask for Authorisation from the User.
self.locationManager.requestAlwaysAuthorization()
// For use in foreground
self.locationManager.requestWhenInUseAuthorization()
if CLLocationManager.locationServicesEnabled() {
locationManager.delegate = self
locationManager.desiredAccuracy = kCLLocationAccuracyNearestTenMeters
locationManager.startUpdatingLocation()
}
然后在 CLLocationManagerDelegate 方法中,您可以獲得用戶的當前位置坐標:
func locationManager(_ manager: CLLocationManager, didUpdateLocations locations: [CLLocation]) {
guard let locValue: CLLocationCoordinate2D = manager.location?.coordinate else { return }
print("locations = \(locValue.latitude) \(locValue.longitude)")
}
在 info.plist 中,您必須添加NSLocationAlwaysUsageDescription
和您的自定義警報消息,例如; AppName(Demo App) 想使用您當前的位置。
你應該做這些步驟:
CoreLocation.framework
添加到 BuildPhases -> Link Binary With Libraries(從 XCode 7.2.1 開始不再需要)CoreLocation
導入您的類 - 很可能是 ViewController.swiftCLLocationManagerDelegate
添加到您的類聲明中NSLocationWhenInUseUsageDescription
和NSLocationAlwaysUsageDescription
添加到 plist初始化位置管理器:
locationManager = CLLocationManager() locationManager.delegate = self; locationManager.desiredAccuracy = kCLLocationAccuracyBest locationManager.requestAlwaysAuthorization() locationManager.startUpdatingLocation()
通過以下方式獲取用戶位置:
func locationManager(_ manager: CLLocationManager, didUpdateLocations locations: [CLLocation]) { let locValue:CLLocationCoordinate2D = manager.location!.coordinate print("locations = \\(locValue.latitude) \\(locValue.longitude)") }
使用Swift 5更新iOS 12.2
您必須在 plist 文件中添加以下隱私權限
<key>NSLocationWhenInUseUsageDescription</key>
<string>Description</string>
<key>NSLocationAlwaysAndWhenInUseUsageDescription</key>
<string>Description</string>
<key>NSLocationAlwaysUsageDescription</key>
<string>Description</string>
這就是我的樣子
獲取當前位置並在 Swift 2.0 中的地圖上顯示
確保您已將CoreLocation和MapKit框架添加到您的項目中(XCode 7.2.1 不需要)
import Foundation
import CoreLocation
import MapKit
class DiscoverViewController : UIViewController, CLLocationManagerDelegate {
@IBOutlet weak var map: MKMapView!
var locationManager: CLLocationManager!
override func viewDidLoad()
{
super.viewDidLoad()
if (CLLocationManager.locationServicesEnabled())
{
locationManager = CLLocationManager()
locationManager.delegate = self
locationManager.desiredAccuracy = kCLLocationAccuracyBest
locationManager.requestAlwaysAuthorization()
locationManager.startUpdatingLocation()
}
}
func locationManager(manager: CLLocationManager, didUpdateLocations locations: [CLLocation])
{
let location = locations.last! as CLLocation
let center = CLLocationCoordinate2D(latitude: location.coordinate.latitude, longitude: location.coordinate.longitude)
let region = MKCoordinateRegion(center: center, span: MKCoordinateSpan(latitudeDelta: 0.01, longitudeDelta: 0.01))
self.map.setRegion(region, animated: true)
}
}
這是結果屏幕
導入庫如:
import CoreLocation
設置委托:
CLLocationManagerDelegate
取變量,如:
var locationManager:CLLocationManager!
在 viewDidLoad() 上寫下這段漂亮的代碼:
locationManager = CLLocationManager()
locationManager.delegate = self
locationManager.desiredAccuracy = kCLLocationAccuracyBest
locationManager.requestAlwaysAuthorization()
if CLLocationManager.locationServicesEnabled(){
locationManager.startUpdatingLocation()
}
編寫 CLLocation 委托方法:
//MARK: - location delegate methods
func locationManager(_ manager: CLLocationManager, didUpdateLocations locations: [CLLocation]) {
let userLocation :CLLocation = locations[0] as CLLocation
print("user latitude = \(userLocation.coordinate.latitude)")
print("user longitude = \(userLocation.coordinate.longitude)")
self.labelLat.text = "\(userLocation.coordinate.latitude)"
self.labelLongi.text = "\(userLocation.coordinate.longitude)"
let geocoder = CLGeocoder()
geocoder.reverseGeocodeLocation(userLocation) { (placemarks, error) in
if (error != nil){
print("error in reverseGeocode")
}
let placemark = placemarks! as [CLPlacemark]
if placemark.count>0{
let placemark = placemarks![0]
print(placemark.locality!)
print(placemark.administrativeArea!)
print(placemark.country!)
self.labelAdd.text = "\(placemark.locality!), \(placemark.administrativeArea!), \(placemark.country!)"
