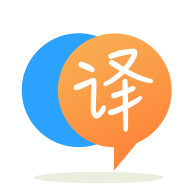
[英]Extract text between two substrings using regular expression multiline in python
[英]Regular expression, matching between two patterns in a multiline string
我有一個多行字符串,並且我想要一個正則表達式從兩個模式之間獲取一些東西。 例如,在這里我試圖匹配標題和日期之間的所有內容
例如:
s ="""\n#here's a title\n\nhello world!!!\n\nPosted on 11-09-2014 02:32:30"""
re.findall(r'#.+\n',s)[0][1:-1] # this grabs the title
Out: "here's a title"
re.findall(r'Posted on .+\n',s)[0][10:-1] #this grabs the date
Out: "11-09-2014 02:32:30"
re.findall(r'^[#\W+]',s) # try to grab everything after the title
Out: ['\n'] # but it only grabs until the end of line
>>> s = '''\n#here's a title\n\nhello world!!!\n\nPosted on 11-09-2014 02:32:30'''
>>> m1 = re.search(r'^#.+$', s, re.MULTILINE)
>>> m2 = re.search(r'^Posted on ', s, re.MULTILINE)
>>> m1.end()
16
>>> m2.start()
34
>>> s[m1.end():m2.start()]
'\n\nhello world!!!\n\n'
不要忘記檢查m1
和m2
是否不是None
。
>>> re.findall(r'\n([^#].*)Posted', s, re.S)
['\nhello world!!!\n\n']
如果要避免換行符:
>>> re.findall(r'^([^#\n].*?)\n+Posted', s, re.S + re.M)
['hello world!!!']
您可以使用一個正則表達式匹配所有內容。
>>> s = '''\n#here's a title\n\nhello world!!!\n\nPosted on 11-09-2014 02:32:30'''
>>> re.search(r'#([^\n]+)\s+([^\n]+)\s+\D+([^\n]+)', s).groups()
("here's a title", 'hello world!!!', '11-09-2014 02:32:30')
您應該使用帶括號的分組匹配:
result = re.search(r'#[^\n]+\n+(.*)\n+Posted on .*', s, re.MULTILINE | re.DOTALL)
result.group(1)
在這里,我使用了search
,但是如果同一字符串可能包含多個匹配項,您仍然可以使用findall
。
如果要捕獲標題,內容和日期,則可以使用多個組:
result = re.search(r'#([^\n]+)\n+(.*)\n+Posted on ([^\n]*)', s, re.MULTILINE | re.DOTALL)
result.group(1) # The title
result.group(2) # The contents
result.group(3) # The date
在同一個正則表達式中捕獲全部3個結果要比對每個部分使用一個正則表達式好得多,特別是如果您的多行字符串可能包含多個匹配項(在其中將各個findall
結果“同步”在一起很容易導致錯誤的title-content-date組合)。
如果您打算大量使用此正則表達式,請考慮對其進行一次編譯以提高性能:
regex = re.compile(r'#([^\n]+)\n+(.*)\n+(Posted on [^\n]*)', re.MULTILINE | re.DOTALL)
# ...
result = regex.search(s)
result = regex.search('another multiline string, ...')
將組匹配與非貪婪搜索(。*?)一起使用。 並給組起一個名稱以便於查找。
>>> s = '\n#here\'s a title\n\nhello world!!!\n\nPosted on 11-09-2014 02:32:30'
>>> pattern = r'\s*#[\w \']+\n+(?P<content>.*?)\n+Posted on'
>>> a = re.match(pattern, s, re.M)
>>> a.group('content')
'hello world!!!'
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.