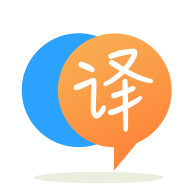
[英]Find the second smallest integer in an array. Returns wrong value for 2nd smallest integer
[英]trying to find second smallest integer in array recursively java
我很迷路。 我需要遞歸地找到數組中的第二個最小整數。 我已經開始編寫該方法,但是我知道它是錯誤的,並且不知道從這里去哪里。
public static int findSecondSmallest(int [] array)
{
int secSmall = array[0], min = array[0];
if(array.length >= 1)
{
findSecondSmallest(array);
if(secSmall > min)
secSmall = array[0+1];
}
return secSmall;
}
您可以做的是在從頭到尾遍歷數組時跟蹤最小的一個和第二個最小的一個。 如果遇到小於第二個最小的東西或大於第二個最小但小於第二個最小的東西,請更新它們。 希望以下代碼有意義:
public class Driver {
public static int MAX_VAL = 1000000;
public static void main(String[] args) {
int[] arr = {2,5,3,6,2,7,43,2,56,2,-1, 1, 5};
int[] smalls = new int[2];
int sm = find(arr, 0, smalls);
System.out.println(sm);
}
public static int find(int[] arr, int index, int [] smalls) {
if(index == 0) {
smalls[0] = arr[index];
smalls[1] = Integer.MAX_VALUE;
find(arr, index+1, smalls);
} else if(index < arr.length){
if(arr[index] < smalls[0]){
smalls[1] = smalls[0];
smalls[0] = arr[index];
} else if(smalls[1] > arr[index]) {
smalls[1] = arr[index];
}
find(arr,index + 1, smalls);
}
return smalls[1];
}
}
在這里,index代表“部分數組”中最后一個元素的索引。 每個遞歸步驟都檢查數組的第一個索引+ 1個元素。 注意:small [0]是部分數組的最小元素,small [1]是部分數組的第二個最小元素。
為了更好地處理遞歸,我建議您選擇Prolog。 這種語言沒有循環,您將嚴重依賴於遞歸。
迭代非常容易做到,因此遞歸解決方案已經有些設計了,有幾種方法可以做到。 例如,您可以編寫一個函數,該函數在數組的兩半上遞歸,並給出返回的四個數字中的第二個最小值。 我假設您想分割第一個元素,然后遞歸到數組的其余部分。
遞歸函數將返回IntPair
最小的和第二個最小的IntPair
,我忽略了它的定義,因此您將需要一個包裝器函數從該對中提取第二個最小的值。
public static int findSecondSmallest(int[] array) {
return findSecondSmallestAndSmallest(0, array).getSecond();
}
private static IntPair recurseSecondSmallest(int index, int[] array) {
if (array.length - index == 2) {
if (array[index] < array[index+1])
return new IntPair(array[index], array[index+1]);
else return new IntPair(array[index+1], array[index]);
}
IntPair recursiveResult = recurseSecondSmallest(index+1, array);
if (array[index] < recursiveResult.getFirst())
return new IntPair(array[index], recursiveResult.getFirst());
else if (array[index] < recursiveResult.getSecond())
return new IntPair(recursiveResult.getSecond(), array[index]);
else return recursiveResult;
}
訣竅是更聰明的偽代碼。 這可能不是最有效的算法,但它是愚蠢的智能算法。 同樣在任何情況下,遞歸都不是解決此類問題的方法。
第二最小(大小為n的數組)
if n == 2
if array[0] < array[1]
return array[1]
else return array[2]
if n not equal 2 then
remove largest element from the array
return secondsmallest(newArray of size n-1)
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.