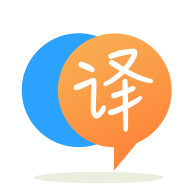
[英]Connect Bluetooth Android Client to Bluetooth Java Server
[英]Android client does not connect to Java server
我正在嘗試在Java和android中實現一個非常簡單的客戶端/服務器應用程序。 當我執行服務器時,服務器似乎運行良好,並且android客戶端在手機上打開。 但是,當我嘗試通過WiFi連接向服務器發送消息時,它不起作用。
這是我的服務器代碼:
package com.SentientShadow;
import java.io.*;
import java.net.ServerSocket;
import java.net.Socket;
public class Server {
public static void main(String[] args) {
Thread thread = new Thread(){
public void run() {
System.out.println("Server has started and is listening...");
try{
ServerSocket socket = new ServerSocket(6879);
while (true){
Socket connection = socket.accept();
DataInputStream input = new DataInputStream(connection.getInputStream());
System.out.println("Received from client: " + input.readUTF());
input.close();
connection.close();
}
}catch(IOException e){
System.out.println("problem accepting connection");
}
}
};
thread.start();
}
}
這是我的客戶活動代碼:
package com.SentientShadow;
import java.io.DataOutputStream;
import java.io.IOException;
import java.net.InetAddress;
import java.net.Socket;
import java.net.UnknownHostException;
import android.support.v7.app.ActionBarActivity;
import android.view.View;
import android.view.View.OnClickListener;
import android.widget.Button;
import android.widget.EditText;
import android.widget.Toast;
import android.os.Bundle;
public class Client extends ActionBarActivity implements OnClickListener {
private EditText etMessage;
private Button bSend;
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_client);
etMessage = (EditText)findViewById(R.id.etMessage);
bSend = (Button)findViewById(R.id.bSend);
bSend.setOnClickListener(this);
}
public void onClick(View view) {
Thread thread = new Thread(){
public void run() {
try {
Socket connection = new Socket("127.0.0.1", 6789);
DataOutputStream output = new DataOutputStream(connection.getOutputStream());
output.writeUTF(etMessage.getText().toString());
output.flush();
output.close();
connection.close();
} catch (UnknownHostException e) {
System.out.println("problem connecting to specified address");
} catch (IOException e) {
System.out.println("problem connecting to port");
}
}
};
thread.start();
Toast.makeText(this, "Message has been sent!", Toast.LENGTH_SHORT).show();
}
}
這是客戶端活動的xml布局文件:
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:paddingBottom="@dimen/activity_vertical_margin"
android:paddingLeft="@dimen/activity_horizontal_margin"
android:paddingRight="@dimen/activity_horizontal_margin"
android:paddingTop="@dimen/activity_vertical_margin"
tools:context="com.SentientShadow.Client" >
<TextView
android:id="@+id/textView1"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Enter message:" />
<EditText
android:id="@+id/etMessage"
android:layout_width="wrap_content"
android:layout_height="100dp"
android:layout_alignLeft="@+id/textView1"
android:layout_below="@+id/textView1"
android:layout_marginTop="14dp"
android:ems="10" />
<Button
android:id="@+id/bSend"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_alignLeft="@+id/editText1"
android:layout_below="@+id/editText1"
android:text="Send" />
</RelativeLayout>
這是我的xml清單文件的代碼:
<?xml version="1.0" encoding="utf-8"?>
<manifest xmlns:android="http://schemas.android.com/apk/res/android"
package="com.SentientShadow"
android:versionCode="1"
android:versionName="1.0" >
<uses-sdk
android:minSdkVersion="8"
android:targetSdkVersion="19" />
<uses-permission android:name="android.permission.INTERNET"/>
<uses-permission android:name="android.permission.ACCESS_NETWORK_STATE"/>
<application
android:allowBackup="true"
android:icon="@drawable/ic_launcher"
android:label="@string/app_name"
android:theme="@style/AppTheme" >
<activity
android:name="Client"
android:label="@string/app_name" >
<intent-filter>
<action android:name="android.intent.action.MAIN"/>
<category android:name="android.intent.category.LAUNCHER"/>
</intent-filter>
</activity>
</application>
</manifest>
我用System.out.println消息替換了IOException和UnknownHostException printstacktrace,以便我可以確定問題是否來自這些錯誤中的任何一個。 但是,沒有任何內容打印到控制台。
我還使用端口掃描程序掃描了多個端口,由於它們以關閉狀態返回,因此我的學校網絡似乎已阻止了所有這些端口。 但是,我之前已經制作了另一個客戶端/服務器應用程序,其中客戶端和服務器都完全用java作為桌面應用程序進行編碼。 在這種情況下,我使用本地主機(127.0.0.1)作為IP地址,並嘗試了具有多個端口的應用程序,並且該應用程序正常工作。 我還使用連接的公共IP地址嘗試了該應用程序,並且該應用程序正常運行。
因此,我開始認為這不是連接端口的問題,而是應用程序的android客戶端的問題。 但我不知道出什么問題了。
順便說一句,當我嘗試發送消息時,運行客戶端的手機和運行服務器的筆記本電腦都連接到同一網絡。
好的,我終於找到了問題,這要歸功於greenapps的建議。 基本上,事實證明防火牆阻止了我計算機上與Java平台的任何傳入連接。
要解決此問題:
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.