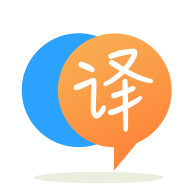
[英]How to suppress inline function used but never defined warning in g++
[英]How to use a function defined in another file with g++?
這是一個非常基本的問題,我在網上找到了很多概念性的答案,但卻未能真正實現。
這是我有的:
文件source.cc
#include <iostream>
int myfunc() {
return 42;
}
int main() {
return 0;
}
然后我通過以下方式創建一個目標文件source.o:
g++ -c source.cc
最后,我用
ar rvs source.a source.o
獲取source.a靜態庫。
現在,麻煩來了。
file user.cc看起來像這樣:
#include <iostream>
#include <source.a>
int main() {
std::cout << myfunc();
}
我顯然想要使用庫中定義的函數,但在嘗試編譯user.cc時:
g++ user.cc -o user
我得到的錯誤是:
user.cc:2:22: fatal error: source.a: No such file or directory
compilation terminated.
#include
是編譯時間,必須是包含例如此內容的C / C ++頭文件(不是庫)
extern int myfunc();
比你必須使用鏈接器編譯所有togehter(指定命令行上所需的所有文件)
不要#include
庫存檔。 它不包含源代碼。 它包含目標代碼。 將它放在鏈接器的命令行上。
用g++ -c user.cc
編譯。 鏈接g++ -o user user.o source.a
。
在C ++(和C99)中,您調用的每個函數都要提前聲明。 為此,您需要提供函數的“簽名”,而不是定義。 在你的情況下,這將是聲明
int myfunc();
它告訴編譯器myfunc
是一個不帶參數並返回int
的函數。 通常,您會在標頭中包含此函數聲明。
.a
文件是一個不包含C或C ++代碼的已編譯存檔,因此#include
-ing將其轉換為C ++文件將不起作用。 相反,您需要創建一個C或C ++標頭,並將.a
存檔添加到要鏈接到最終可執行文件的文件列表中。 你可以說,使用g ++,這很容易
g++ user.cc source.a -o executable
例如。
除了其他人已經在命令行上正確使用g ++的語法上寫的內容之外,您可能還需要考慮代碼組織的以下注釋。
在您的庫代碼 ,你不應該定義main()
函數。 main()
的定義應該是使用您的庫的代碼的一部分,即示例中的文件user.cc
此外,您可能希望向庫的客戶端分發一個頭文件 ,它們可以用來導入庫導出的函數的聲明 。
所以,考慮定義一些像這樣的文件:
頭文件:
// library.h -- Public header for your library's clients
#pragma once // or use #ifndef/#define/#endif "header guards"
// Functions exported by your library:
// their *declarations* go in the library's public header file;
// their *definitions* go in the library's implementation file(s) (.cc, .cpp)
// (exception: inline functions/methods, that are implemented in headers).
int myfunc();
// Add some other exported functions...
// NOTE: "extern" not needed in C++!
實施檔案:
// library.cc -- Library implementation code
#include "library.h" // library public header
#include <...> // headers required by this implementation code
// *Define* functions exported by the library
int myfunc() {
return 42;
}
// ...other function implementations...
然后,圖書館的客戶端將執行以下操作:
包含main()
並使用您的庫的文件:
// main.cc (or user.cc or whatever you call it)
#include <iostream> // For std::cout, std::endl
...#include any other required header file...
#include "library.h" // Your library public header file
int main() {
// Call library's function
std::cout << myfunc() << std::endl;
}
// NOTE: main() is special: "return 0;" can be omitted.
默認情況下, #include <...>
構造(帶尖括號)搜索系統目錄以查找指定的文件。 要搜索其他目錄,可以使用-L
選項,要鏈接到庫,需要在命令行中使用source.a
. 像這樣:
g++ user.cc -L/path/to/library source.a -o user
添加到討論中的幾個想法1)您應該創建一個名為source.h的頭文件,其中包含該行
int myfunc(); 或者,extern int myfunc();
2)在user.cc頂部應該是一行包含“source.h”這將告訴編譯器定義的函數
3)我認為你應該從source.cc中取出main函數,或者至少使它成為靜態函數
正如其他人所指出的那樣,你不能包含一個庫文件(source.a),你的編譯和鏈接過程應該按原樣運行。
您可以使用#include
使用十字架文件。 如果要使用庫,則必須告訴鏈接器在哪里查找庫文件。
檢查這些文章的靜態和動態庫:
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.