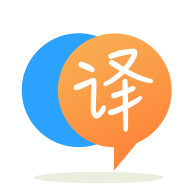
[英]Using PrintWriter and DataOutputStream to write in same file in java
[英]Write int to file using DataOutputStream
我正在生成隨機整數,並嘗試將其寫入文件。 問題是,當我打開創建的文件時,找不到整數,而是找到一組符號,例如正方形等。這是編碼問題嗎?
import java.io.DataOutputStream;
import java.io.File;
import java.io.FileNotFoundException;
import java.io.FileOutputStream;
import java.io.IOException;
import java.util.ArrayList;
import java.util.List;
public class GenerateBigList {
public static void main(String[] args) {
//generate in memory big list of numbers in [0, 100]
List list = new ArrayList<Integer>(1000);
for (int i = 0; i < 1000; i++) {
Double randDouble = Math.random() * 100;
int randInt = randDouble.intValue();
list.add(randInt);
}
//write it down to disk
File file = new File("tmpFileSort.txt");
try {
FileOutputStream fos = new FileOutputStream("C:/tmp/tmpFileSort.txt");
DataOutputStream dos = new DataOutputStream(fos);
writeListInteger(list, dos);
dos.close();
} catch (FileNotFoundException e) {
e.printStackTrace();
} catch (IOException e) {
e.printStackTrace();
}
}
private static void writeListInteger(List<Integer> list, DataOutputStream dos) throws IOException {
for (Integer elt : list) {
dos.writeInt(elt);
}
}
}
從創建的文件中粘貼部分副本:
/ O a C ? 6 N
從文檔 :
public final void writeInt(int v) throws IOException
Writes an int to the underlying output stream as four bytes, high byte first. If no exception is thrown, the counter written is incremented by 4.
沒有編碼問題。 這就是使用文本編輯器打開二進制文件時看到的。 嘗試使用十六進制編輯器打開。
這些“符號”就是您的觀點。 如果在文本編輯器中打開二進制文件,則二進制文件看起來就是這樣。 請注意,該文件的大小恰好為4000字節,並且您寫入了1000個int,每個4個字節。
如果使用DataInputStream讀取文件,則將獲得原始值:
try (DataInputStream dis = new DataInputStream(
new BufferedInputStream(new FileInputStream("C:/tmp/tmpFileSort.txt")))) {
for (int i = 0; i < 1000; i++) {
System.out.println(dis.readInt());
}
} catch (IOException e) {
throw new RuntimeException(e);
}
它寫二進制而不是文本。 您的期望放錯了地方。 如果需要文本,請使用Writer。
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.