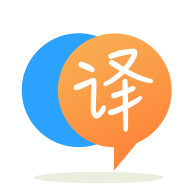
[英]Extract only HTML tags and attributes from a html string using Jsoup
[英]Extract Tags from a html file using Jsoup
我正在對Web文檔進行結構分析。 為此,我只需要提取Web文檔的結構(僅標記)。 我找到了一個名為Jsoup的Java html解析器。 但是我不知道如何使用它來提取標簽。
例:
<html>
<head>
this is head
</head>
<body>
this is body
</body>
</html>
輸出:
html,head,head,body,body,html
聽起來像是深度優先遍歷:
public class JsoupDepthFirst {
private static String htmlTags(Document doc) {
StringBuilder sb = new StringBuilder();
htmlTags(doc.children(), sb);
return sb.toString();
}
private static void htmlTags(Elements elements, StringBuilder sb) {
for(Element el:elements) {
if(sb.length() > 0){
sb.append(",");
}
sb.append(el.nodeName());
htmlTags(el.children(), sb);
sb.append(",").append(el.nodeName());
}
}
public static void main(String... args){
String s = "<html><head>this is head </head><body>this is body</body></html>";
Document doc = Jsoup.parse(s);
System.out.println(htmlTags(doc));
}
}
另一個解決方案是使用jsoup NodeVisitor,如下所示:
SecondSolution ss = new SecondSolution();
doc.traverse(ss);
System.out.println(ss.sb.toString());
類:
public static class SecondSolution implements NodeVisitor {
StringBuilder sb = new StringBuilder();
@Override
public void head(Node node, int depth) {
if (node instanceof Element && !(node instanceof Document)) {
if (sb.length() > 0) {
sb.append(",");
}
sb.append(node.nodeName());
}
}
@Override
public void tail(Node node, int depth) {
if (node instanceof Element && !(node instanceof Document)) {
sb.append(",").append(node.nodeName());
}
}
}
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.