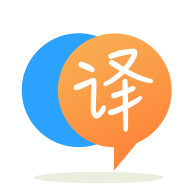
[英]Sorting a std::vector of std::pair's using user defined compare class
[英]Sorting a std::vector with a compare functor which has access to class members
我想用std :: sort和自定義比較函數/函子來整理一個向量。 在這個函數里面,我總是希望在類中定義訪問函數或變量。
Class MainClass
{
protected: // Variables
float fixedpoint;
protected: // Methods
float DistanceBetweenTwoPoints(float,float);
void function1();
struct CompareDistanceToGoal : public std::binary_function<float,float,bool>
{
bool operator()(float p1, float p2)
{
// return ( p1 < p2);
// I want to use the following code instead of the above
return DistanceBetweenTwoPoints(fixedpoint,p1) < DistanceBetweenTwoPoints(fixedpoint,p2);
}
};
}
內部功能1:
void MainClass::function1()
{
std::vector<float> V1;
std::sort(V1.begin(),V1.end(),MainClass::CompareDistanceToGoal());
}
因此,我想要訪問fixedpoint和DistanceBetweenTwoPoints()函數,而不是使用“return(p1 <p2)”。 這是可能的(即以某種方式使用朋友標識符)?
誰能告訴我怎么做? 謝謝。
作為嵌套類型, CompareDistanceToGoal
可以訪問MainClass
所有成員; 沒有必要宣布它是朋友。 (雖然這是對語言的近期適度更改;未實現C ++ 11的編譯器可能需要朋友聲明, friend CompareDistanceToGoal;
以匹配現代行為)。
但是,由於這些成員是非靜態成員,因此除非提供MainClass
對象,否則無法對這些成員執行任何操作。 也許你想讓它們變得靜止; 或者你想“捕捉”一個物體:
struct CompareDistanceToGoal // no need for that binary_function nonsense
{
MainClass & mc;
CompareDistanceToGoal(MainClass & mc) : mc(mc) {}
bool operator()(float p1, float p2)
{
return mc.DistanceBetweenTwoPoints(mc.fixedpoint,p1) <
mc.DistanceBetweenTwoPoints(mc.fixedpoint,p2);
}
};
std::sort(V1.begin(),V1.end(),MainClass::CompareDistanceToGoal(some_main_class_object));
這是一個有點難以知道你想做什么,但這似乎有被你希望為別的什么盡可能多的機會......注意, MainClass
存儲fixedpoint
,然后提供了一個仿函數(無需嵌套類然后由sort
。 在下面的代碼中,它對向量進行排序,因此最接近MainClass
fixedpoint
元素在vector
中較早。 看它在ideone.com上運行。
#include <iostream>
#include <vector>
#include <algorithm>
#include <cmath>
class MainClass
{
public:
MainClass(float fixedpoint) : fixedpoint_(fixedpoint) { }
bool operator()(float p1, float p2) const
{
float d1 = DistanceBetweenTwoPoints(fixedpoint_,p1);
float d2 = DistanceBetweenTwoPoints(fixedpoint_,p2);
return d1 < d2 || d1 == d2 && p1 < p2;
};
protected: // Variables
float fixedpoint_;
static float DistanceBetweenTwoPoints(float a,float b) { return std::fabs(a - b); }
void function1();
};
int main()
{
std::vector<float> v { 1, 3, 4.5, 2.3, 9, 12 };
std::sort(std::begin(v), std::end(v), MainClass(9.3));
for (auto f : v)
std::cout << f << '\n';
}
你可以手動捕獲價值
struct CompareDistanceToGoal : public std::binary_function<float,float,bool>
{
float fixedpoint;
CompareDistanceToGoal(float p) : fixedpoint(p) {}
bool operator()(float p1, float p2)
{
return DistanceBetweenTwoPoints(fixedpoint,p1) < DistanceBetweenTwoPoints(fixedpoint,p2);
}
};
並使用它
void MainClass::function1()
{
std::vector<float> V1;
std::sort(V1.begin(),V1.end(),MainClass::CompareDistanceToGoal(fixedpoint));
}
或者如果C ++ 11可用,請使用lambda來捕獲值
void MainClass::function1()
{
std::vector<float> V1;
std::sort(V1.begin(),V1.end(),[=](float p1, float p2){
return DistanceBetweenTwoPoints(fixedpoint,p1) < DistanceBetweenTwoPoints(fixedpoint,p2);
});
}
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.