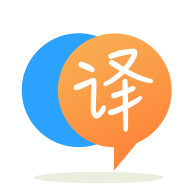
[英]Unique paths from top-left corner to bottom-right corner of grid by getting input
[英]hello,I would like to move my satellite menu from top right corner to the bottom left corner of the screen
我有2個Java文件,這是第一個叫做ArcLayout的文件
public class ArcLayout extends ViewGroup {
/**
* children will be set the same size.
*/
private int mChildSize;
private int mChildPadding = 5;
private int mLayoutPadding = 10;
public static final float DEFAULT_FROM_DEGREES = 270.0f;
public static final float DEFAULT_TO_DEGREES = 360.0f;
private float mFromDegrees = DEFAULT_FROM_DEGREES;
private float mToDegrees = DEFAULT_TO_DEGREES;
private static final int MIN_RADIUS = 100;
/* the distance between the layout's center and any child's center */
private int mRadius;
private boolean mExpanded = false;
public ArcLayout(Context context) {
super(context);
}
public ArcLayout(Context context, AttributeSet attrs) {
super(context, attrs);
if (attrs != null) {
TypedArray a = getContext().obtainStyledAttributes(attrs, R.styleable.ArcLayout, 0, 0);
mFromDegrees = a.getFloat(R.styleable.ArcLayout_fromDegrees, DEFAULT_FROM_DEGREES);
mToDegrees = a.getFloat(R.styleable.ArcLayout_toDegrees, DEFAULT_TO_DEGREES);
mChildSize = Math.max(a.getDimensionPixelSize(R.styleable.ArcLayout_childSize, 0), 0);
a.recycle();
}
}
private static int computeRadius(final float arcDegrees, final int childCount, final int childSize,
final int childPadding, final int minRadius) {
if (childCount < 2) {
return minRadius;
}
final float perDegrees = arcDegrees / (childCount - 1);
final float perHalfDegrees = perDegrees / 2;
final int perSize = childSize + childPadding;
final int radius = (int) ((perSize / 2) / Math.sin(Math.toRadians(perHalfDegrees)));
return Math.max(radius, minRadius);
}
private static Rect computeChildFrame(final int centerX, final int centerY, final int radius, final float degrees,
final int size) {
final double childCenterX = centerX + radius * Math.cos(Math.toRadians(degrees));
final double childCenterY = centerY + radius * Math.sin(Math.toRadians(degrees));
return new Rect((int) (childCenterX - size / 2), (int) (childCenterY - size / 2),
(int) (childCenterX + size / 2), (int) (childCenterY + size / 2));
}
@Override
protected void onMeasure(int widthMeasureSpec, int heightMeasureSpec) {
final int radius = mRadius = computeRadius(Math.abs(mToDegrees - mFromDegrees), getChildCount(), mChildSize,
mChildPadding, MIN_RADIUS);
final int size = radius * 2 + mChildSize + mChildPadding + mLayoutPadding * 2;
setMeasuredDimension(size, size);
final int count = getChildCount();
for (int i = 0; i < count; i++) {
getChildAt(i).measure(MeasureSpec.makeMeasureSpec(mChildSize, MeasureSpec.EXACTLY),
MeasureSpec.makeMeasureSpec(mChildSize, MeasureSpec.EXACTLY));
}
}
@Override
protected void onLayout(boolean changed, int l, int t, int r, int b) {
final int centerX = getWidth() / 2;
final int centerY = getHeight() / 2;
final int radius = mExpanded ? mRadius : 0;
final int childCount = getChildCount();
final float perDegrees = (mToDegrees - mFromDegrees) / (childCount - 1);
float degrees = mFromDegrees;
for (int i = 0; i < childCount; i++) {
Rect frame = computeChildFrame(centerX, centerY, radius, degrees, mChildSize);
degrees += perDegrees;
getChildAt(i).layout(frame.left, frame.top, frame.right, frame.bottom);
}
}
/**
* refers to {@link LayoutAnimationController#getDelayForView(View view)}
*/
private static long computeStartOffset(final int childCount, final boolean expanded, final int index,
final float delayPercent, final long duration, Interpolator interpolator) {
final float delay = delayPercent * duration;
final long viewDelay = (long) (getTransformedIndex(expanded, childCount, index) * delay);
final float totalDelay = delay * childCount;
float normalizedDelay = viewDelay / totalDelay;
normalizedDelay = interpolator.getInterpolation(normalizedDelay);
return (long) (normalizedDelay * totalDelay);
}
private static int getTransformedIndex(final boolean expanded, final int count, final int index) {
if (expanded) {
return count - 1 - index;
}
return index;
}
private static Animation createExpandAnimation(float fromXDelta, float toXDelta, float fromYDelta, float toYDelta,