}
}
}
func locationManager(_ manager: CLLocationManager, didFailWithError error: Error) {
print("Error \(error)")
}
現在設置訪問該位置的權限,因此將這些鍵值添加到您的info.plist文件中
<key>NSLocationAlwaysUsageDescription</key>
<string>Will you allow this app to always know your location?</string>
<key>NSLocationWhenInUseUsageDescription</key>
<string>Do you allow this app to know your current location?</string>
<key>NSLocationAlwaysAndWhenInUseUsageDescription</key>
<string>Do you allow this app to know your current location?</string>
100% 工作沒有任何問題。 已測試
NSLocationWhenInUseUsageDescription = 當應用程序在后台時請求使用位置服務的權限。 在你的 plist 文件中。
如果這有效,那么請投票給答案。
首先導入 Corelocation 和 MapKit 庫:
import MapKit
import CoreLocation
從 CLLocationManagerDelegate 繼承到我們的類
class ViewController: UIViewController, CLLocationManagerDelegate
創建一個 locationManager 變量,這將是您的位置數據
var locationManager = CLLocationManager()
創建一個函數來獲取位置信息,具體來說這個確切的語法有效:
func locationManager(manager: CLLocationManager, didUpdateLocations locations: [CLLocation]) {
在您的函數中為用戶當前位置創建一個常量
let userLocation:CLLocation = locations[0] as CLLocation // note that locations is same as the one in the function declaration
停止更新位置,這可以防止您的設備在移動時不斷更改窗口以將您的位置居中(如果您希望它以其他方式運行,則可以省略此項)
manager.stopUpdatingLocation()
從您剛剛定義的 userLocatin 獲取用戶坐標:
let coordinations = CLLocationCoordinate2D(latitude: userLocation.coordinate.latitude,longitude: userLocation.coordinate.longitude)
定義您希望地圖的縮放程度:
let span = MKCoordinateSpanMake(0.2,0.2)
將這兩者結合起來得到區域:
let region = MKCoordinateRegion(center: coordinations, span: span)//this basically tells your map where to look and where from what distance
現在設置區域並選擇是否要它帶動畫去那里
mapView.setRegion(region, animated: true)
關閉你的功能}
從您的按鈕或您想將 locationManagerDeleget 設置為 self 的其他方式
現在允許顯示位置
指定准確度
locationManager.desiredAccuracy = kCLLocationAccuracyBest
授權:
locationManager.requestWhenInUseAuthorization()
為了能夠授權定位服務,您需要將這兩行添加到您的 plist
獲取位置:
locationManager.startUpdatingLocation()
顯示給用戶:
mapView.showsUserLocation = true
這是我的完整代碼:
import UIKit
import MapKit
import CoreLocation
class ViewController: UIViewController, CLLocationManagerDelegate {
@IBOutlet weak var mapView: MKMapView!
var locationManager = CLLocationManager()
override func viewDidLoad() {
super.viewDidLoad()
// Do any additional setup after loading the view, typically from a nib.
}
override func didReceiveMemoryWarning() {
super.didReceiveMemoryWarning()
// Dispose of any resources that can be recreated.
