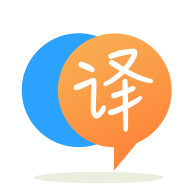
[英]Is there a PHP equivalent of JavaScript's Array.prototype.some() function
[英]What is the python equivalent of JavaScript's Array.prototype.some?
python 有任何等效於 JavaScript 的Array.prototype.some / every嗎?
簡單的 JavaScript 示例:
var arr = [ "a", "b", "c" ];
arr.some(function (element, index) {
console.log("index: " + index + ", element: " + element)
if(element === "b"){
return true;
}
});
將輸出:
index: 0, element: a
index: 1, element: b
下面的 python 似乎在功能上是等效的,但我不知道是否有更“pythonic”的方法。
arr = [ "a", "b", "c" ]
for index, element in enumerate(arr):
print("index: %i, element: %s" % (index, element))
if element == "b":
break
Python 有all(iterable)
和any(iterable)
。 因此,如果您制作了一個生成器或迭代器來滿足您的需求,您可以使用這些函數對其進行測試。 例如:
some_is_b = any(x == 'b' for x in ary)
all_are_b = all(x == 'b' for x in ary)
它們實際上在文檔中由它們的代碼等價物定義。 這看起來很熟悉嗎?
def any(iterable):
for element in iterable:
if element:
return True
return False
不。NumPy 數組有,但標准 python 列表沒有。 即便如此,numpy 數組實現並不是您所期望的:它們不采用謂詞,而是通過將每個元素轉換為布爾值來評估每個元素。
編輯: any
和all
作為函數(而不是方法)存在,但它們不應用謂詞,而是將布爾值視為 numpy 方法。
在 Python 中, some
可能是:
def some(list_, pred):
return bool([i for i in list_ if pred(i)])
#or a more efficient approach, which doesn't build a new list
def some(list_, pred):
return any(pred(i) for i in list_) #booleanize the values, and pass them to any
你可以實現every
:
def every(list_, pred):
return all(pred(i) for i in list_)
編輯:愚蠢的樣本:
every(['a', 'b', 'c'], lambda e: e == 'b')
some(['a', 'b', 'c'], lambda e: e == 'b')
自己試試
numbers = [1, 2, 3, 4, 5, 6, 7, 8]
def callback( n ):
return (n % 2 == 0)
# 1) Demonstrates how list() works with an iterable
isEvenList = list( callback(n) for n in numbers)
print(isEvenList)
# 2) Demonstrates how any() works with an iterable
anyEvenNumbers = any( callback(n) for n in numbers)
print(anyEvenNumbers)
# 3) Demonstrates how all() works with an iterable
allEvenNumbers = all( callback(n) for n in numbers)
print(allEvenNumbers)
isEvenList = list(callback(n) for n in numbers)
print(isEvenList)
#[False, True, False, True, False, True, False, True]
anyEvenNumbers = any( callback(n) for n in numbers)
print(anyEvenNumbers)
#True
allEvenNumbers = all( callback(n) for n in numbers)
print(allEvenNumbers)
#False
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.