long startOffset, long duration, Interpolator interpolator) {
Animation animation = new RotateAndTranslateAnimation(0, toXDelta, 0, toYDelta, 0, 720);
animation.setStartOffset(startOffset);
animation.setDuration(duration);
animation.setInterpolator(interpolator);
animation.setFillAfter(true);
return animation;
}
private static Animation createShrinkAnimation(float fromXDelta, float toXDelta, float fromYDelta, float toYDelta,
long startOffset, long duration, Interpolator interpolator) {
AnimationSet animationSet = new AnimationSet(false);
animationSet.setFillAfter(true);
final long preDuration = duration / 2;
Animation rotateAnimation = new RotateAnimation(0, 360, Animation.RELATIVE_TO_SELF, 0.5f,
Animation.RELATIVE_TO_SELF, 0.5f);
rotateAnimation.setStartOffset(startOffset);
rotateAnimation.setDuration(preDuration);
rotateAnimation.setInterpolator(new LinearInterpolator());
rotateAnimation.setFillAfter(true);
animationSet.addAnimation(rotateAnimation);
Animation translateAnimation = new RotateAndTranslateAnimation(0, toXDelta, 0, toYDelta, 360, 720);
translateAnimation.setStartOffset(startOffset + preDuration);
translateAnimation.setDuration(duration - preDuration);
translateAnimation.setInterpolator(interpolator);
translateAnimation.setFillAfter(true);
animationSet.addAnimation(translateAnimation);
return animationSet;
}
private void bindChildAnimation(final View child, final int index, final long duration) {
final boolean expanded = mExpanded;
final int centerX = getWidth() / 2;
final int centerY = getHeight() / 2;
final int radius = expanded ? 0 : mRadius;
final int childCount = getChildCount();
final float perDegrees = (mToDegrees - mFromDegrees) / (childCount - 1);
Rect frame = computeChildFrame(centerX, centerY, radius, mFromDegrees + index * perDegrees, mChildSize);
final int toXDelta = frame.left - child.getLeft();
final int toYDelta = frame.top - child.getTop();
Interpolator interpolator = mExpanded ? new AccelerateInterpolator() : new OvershootInterpolator(1.5f);
final long startOffset = computeStartOffset(childCount, mExpanded, index, 0.1f, duration, interpolator);
Animation animation = mExpanded ? createShrinkAnimation(0, toXDelta, 0, toYDelta, startOffset, duration,
interpolator) : createExpandAnimation(0, toXDelta, 0, toYDelta, startOffset, duration, interpolator);
final boolean isLast = getTransformedIndex(expanded, childCount, index) == childCount - 1;
animation.setAnimationListener(new AnimationListener() {
@Override
public void onAnimationStart(Animation animation) {
}
@Override
public void onAnimationRepeat(Animation animation) {
}
@Override
public void onAnimationEnd(Animation animation) {
if (isLast) {
postDelayed(new Runnable() {
@Override
public void run() {
onAllAnimationsEnd();
}
}, 0);
}
}
});
child.setAnimation(animation);
}
public boolean isExpanded() {
return mExpanded;
}
public void setArc(float fromDegrees, float toDegrees) {
if (mFromDegrees == fromDegrees && mToDegrees == toDegrees) {
return;
}
mFromDegrees = fromDegrees;
mToDegrees = toDegrees;
requestLayout();
}
public void setChildSize(int size) {
if (mChildSize == size || size < 0) {
return;
}
mChildSize = size;
requestLayout();
}
public int getChildSize() {
return mChildSize;
}
/**
* switch between expansion and shrinkage
*
* @param showAnimation
*/
public void switchState(final boolean showAnimation) {
if (showAnimation) {
final int childCount = getChildCount();
for (int i = 0; i < childCount; i++) {
bindChildAnimation(getChildAt(i), i, 300);
}
}
mExpanded = !mExpanded;
if (!showAnimation) {
requestLayout();
}
invalidate();
}
private void onAllAnimationsEnd() {
final int childCount = getChildCount();
for (int i = 0; i < childCount; i++) {
getChildAt(i).clearAnimation();
}
requestLayout();
}
}
這是第二個名為ArcMenu的Java文件
public class ArcMenu extends RelativeLayout {
private ArcLayout mArcLayout;
private ImageView mHintView;
public ArcMenu(Context context) {
super(context);
init(context);
}
public ArcMenu(Context context, AttributeSet attrs) {
super(context, attrs);
init(context);
applyAttrs(attrs);
}
private void init(Context context) {
LayoutInflater li = (LayoutInflater) context.getSystemService(Context.LAYOUT_INFLATER_SERVICE);