}
@IBAction func locateMe(sender: UIBarButtonItem) {
locationManager.delegate = self
locationManager.desiredAccuracy = kCLLocationAccuracyBest
locationManager.requestWhenInUseAuthorization()
locationManager.startUpdatingLocation()
mapView.showsUserLocation = true
}
func locationManager(manager: CLLocationManager, didUpdateLocations locations: [CLLocation]) {
let userLocation:CLLocation = locations[0] as CLLocation
manager.stopUpdatingLocation()
let coordinations = CLLocationCoordinate2D(latitude: userLocation.coordinate.latitude,longitude: userLocation.coordinate.longitude)
let span = MKCoordinateSpanMake(0.2,0.2)
let region = MKCoordinateRegion(center: coordinations, span: span)
mapView.setRegion(region, animated: true)
}
}
斯威夫特 3.0
如果您不想在地圖中顯示用戶位置,而只想將其存儲在 firebase 或其他地方,請按照以下步驟操作,
import MapKit
import CoreLocation
現在在您的 VC 上使用 CLLocationManagerDelegate,您必須覆蓋下面顯示的最后三個方法。 您可以看到 requestLocation() 方法如何使用這些方法為您獲取當前用戶位置。
class MyVc: UIViewController, CLLocationManagerDelegate {
let locationManager = CLLocationManager()
override func viewDidLoad() {
super.viewDidLoad()
isAuthorizedtoGetUserLocation()
if CLLocationManager.locationServicesEnabled() {
locationManager.delegate = self
locationManager.desiredAccuracy = kCLLocationAccuracyNearestTenMeters
}
}
//if we have no permission to access user location, then ask user for permission.
func isAuthorizedtoGetUserLocation() {
if CLLocationManager.authorizationStatus() != .authorizedWhenInUse {
locationManager.requestWhenInUseAuthorization()
}
}
//this method will be called each time when a user change his location access preference.
func locationManager(_ manager: CLLocationManager, didChangeAuthorization status: CLAuthorizationStatus) {
if status == .authorizedWhenInUse {
print("User allowed us to access location")
//do whatever init activities here.
}
}
//this method is called by the framework on locationManager.requestLocation();
func locationManager(_ manager: CLLocationManager, didUpdateLocations locations: [CLLocation]) {
print("Did location updates is called")
//store the user location here to firebase or somewhere
}
func locationManager(_ manager: CLLocationManager, didFailWithError error: Error) {
print("Did location updates is called but failed getting location \(error)")
}
}
現在,一旦用戶登錄到您的應用程序,您就可以對以下調用進行編碼。 當 requestLocation() 被調用時,它將進一步調用上面的 didUpdateLocations,您可以將位置存儲到 Firebase 或其他任何地方。
if CLLocationManager.locationServicesEnabled() {
locationManager.requestLocation();
}
如果您使用的是 GeoFire,那么在上面的 didUpdateLocations 方法中,您可以將位置存儲如下
geoFire?.setLocation(locations.first, forKey: uid) where uid is the user id who logged in to the app. I think you will know how to get UID based on your app sign in implementation.
最后但並非最不重要的一點是,轉到您的 Info.plist 並啟用“使用使用說明時的隱私 - 位置”。
當您使用模擬器進行測試時,它始終為您提供一個您在模擬器 -> 調試 -> 位置中配置的自定義位置。
首先在你的項目中添加兩個框架
1:地圖套件
2:核心定位(從 XCode 7.2.1 開始不再需要)
在你的班級中定義
var manager:CLLocationManager!
var myLocations: [CLLocation] = []
然后在 viewDidLoad 方法中編碼這個
manager = CLLocationManager()
manager.desiredAccuracy = kCLLocationAccuracyBest
manager.requestAlwaysAuthorization()
manager.startUpdatingLocation()
//Setup our Map View
mapobj.showsUserLocation = true
不要忘記在 plist 文件中添加這兩個值
1: NSLocationWhenInUseUsageDescription
2: NSLocationAlwaysUsageDescription
用法:
在類中定義字段
let getLocation = GetLocation()
通過簡單的代碼在類的函數中使用:
getLocation.run {
if let location = $0 {
print("location = \(location.coordinate.latitude) \(location.coordinate.longitude)")
} else {
print("Get Location failed \(getLocation.didFailWithError)")
}
}
班級:
import CoreLocation
public class GetLocation: NSObject, CLLocationManagerDelegate {
let manager = CLLocationManager()
var locationCallback: ((CLLocation?) -> Void)!
var locationServicesEnabled = false
var didFailWithError: Error?
public func run(callback: @escaping (CLLocation?) -> Void) {
locationCallback = callback
manager.delegate = self
manager.desiredAccuracy = kCLLocationAccuracyBestForNavigation
manager.requestWhenInUseAuthorization()
locationServicesEnabled = CLLocationManager.locationServicesEnabled()
if locationServicesEnabled { manager.startUpdatingLocation() }
else { locationCallback(nil) }
}
public func locationManager(_ manager: CLLocationManager,
didUpdateLocations locations: [CLLocation]) {
locationCallback(locations.last!)
