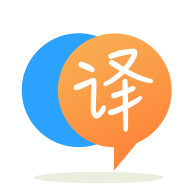
[英]How can I count how many times char occur at first String also cccur at second String?
[英]To count the no. of times a char occur in a string
public static void main(String[] args) {
int a=0,b=0,c=0,d=0,e=0,f=0,g=0,h=0,i1=0,j=0,k=0,l=0,m=0,n=0,o=0,p=0,q=0,r=0,s=0,t=0,u=0,v=0,w=0,x=0,y=0,z = 0;
/初始化變量並計算每個匹配項。
System.out.println("enter the string");
Scanner sc= new Scanner(System.in);
String s1=sc.nextLine();
/ im從用戶那里獲取一個字符串作為ai / p
int a = 0;
for(int i=0;i<(s1.length());i++)
{
if (s1.charAt(i)=='a')
{
a++;
}
if (s1.charAt(i)=='b')
{
b++;
} if (s1.charAt(i)=='c')
{
c++;
} if (s1.charAt(i)=='d')
{
d++;
} if (s1.charAt(i)=='e')
{
e++;
} if (s1.charAt(i)=='f')
{
f++;
} if (s1.charAt(i)=='g')
{
g++;
} if (s1.charAt(i)=='h')
{
h++;
}
if (s1.charAt(i)=='i')
{
i1++;
}
if (s1.charAt(i)=='j')
{
j++;
} if (s1.charAt(i)=='k')
{
k++;
} if (s1.charAt(i)=='l')
{
l++;
} if (s1.charAt(i)=='m')
{
m++;
} if (s1.charAt(i)=='m')
{
m++;
} if (s1.charAt(i)=='n')
{
n++;
} if (s1.charAt(i)=='o')
{
o++;
} if (s1.charAt(i)=='p')
{
p++;
} if (s1.charAt(i)=='q')
{
q++;
} if (s1.charAt(i)=='r')
{
r++;
} if (s1.charAt(i)=='s')
{
s++;
} if (s1.charAt(i)=='t')
{
t++;
} if (s1.charAt(i)=='u')
{
u++;
} if (s1.charAt(i)=='v')
{
v++;
} if (s1.charAt(i)=='w')
{
w++;
}
if (s1.charAt(i)=='x')
{
x++;
} if (s1.charAt(i)=='y')
{
y++;
} if (s1.charAt(i)=='z')
{
z++;
}
/...................................adkjagkdgakjdjakdjg/
}
但是也不能再次寫出全部26個變量以進行打印...請給我一些建議。
最好的方法是使用26
元素的int
數組。 讓我們初始化26個元素的數組:
int[] array=new int[26];
現在可以認為0th
元素是a
, 1st
元素是b
,依此類推。 現在假設我們有字符串str
。 現在將字符串迭代到結尾。
for(int i=0;i<str.length();i++)
{
array[str.charAt(i)-'a']++; //Storing occurrence of characters in array
}
現在,我們在數組中出現了字符,並且只需遍歷array
獲得所有值的打印內容。
for(int i=0;i<26;i++)
{
char ch=(char)('a'+i);
System.out.println(ch+":"+array[i]);
}
或者,如果您只想打印出現的字符:-
for(int i=0;i<26;i++)
{
char ch=(char)('a'+i);
if(array[i]>0)
System.out.println(ch+":"+array[i]);
}
您可以嘗試使用Map
String str = "occurrences";
Map<String, Integer> map = new HashMap<>();
for (char i : str.toCharArray()) {
Integer value = map.get(i + "");
if (value != null) {
map.put(i + "", value + 1);
} else {
map.put(i + "", 1);
}
}
System.out.println(map);
輸出:
{u=1, e=2, s=1, r=2, c=3, n=1, o=1}
如注釋中所述,如果要將唯一鍵與值(在您的情況下為字符及其出現次數)相關聯,則Map<Character,Integer>
是正確的選擇。
有許多網站可以教您如何使用地圖。 這是給初學者的 。 我不會為您提供代碼,因為您顯然正在嘗試學習。
如注釋中所建議,使用Map
。
例如:
System.out.println("enter the string");
Scanner sc= new Scanner(System.in);
String s1=sc.nextLine();
if (s1 != null && !s1.isEmpty()) {
// will display character counts alphabetically
Map<Character, Integer> count = new TreeMap<Character, Integer>();
char[] chars = s1.toCharArray();
for (char c: chars) {
// no count yet for this character
if (count.get(c) == null) {
count.put(c, 1);
}
// this character appeared at least once: incrementing count
else {
count.put(c, count.get(c) + 1);
}
}
System.out.println(count);
}
輸出量
enter the string
abcdeff
{a=1, b=1, c=1, d=1, e=1, f=2}
HashMap<Character, Integer> charCountMap = new HashMap<Character, Integer>;
for(int i=0;i<(s1.length());i++)
{
Integer count = charCountMap(s1.charAt(i));
if(count == null){
charCountMap.put(1);
} else {
charCountMap.put(++count);
}
}
獲取a的出現次數:
int count = charCountMap.get('a');
您可以使用ascii值來實現。
String s;
int[] arr = new int[26];
for(char x : s.toLowerCase().toCharArray()){
arr[x-97]++;
}
for(int i=0; i<arr.length; i++){
System.out.println("Count of "+(char)(97+i)+" :"+arr[i]);
}
int[] array = new int[256];
String str = "sdfdagfvdsgfrewfwqafasfdfa";
for (int i = 0; i < str.length(); i++) {
array[str.charAt(i)]++;
}
for (int i = 'a'; i <= 'z'; i++) {
if (array[i] > 0) {
System.out.println((char) i + ":" + array[i]);
}
}
使用2D數組可以做到如下
公共課櫃台{
private String[][] letterArray;
Counter(){
String letter = "abcdefghijklmnopqrstuvwxyz";
letterArray = new String[26][2];
for(int i = 0; i < letterArray.length; i++){
letterArray[i] = new String[]{letter.charAt(i)+ "", "0"};
}
}
void setLetterCount(String sentence){
for(int i = 0; i < sentence.length(); i++){
String ch = sentence.charAt(i)+"";
for(int x = 0; x < letterArray.length; x++){
String letter = letterArray[x][0].toLowerCase();
int num = Integer.parseInt(letterArray[x][1].toString());
if (letter.equals(ch)){
letterArray[x][1] = ++num+"";
continue;
}
}
}
}
void printLetters(){
for(String arr[] : letterArray){
System.out.println(arr[0]+ " : "+ arr[1]);
}
}
}
公共類CounterApp {
public static void main(String[] args) {
Scanner sc= new Scanner(System.in);
Counter counter = new Counter();
System.out.print("Enter the sentence : ");
String sentence=sc.nextLine();
counter.setLetterCount(sentence);
counter.printLetters();
}
}
沒有2D陣列
公共類LetterCounter {
public static void main(String[] args) {
Scanner sc= new Scanner(System.in);
System.out.print("Enter the sentence : ");
String sentence=sc.nextLine().toLowerCase();
int arr[] = new int[26];
for(int i = 0; i < sentence.length(); i++){
char ch = sentence.charAt(i);
int num = ((int)ch) - 97;
arr[num] = arr[num] + 1;
}
int ch = 97;
for(int i : arr){
if(i > 0) {
System.out.println(((char)ch)+ " : "+ i);
}
ch++;
}
}
}
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.