li.inflate(R.layout.arc_menu, this);
mArcLayout = (ArcLayout) findViewById(R.id.item_layout);
final ViewGroup controlLayout = (ViewGroup) findViewById(R.id.control_layout);
controlLayout.setClickable(true);
controlLayout.setOnTouchListener(new OnTouchListener() {
@Override
public boolean onTouch(View v, MotionEvent event) {
if (event.getAction() == MotionEvent.ACTION_DOWN) {
mHintView.startAnimation(createHintSwitchAnimation(mArcLayout.isExpanded()));
mArcLayout.switchState(true);
}
return false;
}
});
mHintView = (ImageView) findViewById(R.id.control_hint);
}
private void applyAttrs(AttributeSet attrs) {
if (attrs != null) {
TypedArray a = getContext().obtainStyledAttributes(attrs, R.styleable.ArcLayout, 0, 0);
float fromDegrees = a.getFloat(R.styleable.ArcLayout_fromDegrees, ArcLayout.DEFAULT_FROM_DEGREES);
float toDegrees = a.getFloat(R.styleable.ArcLayout_toDegrees, ArcLayout.DEFAULT_TO_DEGREES);
mArcLayout.setArc(fromDegrees, toDegrees);
int defaultChildSize = mArcLayout.getChildSize();
int newChildSize = a.getDimensionPixelSize(R.styleable.ArcLayout_childSize, defaultChildSize);
mArcLayout.setChildSize(newChildSize);
a.recycle();
}
}
public void addItem(View item, OnClickListener listener) {
mArcLayout.addView(item);
item.setOnClickListener(getItemClickListener(listener));
}
private OnClickListener getItemClickListener(final OnClickListener listener) {
return new OnClickListener() {
@Override
public void onClick(final View viewClicked) {
Animation animation = bindItemAnimation(viewClicked, true, 400);
animation.setAnimationListener(new AnimationListener() {
@Override
public void onAnimationStart(Animation animation) {
}
@Override
public void onAnimationRepeat(Animation animation) {
}
@Override
public void onAnimationEnd(Animation animation) {
postDelayed(new Runnable() {
@Override
public void run() {
itemDidDisappear();
}
}, 0);
}
});
final int itemCount = mArcLayout.getChildCount();
for (int i = 0; i < itemCount; i++) {
View item = mArcLayout.getChildAt(i);
if (viewClicked != item) {
bindItemAnimation(item, false, 300);
}
}
mArcLayout.invalidate();
mHintView.startAnimation(createHintSwitchAnimation(true));
if (listener != null) {
listener.onClick(viewClicked);
}
}
};
}
private Animation bindItemAnimation(final View child, final boolean isClicked, final long duration) {
Animation animation = createItemDisapperAnimation(duration, isClicked);
child.setAnimation(animation);
return animation;
}
private void itemDidDisappear() {
final int itemCount = mArcLayout.getChildCount();
for (int i = 0; i < itemCount; i++) {
View item = mArcLayout.getChildAt(i);
item.clearAnimation();
}
mArcLayout.switchState(false);
}
private static Animation createItemDisapperAnimation(final long duration, final boolean isClicked) {
AnimationSet animationSet = new AnimationSet(true);
animationSet.addAnimation(new ScaleAnimation(1.0f, isClicked ? 2.0f : 0.0f, 1.0f, isClicked ? 2.0f : 0.0f,
Animation.RELATIVE_TO_SELF, 0.5f, Animation.RELATIVE_TO_SELF, 0.5f));
animationSet.addAnimation(new AlphaAnimation(1.0f, 0.0f));
animationSet.setDuration(duration);
animationSet.setInterpolator(new DecelerateInterpolator());
animationSet.setFillAfter(true);
return animationSet;
}
private static Animation createHintSwitchAnimation(final boolean expanded) {
Animation animation = new RotateAnimation(expanded ? 45 : 0, expanded ? 0 : 45, Animation.RELATIVE_TO_SELF,
0.5f, Animation.RELATIVE_TO_SELF, 0.5f);
animation.setStartOffset(0);
animation.setDuration(100);
animation.setInterpolator(new DecelerateInterpolator());
animation.setFillAfter(true);
return animation;
}
}
我認為這與x和y軸有關,但是我不確定我需要在哪個Java文件中進行更改,或者確切地在何處進行更改
幾個小時后,我想出了要進行更改的地方,它在arc菜單的XML文件中
<com.capricorn.ArcMenu
android:id="@+id/arc_menu_2"
android:layout_width="fill_parent"
android:layout_height="fill_parent"
android:layout_marginLeft="-210dp"
a ndroid:layout_marginBottom="-0dp"
android:layout_alignParentLeft="true"
android:layout_alignParentBottom="true"
arc:fromDegrees="@dimen/menuFromDegrees"
arc:toDegrees="@dimen/menuToDegrees"
arc:childSize="@dimen/menuChildSize"/>
但我把它拉到屏幕的最左邊。無論我怎么做,仍然很難把它拉到屏幕的底部,它一直在屏幕的最中間。
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.