manager.stopUpdatingLocation()
}
public func locationManager(_ manager: CLLocationManager, didFailWithError error: Error) {
didFailWithError = error
locationCallback(nil)
manager.stopUpdatingLocation()
}
deinit {
manager.stopUpdatingLocation()
}
}
不要忘記在 info.plist 中添加“NSLocationWhenInUseUsageDescription”。
import CoreLocation
import UIKit
class ViewController: UIViewController, CLLocationManagerDelegate {
var locationManager: CLLocationManager!
override func viewDidLoad() {
super.viewDidLoad()
locationManager = CLLocationManager()
locationManager.delegate = self
locationManager.requestWhenInUseAuthorization()
}
func locationManager(_ manager: CLLocationManager, didChangeAuthorization status: CLAuthorizationStatus) {
if status != .authorizedWhenInUse {return}
locationManager.desiredAccuracy = kCLLocationAccuracyBest
locationManager.startUpdatingLocation()
let locValue: CLLocationCoordinate2D = manager.location!.coordinate
print("locations = \(locValue.latitude) \(locValue.longitude)")
}
}
由於對requestWhenInUseAuthorization
的調用是異步的,因此應用在用戶授予權限或拒絕權限后調用locationManager
函數。 因此,在用戶授予權限的情況下,將您的位置獲取代碼放置在該函數中是合適的。 這是我在上面找到的最好的教程。
override func viewDidLoad() {
super.viewDidLoad()
locationManager.requestWhenInUseAuthorization();
if CLLocationManager.locationServicesEnabled() {
locationManager.delegate = self
locationManager.desiredAccuracy = kCLLocationAccuracyNearestTenMeters
locationManager.startUpdatingLocation()
}
else{
print("Location service disabled");
}
}
這是您的視圖確實加載方法,並且在 ViewController 類中還包括 mapStart 更新方法,如下所示
func locationManager(manager: CLLocationManager!, didUpdateLocations locations: [AnyObject]!) {
var locValue : CLLocationCoordinate2D = manager.location.coordinate;
let span2 = MKCoordinateSpanMake(1, 1)
let long = locValue.longitude;
let lat = locValue.latitude;
print(long);
print(lat);
let loadlocation = CLLocationCoordinate2D(
latitude: lat, longitude: long
)
mapView.centerCoordinate = loadlocation;
locationManager.stopUpdatingLocation();
}
另外不要忘記在您的項目中添加 CoreLocation.FrameWork 和 MapKit.Framework (從XCode 7.2.1 開始不再需要)
import Foundation
import CoreLocation
enum Result<T> {
case success(T)
case failure(Error)
}
final class LocationService: NSObject {
private let manager: CLLocationManager
init(manager: CLLocationManager = .init()) {
self.manager = manager
super.init()
manager.delegate = self
}
var newLocation: ((Result<CLLocation>) -> Void)?
var didChangeStatus: ((Bool) -> Void)?
var status: CLAuthorizationStatus {
return CLLocationManager.authorizationStatus()
}
func requestLocationAuthorization() {
manager.delegate = self
manager.desiredAccuracy = kCLLocationAccuracyBest
manager.requestWhenInUseAuthorization()
if CLLocationManager.locationServicesEnabled() {
manager.startUpdatingLocation()
//locationManager.startUpdatingHeading()
}
}
func getLocation() {
manager.requestLocation()
}
deinit {
manager.stopUpdatingLocation()
}
}
extension LocationService: CLLocationManagerDelegate {
func locationManager(_ manager: CLLocationManager, didFailWithError error: Error) {
newLocation?(.failure(error))
manager.stopUpdatingLocation()
}
func locationManager(_ manager: CLLocationManager, didUpdateLocations locations: [CLLocation]) {
if let location = locations.sorted(by: {$0.timestamp > $1.timestamp}).first {
newLocation?(.success(location))
}
manager.stopUpdatingLocation()
}
func locationManager(_ manager: CLLocationManager, didChangeAuthorization status: CLAuthorizationStatus) {
switch status {
case .notDetermined, .restricted, .denied:
didChangeStatus?(false)
default:
didChangeStatus?(true)
}
}
}
需要在所需的 ViewController 中編寫此代碼。
//NOTE:: Add permission in info.plist::: NSLocationWhenInUseUsageDescription
let locationService = LocationService()
@IBAction func action_AllowButtonTapped(_ sender: Any) {
didTapAllow()
}
func didTapAllow() {
locationService.requestLocationAuthorization()
}
func getCurrentLocationCoordinates(){
locationService.newLocation = {result in
switch result {
case .success(let location):
print(location.coordinate.latitude, location.coordinate.longitude)
case .failure(let error):
assertionFailure("Error getting the users location \(error)")
}
}
}
func getCurrentLocationCoordinates() {
locationService.newLocation = { result in
switch result {
case .success(let location):
print(location.coordinate.latitude, location.coordinate.longitude)
CLGeocoder().reverseGeocodeLocation(location, completionHandler: {(placemarks, error) -> Void in
if error != nil {
print("Reverse geocoder failed with error" + (error?.localizedDescription)!)
return
}
if (placemarks?.count)! > 0 {
print("placemarks", placemarks!)
let pmark = placemarks?[0]
self.displayLocationInfo(pmark)
} else {
print("Problem with the data received from geocoder")
}
})
case .failure(let error):
assertionFailure("Error getting the users location \(error)")
}
}
}
這是一個對我有用的復制粘貼示例。
http://swiftdeveloperblog.com/code-examples/determine-users-current-location-example-in-swift/
import UIKit
import CoreLocation
class ViewController: UIViewController, CLLocationManagerDelegate {
var locationManager:CLLocationManager!
override func viewDidLoad() {
super.viewDidLoad()
// Do any additional setup after loading the view, typically from a nib.
}
override func didReceiveMemoryWarning() {
super.didReceiveMemoryWarning()
// Dispose of any resources that can be recreated.
}
override func viewWillAppear(_ animated: Bool) {
super.viewWillAppear(animated)
determineMyCurrentLocation()
}
func determineMyCurrentLocation() {
locationManager = CLLocationManager()
locationManager.delegate = self
locationManager.desiredAccuracy = kCLLocationAccuracyBest
locationManager.requestAlwaysAuthorization()
if CLLocationManager.locationServicesEnabled() {
locationManager.startUpdatingLocation()
//locationManager.startUpdatingHeading()
}
}
func locationManager(_ manager: CLLocationManager, didUpdateLocations locations: [CLLocation]) {
let userLocation:CLLocation = locations[0] as CLLocation
// Call stopUpdatingLocation() to stop listening for location updates,
// other wise this function will be called every time when user location changes.
// manager.stopUpdatingLocation()
print("user latitude = \(userLocation.coordinate.latitude)")
print("user longitude = \(userLocation.coordinate.longitude)")
}
func locationManager(_ manager: CLLocationManager, didFailWithError error: Error)
{
print("Error \(error)")
}
}
以前的所有答案都沒有真正正確地遵循正確的步驟集。 設置CLLocationManager
時,您最初不需要調用CLLocationManager.locationServicesEnabled()
或locationManager.requestWhenInUseAuthorization()
。 在您確認您擁有授權之前,您不應該調用startUpdatingLocation()
。
要開始,請添加所需的隱私設置(如果尚未完成)。 Select 您的應用程序目標和 go 到“信息”選項卡。 在“自定義 iOS 目標屬性”部分下,右鍵單擊 select“添加行”。 向下滾動到“隱私”條目和 select:
根據您的應用程序的需要。 對於該值,請務必輸入一個句子,向用戶解釋為什么您的應用需要訪問他們的位置。 如果給定的描述對用戶沒有用,您的應用可能會被 Apple 拒絕。
然后使用以下(大量注釋)代碼作為獲取用戶在視圖 controller 中的位置的示例:
import UIKit
import CoreLocation
class ViewController: UIViewController {
var locationManager: CLLocationManager!
override func viewDidLoad() {
super.viewDidLoad()
// Setup the location manager
locationManager = CLLocationManager()
locationManager.delegate = self
locationManager.desiredAccuracy = kCLLocationAccuracyBestForNavigation // Or other desired accuracy
// That's it for the initial setup. Everything else is handled in the
// locationManagerDidChangeAuthorization delegate method.
}
}
extension ViewController: CLLocationManagerDelegate {
// This is called as soon as the location manager is setup (in viewDidLoad)
// This is called when the user responds to the privacy dialog
// This is called if the user changes the privacy setting in the Settings app
func locationManagerDidChangeAuthorization(_ manager: CLLocationManager) {
switch manager.authorizationStatus {
case .notDetermined:
// Request the appropriate authorization based on the needs of the app
manager.requestWhenInUseAuthorization()
// manager.requestAlwaysAuthorization()
case .restricted:
print("Sorry, restricted")
// Optional: Offer to take user to app's settings screen
case .denied:
print("Sorry, denied")
// Optional: Offer to take user to app's settings screen
case .authorizedAlways, .authorizedWhenInUse:
// The app has permission so start getting location updates
manager.startUpdatingLocation()
@unknown default:
print("Unknown status")
}
}
func locationManager(_ manager: CLLocationManager, didUpdateLocations locations: [CLLocation]) {
print("Received \(locations.count) locations")
// Some location updates can be invalid or have insufficient accuracy.
// Find the first location that has sufficient horizontal accuracy.
// If the manager's desiredAccuracy is one of kCLLocationAccuracyNearestTenMeters,
// kCLLocationAccuracyHundredMeters, kCLLocationAccuracyKilometer, or kCLLocationAccuracyThreeKilometers
// then you can use $0.horizontalAccuracy <= manager.desiredAccuracy. Otherwise enter the number of meters desired.
if let location = locations.first(where: { $0.horizontalAccuracy <= 40 }) {
print("Good location found: \(location)")
// Call the following if you don't need any more updates
manager.stopUpdatingLocation()
// Do something useful with the found location
// - show on a map
// - Reverse geocode to get the address
// - send to server
}
}
func locationManager(_ manager: CLLocationManager, didFailWithError error: Error) {
print("Location manager error: \(error)")
}
}
// its with strongboard
@IBOutlet weak var mapView: MKMapView!
//12.9767415,77.6903967 - exact location latitude n longitude location
let cooridinate = CLLocationCoordinate2D(latitude: 12.9767415 , longitude: 77.6903967)
let spanDegree = MKCoordinateSpan(latitudeDelta: 0.2,longitudeDelta: 0.2)
let region = MKCoordinateRegion(center: cooridinate , span: spanDegree)
mapView.setRegion(region, animated: true)
100% 在 iOS Swift 4 中工作,作者:Parmar Sajjad
第 1 步:轉到 GoogleDeveloper Api 控制台並創建您的 ApiKey
第 2 步:轉到項目安裝 Cocoapods GoogleMaps pod
第 3 步:轉到 AppDelegate.swift 導入 GoogleMaps 和
func application(_ application: UIApplication, didFinishLaunchingWithOptions launchOptions: [UIApplicationLaunchOptionsKey: Any]?) -> Bool {
// Override point for customization after application launch.
GMSServices.provideAPIKey("ApiKey")
return true
}
第 4 步:導入 UIKit 導入 GoogleMaps 類 ViewController: UIViewController, CLLocationManagerDelegate {
@IBOutlet weak var mapview: UIView!
let locationManager = CLLocationManager()
override func viewDidLoad() {
super.viewDidLoad()
locationManagerSetting()
// Do any additional setup after loading the view, typically from a nib.
}
override func didReceiveMemoryWarning() {
super.didReceiveMemoryWarning()
// Dispose of any resources that can be recreated.
}
func locationManagerSetting() {
self.locationManager.delegate = self
self.locationManager.desiredAccuracy = kCLLocationAccuracyNearestTenMeters
self.locationManager.requestWhenInUseAuthorization()
self.locationManager.startUpdatingLocation()
}
func locationManager(_ manager: CLLocationManager, didUpdateLocations locations: [CLLocation]) {
self.showCurrentLocationonMap()
self.locationManager.stopUpdatingLocation()
}
func showCurrentLocationonMap() {
let
cameraposition = GMSCameraPosition.camera(withLatitude: (self.locationManager.location?.coordinate.latitude)! , longitude: (self.locationManager.location?.coordinate.longitude)!, zoom: 18)
let mapviewposition = GMSMapView.map(withFrame: CGRect(x: 0, y: 0, width: self.mapview.frame.size.width, height: self.mapview.frame.size.height), camera: cameraposition)
mapviewposition.settings.myLocationButton = true
mapviewposition.isMyLocationEnabled = true
let marker = GMSMarker()
marker.position = cameraposition.target
marker.snippet = "Macczeb Technologies"
marker.appearAnimation = GMSMarkerAnimation.pop
marker.map = mapviewposition
self.mapview.addSubview(mapviewposition)
}
}
第 5 步:打開 info.plist 文件並在下面添加 Privacy - Location When In Use Usage Description ......在主故事板文件基本名稱下方
第 6 步:運行
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.